Concrete JavaScript regular expression for accented characters (diacritics)
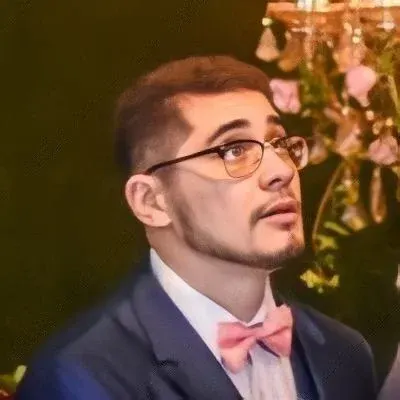
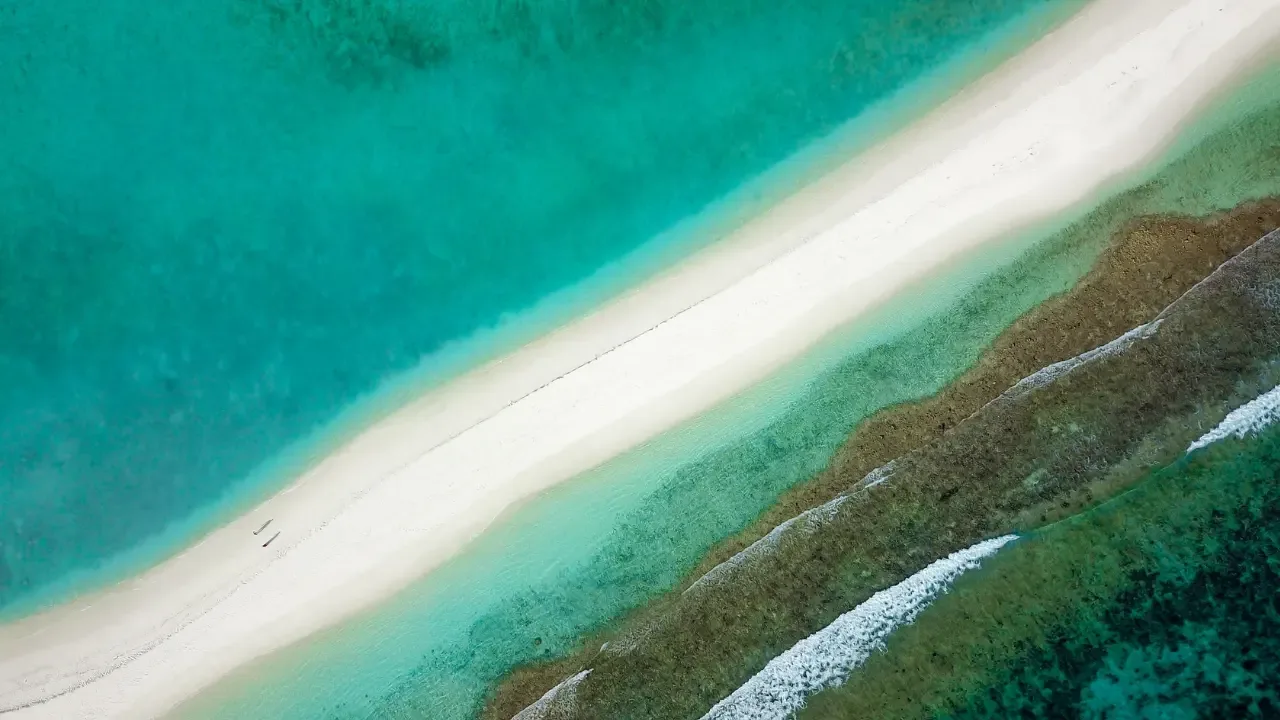
How to Match Accented Characters (Diacritics) in JavaScript: A Comprehensive Guide
Are you struggling with matching accented characters (those with diacritical marks) in JavaScript? 🤔 Worry no more! In this guide, we'll discuss common issues and provide three easy-to-implement solutions for this problem. Let's dive in! 💪
The Problem
You want to enforce a UI field format that requires the last name and first name to be separated by a comma and a space. It seems straightforward, but when it comes to supporting diacritics, JavaScript presents some challenges.
Existing Solutions
You've explored various sources, such as Stack Overflow, but haven't found a concrete answer to your question. Let's take a look at three possible solutions you've considered:
1. The Accented Characters List Approach 📃
This approach involves explicitly listing all accented characters that you want to accept as valid. Although it works, it can be cumbersome and prone to errors.
