Convert a Unix timestamp to time in JavaScript
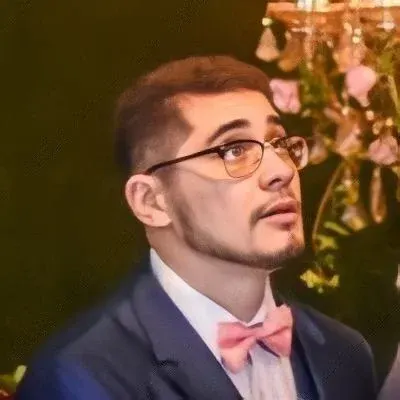
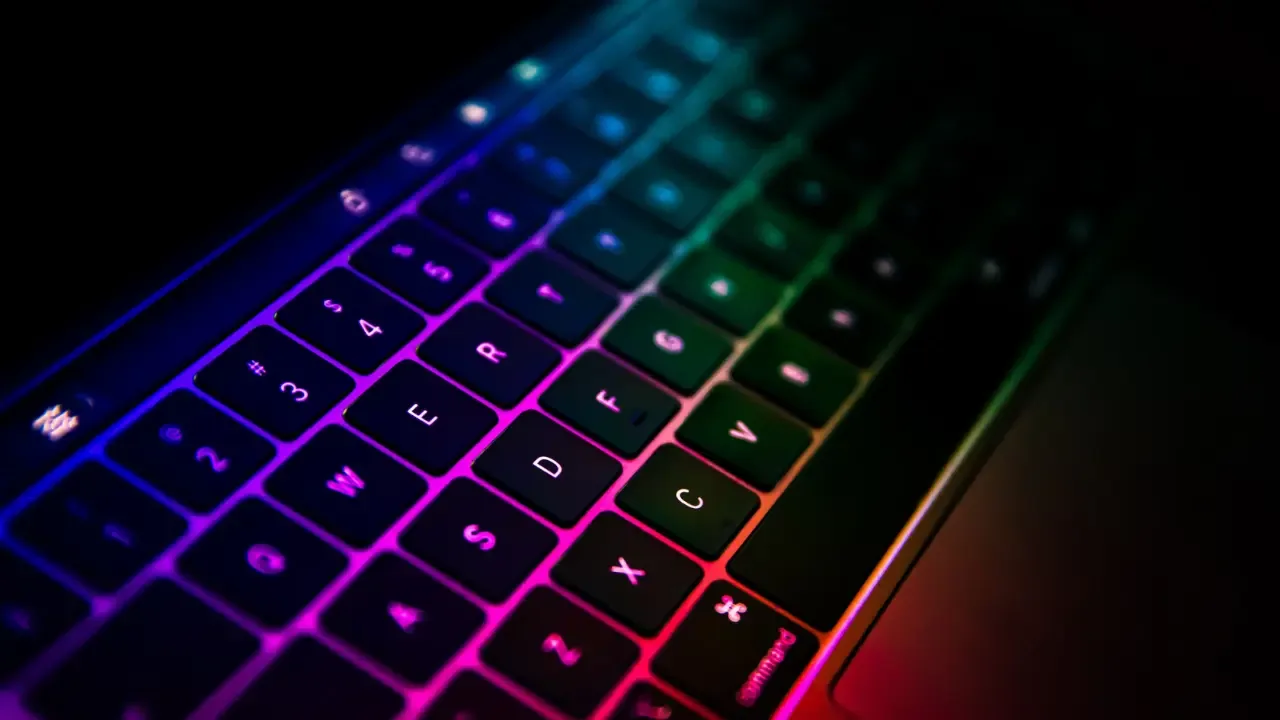
Converting Unix Timestamp to Time in JavaScript 🕒
So, you've got a Unix timestamp and you want to convert it to the actual time in JavaScript? You've come to the right place! In this guide, we'll explore some common issues, provide easy solutions, and help you get the time you desire in a snappy ⏰ manner.
Understanding the Unix Timestamp 📅
Before we dive into the conversion process, let's quickly understand what a Unix timestamp is. In simple terms, a Unix timestamp represents the number of seconds that have elapsed since the Unix epoch - January 1, 1970, at 00:00:00 UTC.
The Problem at Hand 🤔
You have a Unix timestamp stored in your MySQL database, and you need to extract just the time from it in the HH:MM:SS
format. Well, fret not! We've got you covered.
