Convert array to JSON
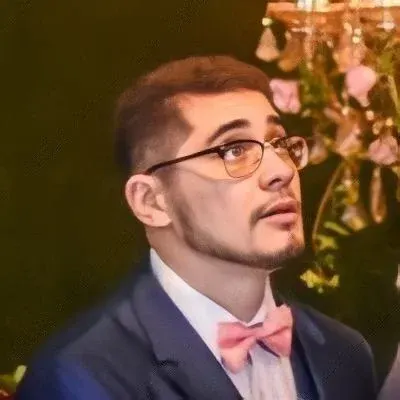
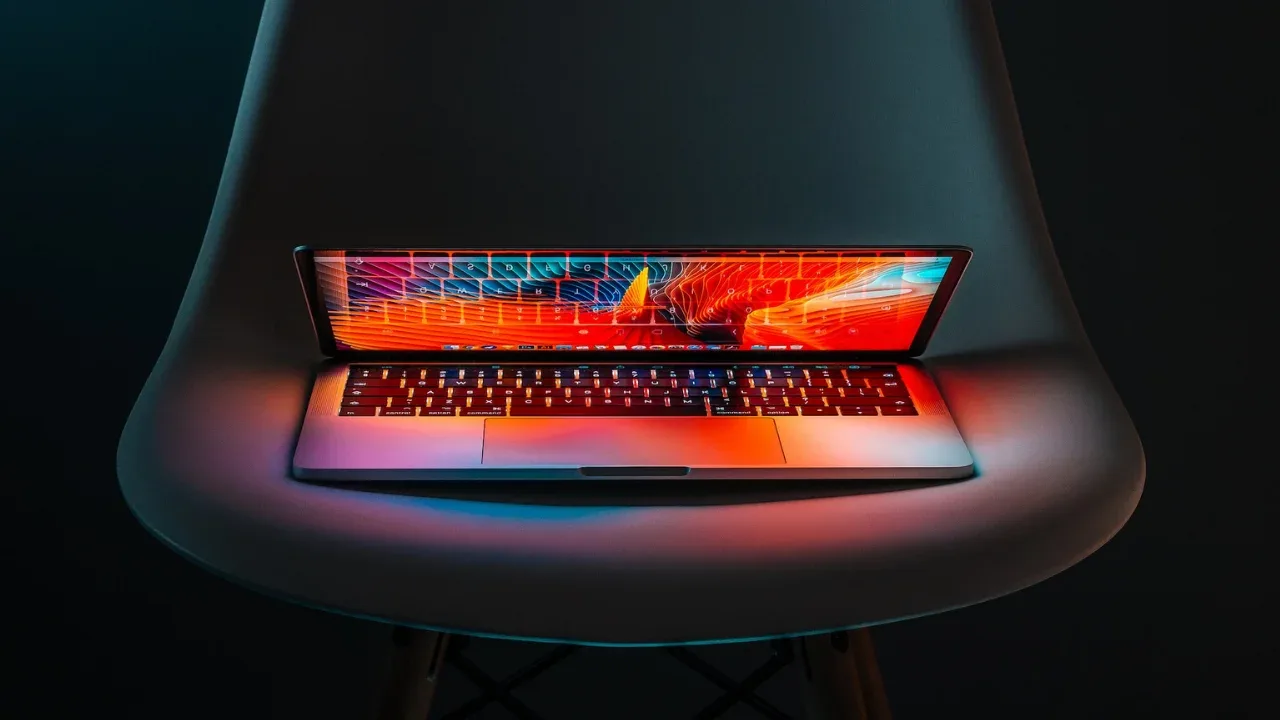
Converting an Array to JSON Made Easy 🚀
So, you've got an array of integers and you need to send it to a page using jQuery's .get
method. The catch is that you need to convert the array into a JSON object first. Don't worry, I've got your back! In this blog post, I'll show you how to tackle this problem with ease. Let's dive in! 💪
Understanding the Challenge 🤔
Before we jump into the solution, let's take a moment to understand the challenge at hand. In JavaScript, an array is a collection of elements, while a JSON object is a key-value pair representation of data. So, in order to send our array as JSON, we'll need to convert it into an object where the array values become the values of the object.
