componentDidMount equivalent on a React function/Hooks component?
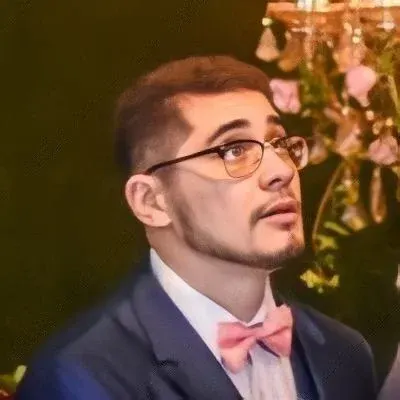
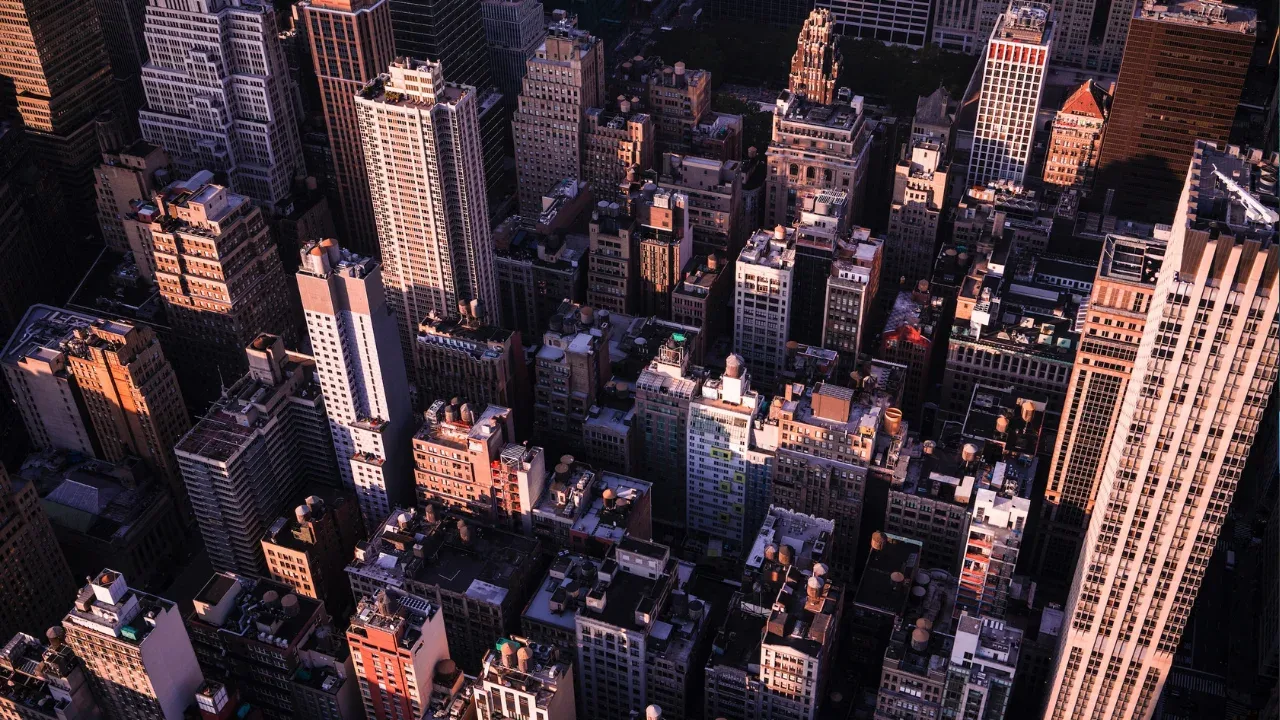
🚀 Simulating componentDidMount in React Function/Hooks Components
Are you a React developer trying to figure out how to achieve the same functionality as componentDidMount
in functional components using React Hooks? 🎣 Don't worry, we've got you covered! In this blog post, we'll dive into the world of React Hooks and explore how you can simulate the behavior of componentDidMount
in your functional components. 🎉
💡 Understanding the Problem
Before we offer you solutions, let's make sure we understand the problem at hand. In class-based components, the componentDidMount
lifecycle method is used to perform actions after the component has been rendered and mounted. However, in functional components, there is no direct equivalent.
