Concatenating variables and strings in React
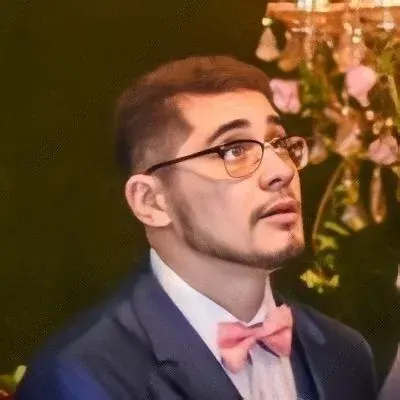
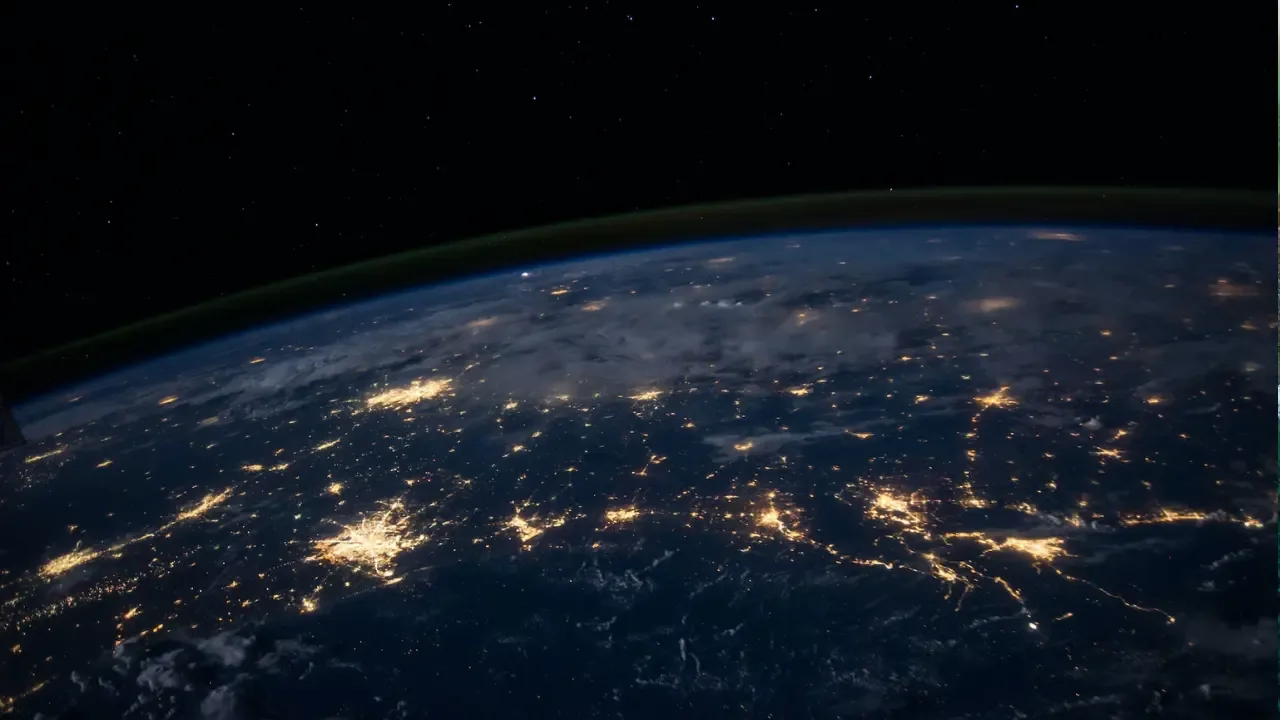
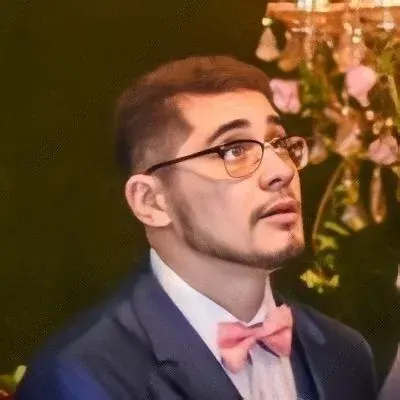
How to Concatenate Variables and Strings in React
Hello fellow React enthusiasts! 👋 Are you struggling to incorporate React's curly brace notation and an href
tag? Do you want to dynamically generate values for HTML attributes in your React app? Look no further, because we have the solution for you! In this article, we will explore how to concatenate variables and strings in React with ease. Let's jump right in! 💪
The Problem
The question at hand is how to add the id
state to the href
HTML attribute using React's curly brace notation. Here is the code snippet we're working with:
{this.state.id}
And the desired HTML attribute on a tag:
href="#demo1"
id="demo1"
The goal is to dynamically add the id
state value to the href
attribute, resulting in the following output:
href="#demo1"
The Solution
To achieve this, we need to concatenate the string "#demo" with the value of this.state.id
. React provides various ways to accomplish this, but we'll focus on two common approaches: using template literals or using the +
operator. Let's dive into each method:
1. Using Template Literals
Template literals, denoted by the backtick (`), allow us to embed expressions inside strings. Here's how we can use template literals to concatenate the href
value:
href={`#demo${this.state.id}`}
The ${}
syntax enables us to inject the value of this.state.id
into the string. React will evaluate the expression within the curly braces and replace it with the corresponding value, resulting in the desired output.
2. Using the +
Operator
If you prefer a more traditional approach, you can also use the +
operator to concatenate the string and variable:
href={"#demo" + this.state.id}
Here, we simply concatenate the string "#demo" with this.state.id
. React automatically treats the expression as a string and merges them together. This method achieves the same result as using template literals, but with a slightly different syntax.
Putting It All Together
Now that you know the two methods for concatenating variables and strings in React, it's time to apply this knowledge to your own projects. Experiment with both approaches and choose the one that resonates with you and your team.
Remember, the key is to wrap your concatenated value within curly braces when using JSX attributes. This signals to React that the value is an expression to be evaluated.
Conclusion
Congratulations! 🎉 You have learned how to concatenate variables and strings in React effortlessly. Whether you prefer using template literals or the +
operator, you now have the power to dynamically generate HTML attribute values in your React components. Happy coding! 💻
If you found this article helpful, don't hesitate to share it with your fellow React developers. Leave a comment below to let us know your preferred method, or if you have any other tips related to concatenating variables and strings in React. Keep exploring, learning, and building amazing things with React! 👍