The Solution 🎉
To convert our array to JSON, we can simply use the JSON.stringify()
method. This method takes an object or value as an argument and returns the corresponding JSON string representation.
Here's how you can use JSON.stringify()
to convert your array to JSON:
var cars = [2, 3, ...]; // your array
var jsonCars = JSON.stringify(cars);
Now, jsonCars
will be a JSON string representation of your array. 😃
Feeling Good So Far? Let's Go the Extra Mile 💪
Converting your array to JSON is great, but what if you need to send that JSON data as part of an AJAX request? No worries, I've got you covered! Here's a snippet that demonstrates how you can use the converted JSON data in an AJAX request with jQuery:
var cars = [2, 3, ...]; // your array
var jsonCars = JSON.stringify(cars);
$.ajax({
url: "some-page.php",
method: "GET",
data: { cars: jsonCars },
success: function(response) {
// Handle success
},
error: function(error) {
// Handle error
}
});
In the example above, we set the data
property of the AJAX request to an object with the key cars
, which holds our converted JSON string. This way, the server can easily parse the JSON data. 🌟
Wrap Up and Take Action ✨
Now that you know how to convert an array to JSON using JSON.stringify()
and send it in an AJAX request with jQuery, you have a powerful trick up your sleeve! So why not give it a try and level up your JavaScript skills? 😉
Feel free to experiment with the code snippets provided and adapt them to your specific needs. And if you have any questions or cool ideas to share, I'd love to hear from you in the comments below!
Happy coding! 💻✌️
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
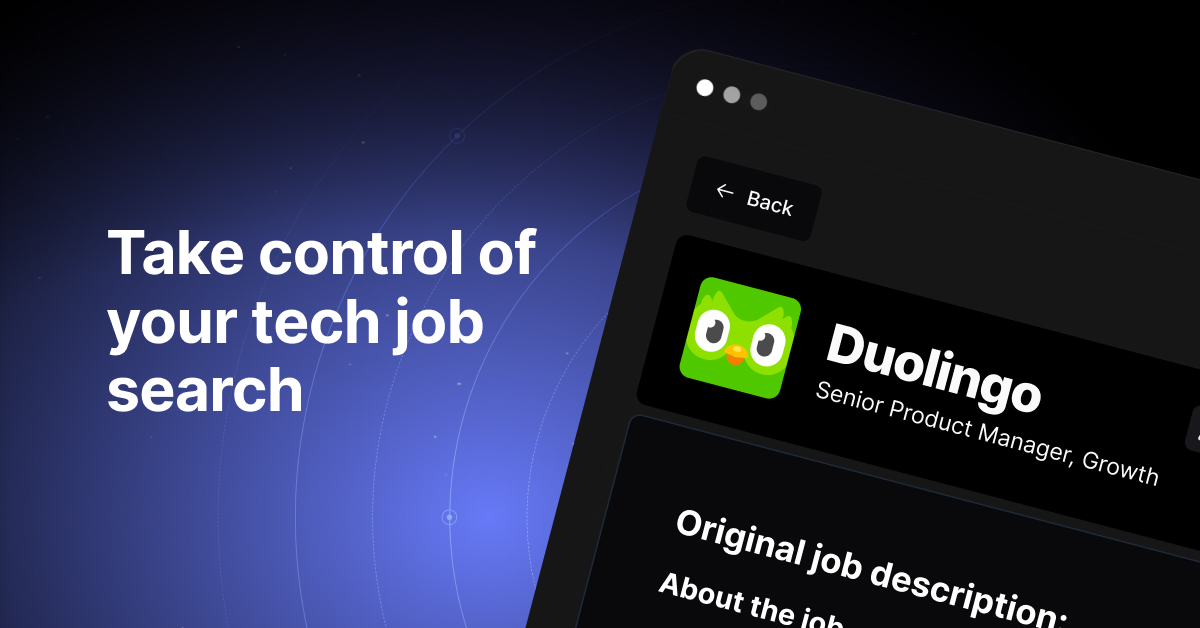