The Solution 💡
Step 1: Getting the Unix Timestamp
First things first, you'll need to retrieve the Unix timestamp from your MySQL database using your preferred method. Once you have the timestamp, you can proceed to convert it to a readable time format.
Step 2: Converting the Unix Timestamp
To convert the Unix timestamp to a readable time format, JavaScript provides the Date
object. Simply create a new Date
object, passing the Unix timestamp as an argument.
const unixTimestamp = 1590571220; // Replace with your actual Unix timestamp
const date = new Date(unixTimestamp * 1000);
Note: The Unix timestamp is in seconds, whereas the JavaScript Date
object works with milliseconds. Hence, you need to multiply the Unix timestamp by 1000.
Step 3: Getting the Time Components
Now that you have the Date
object ready, you can access its various methods to obtain the individual time components. Here's how you can get the hours, minutes, and seconds:
const hours = date.getHours();
const minutes = date.getMinutes();
const seconds = date.getSeconds();
Step 4: Formatting the Time Components
To display the time in the desired HH:MM:SS
format, we need to ensure that each component is two digits long. If a component is only one digit, we'll prefix it with a leading zero.
function formatTime(time) {
return time < 10 ? `0${time}` : time;
}
const formattedHours = formatTime(hours);
const formattedMinutes = formatTime(minutes);
const formattedSeconds = formatTime(seconds);
Step 5: Combining the Time Components
Lastly, we can concatenate the formatted time components using colons to form the final time string.
const timeString = `${formattedHours}:${formattedMinutes}:${formattedSeconds}`;
Congratulations! You now have the time extracted from the Unix timestamp in your desired format. 🎉
Time to Engage! 🚀
Now that you know how to convert a Unix timestamp to time in JavaScript, why not put your newfound skills to the test? Try it out using your own Unix timestamps or experiment further with the code provided.
We would love to hear your experiences and any additional tips or tricks you've uncovered along the way. Share your thoughts, questions, or suggestions in the comments below and let's continue the conversation! 👇
Keep exploring, keep coding! 😄
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
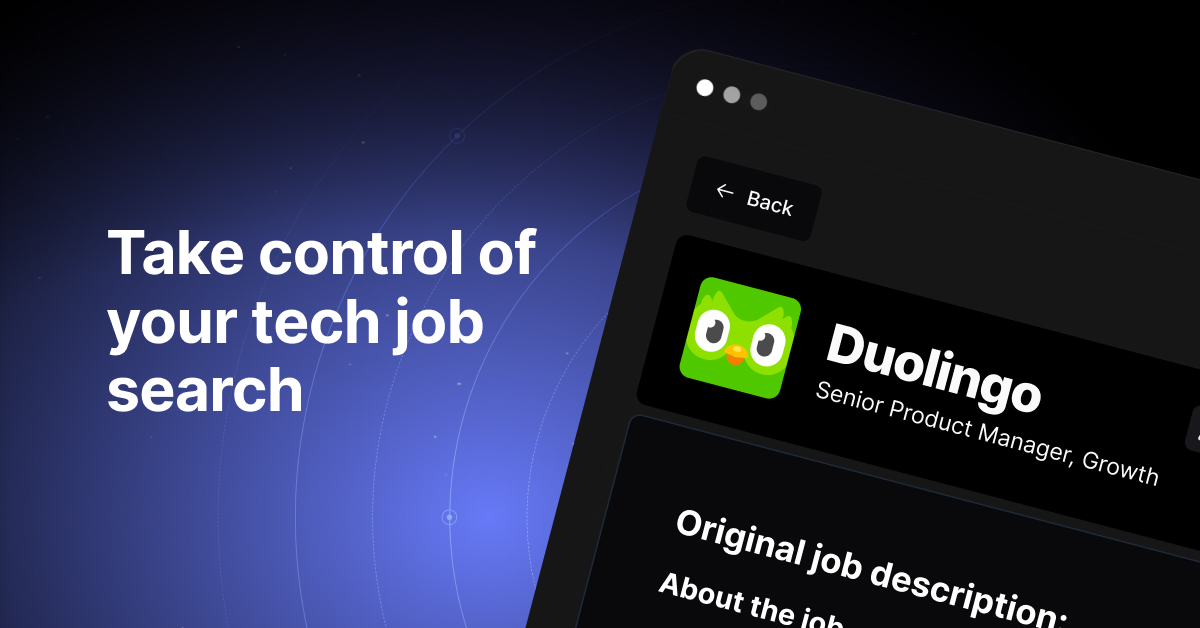