🧱 Solution 1: useEffect Hook
The useEffect
Hook is a versatile tool that can help us achieve the desired effect. By leveraging the useEffect
Hook, we can simulate the behavior of componentDidMount
.
Here's an example of how you can use the useEffect
Hook to simulate componentDidMount
:
import React, { useEffect } from 'react';
function MyComponent() {
useEffect(() => {
// Code to run after component mount
// You can perform side effects or fetch data here
console.log("Component mounted!");
}, []);
return (
// Your component UI
<div>
{/* ... */}
</div>
);
}
In the example above, we're passing an empty array ([]
) as the second argument to useEffect
. By doing this, the useEffect
Hook will only run once, simulating the behavior of componentDidMount
.
🧩 Solution 2: useRef Hook
The useRef
Hook can also be used to simulate componentDidMount
. Although it is primarily used to get access to a mutable value across renders, it can serve our purpose here too.
Here's an example of how you can use the useRef
Hook to simulate componentDidMount
:
import React, { useRef, useEffect } from 'react';
function MyComponent() {
const isFirstRender = useRef(true);
useEffect(() => {
if (isFirstRender.current) {
// Code to run after component mount
console.log("Component mounted!");
isFirstRender.current = false;
}
});
return (
// Your component UI
<div>
{/* ... */}
</div>
);
}
In the example above, we're using a useRef
variable called isFirstRender
to keep track of the first render. When the component mounts, we check the value of isFirstRender.current
and perform the desired actions accordingly.
🔧 Looking for More?
If you're interested in diving deeper into React Hooks, make sure to check out the official React Hooks documentation. There, you'll find more information about different Hooks and how they can be used to solve common problems.
🙌 Your Turn
Now it's your turn to experiment with these solutions! Give them a try in your functional components and see how they work for you. If you encounter any issues or have questions, feel free to leave a comment below. Let's keep the conversation going! 💬
Happy coding! 👩💻👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
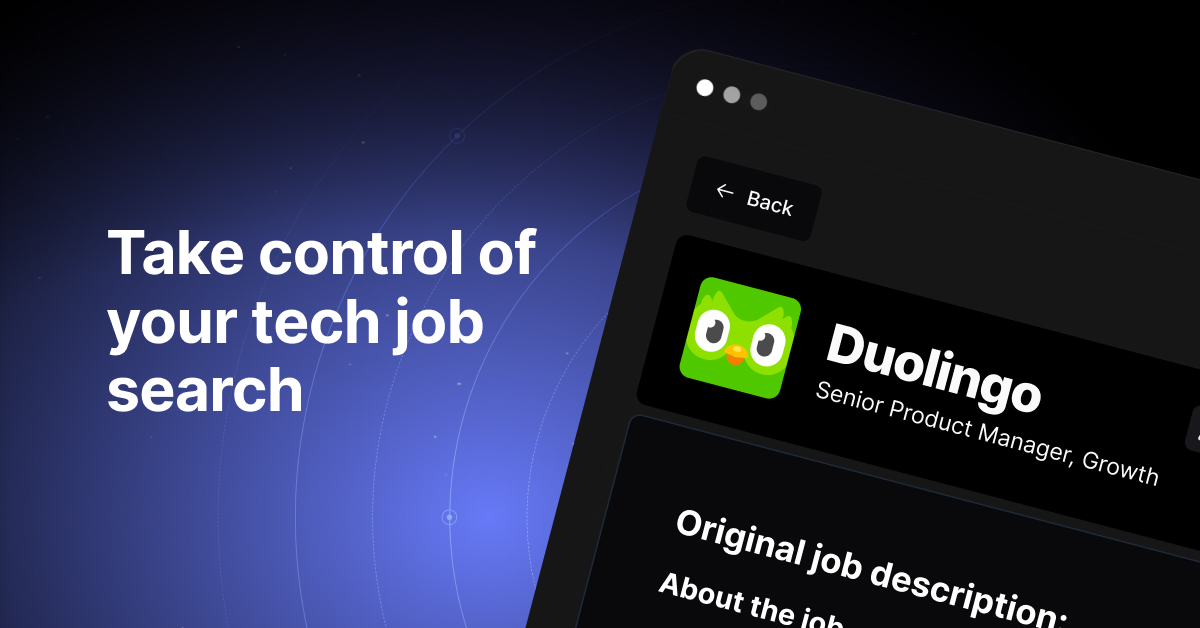