Here's an example implementation:
var accentedCharacters = "àèìòùÀÈÌÒÙáéíóúýÁÉÍÓÚÝâêîôûÂÊÎÔÛãñõÃÑÕäëïöüÿÄËÏÖÜŸçÇߨøÅ寿œ";
var regex = "^[a-zA-Z" + accentedCharacters + "]+,\\s[a-zA-Z" + accentedCharacters + "]+$";
var regexCompiled = new RegExp(regex);
This solution matches a last/first name with any supported accented characters from the accentedCharacters
list.
2. The Any Character Wildcard Approach 🃏
Another approach is using the .
character class, which matches any character except the newline character. It simplifies the expression but may be too lenient in its matching criteria.
Here's an example implementation:
var regex = /^.+,\s.+$/;
This solution matches for almost anything in the form of something, something
. While concise, it may not provide the precise control you desire.
3. The Unicode Range Approach 💫
The last approach utilizes Unicode character ranges to match accented characters. It provides better precision and control over the matching process.
Here's an example implementation:
var regex = /^[a-zA-Z\u00C0-\u017F]+,\s[a-zA-Z\u00C0-\u017F]+$/;
This solution matches a range of Unicode characters commonly used in names. It is more accurate and suitable for your expected input.
Considerations and Recommendations
When choosing a solution, it's essential to consider a few factors:
Flexibility: The first approach is limiting and cumbersome to maintain. Avoid it if possible.
Precision: The second approach is concise but may match more than necessary. Exercise caution when using it.
Accuracy: The third approach seems to be the most precise. It restricts matches to the desired range of Unicode characters.
Remember that faculty members won't be submitting forms with names in non-Latin character sets (e.g., Arabic, Chinese, Japanese). This simplifies the matching requirements, allowing you to focus on matching Latin characters.
Your Call to Action 📢
Now that you have three viable solutions for matching accented characters, it's time to put them into practice! Experiment with each solution and assess which one best fits your needs.
Feel free to leave a comment below, sharing your experiences or asking any further questions. We'd love to hear from you! Happy coding! 😄✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
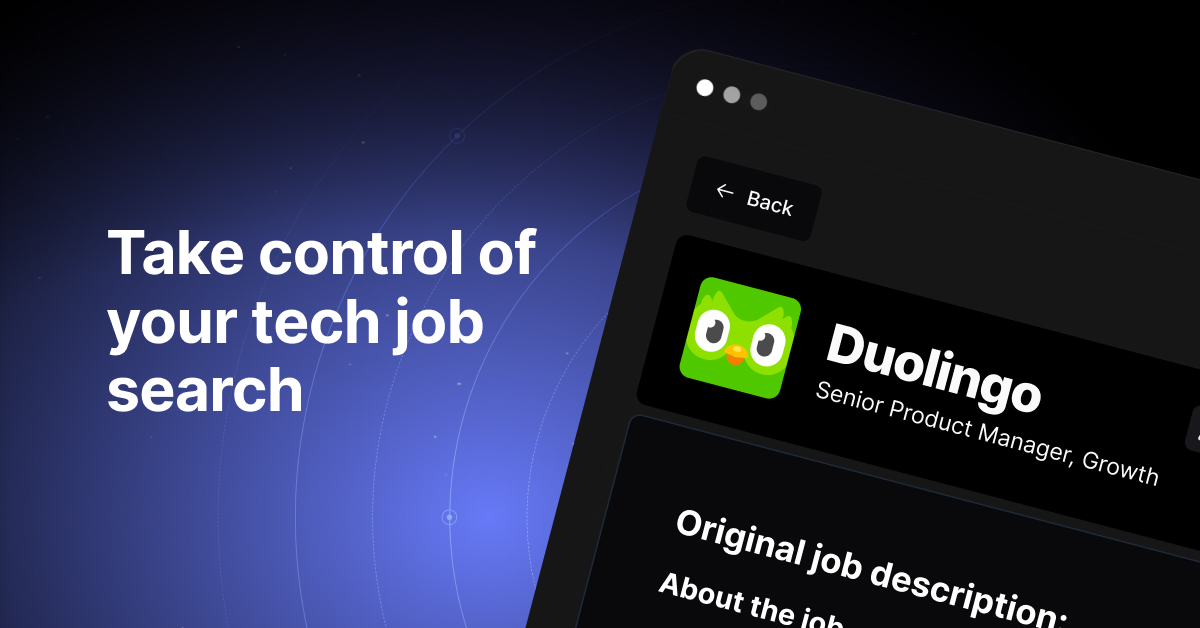