Convert camelCaseText to Title Case Text
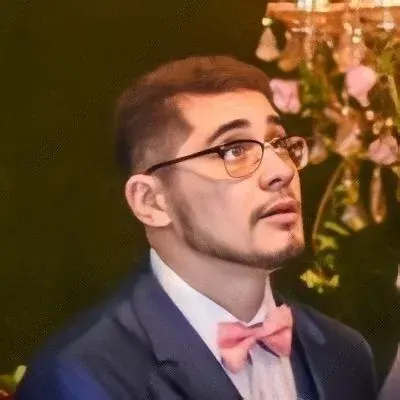
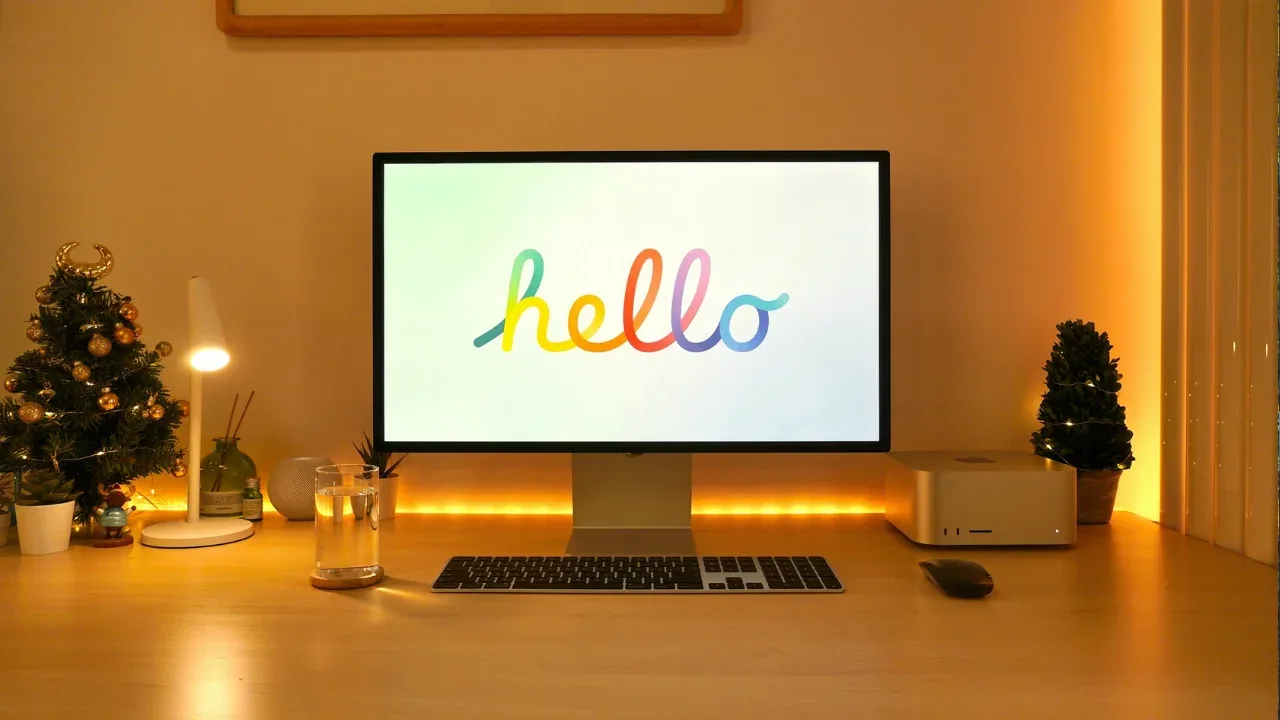
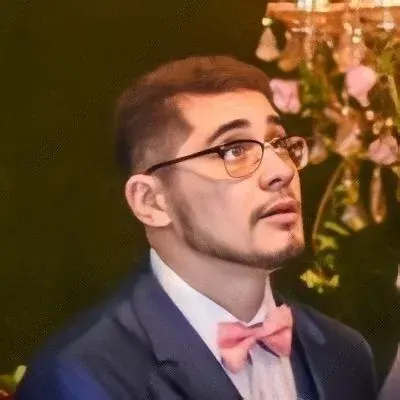
🐫🔀💬 Converting camelCaseText to Title Case Text in JavaScript
Have you ever encountered an awkward situation where you need to convert a string written in camel case format to a more readable and conventional title case format? 🆘 Don't worry, we've got you covered! In this guide, we'll explore common issues associated with this problem and provide you with simple solutions using JavaScript.
The Challenge
Let's consider the following scenario: someone asks you to convert a string like 'helloThere'
or 'HelloThere'
to 'Hello There'
. If you're scratching your head and wondering how to tackle this, you're in the right place! The challenge is to convert these camel case strings into title case strings, where each word begins with a capital letter, creating visually appealing and easy-to-read text. 😎
Common Issues
Before diving into the solutions, let's demystify some common issues you might encounter when approaching this problem:
Retaining Existing Capitalization: One issue to consider is whether the first letter of the original string is already capitalized or not. Depending on the input, we may need to preserve that capitalization or capitalize it if needed.
Handle Abbreviations and Acronyms: Another challenge arises when dealing with abbreviations or acronyms within the string. In title case, these should typically remain in all caps, like "XML" or "API".
Simple Solutions
Now, let's explore some straightforward solutions using JavaScript to convert camelCaseText into Title Case Text:
function convertCamelCaseToTitleCase(input) {
// Place a space before any capital letters that are preceded by lowercase letters
const adjustedString = input.replace(/([a-z])([A-Z])/g, '$1 $2');
// Capitalize the first letter of the adjusted string
const titleCasedString = adjustedString.charAt(0).toUpperCase() + adjustedString.slice(1);
return titleCasedString;
}
// Usage example
const camelCaseString = 'helloThere';
const titleCaseString = convertCamelCaseToTitleCase(camelCaseString);
console.log(titleCaseString); // Output: "Hello There"
In this solution, we use a regular expression (/([a-z])([A-Z])/g
) to identify any capital letters that are preceded by lowercase letters. We then insert a space between them. Next, we capitalize the first letter of the adjusted string using .toUpperCase()
and slice()
. Finally, we return the title cased string.
Level up Your Function
Now that you have a basic solution, you can take it to the next level by improving the function to account for additional edge cases and enhancing its flexibility. Here are a few ideas to get you started:
Handling Acronyms: When encountering acronyms like "API" or "XML", we can modify the function to exclude them from the regular expression logic.
Supporting Different Separator Characters: Apart from camelCase, some strings may contain underscores or hyphens as separators. You can include additional logic to handle these scenarios as well.
Creating a Helper Function: Instead of writing the same conversion logic multiple times, you can abstract the steps into a reusable helper function to convert any string to title case automatically.
What's Next?
Congratulations! 🎉 You have successfully learned how to convert camelCaseText to Title Case Text using JavaScript. Now it's time to put your newfound knowledge into practice! Modify the provided function or create your own improved version to handle different scenarios.
Feel free to experiment with the function and share any cool variations or additional features you come up with. If you have any questions or need further assistance, don't hesitate to leave a comment below. Happy coding! 💻✨
Inspired by a question on Stack Overflow.