Compare two dates with JavaScript
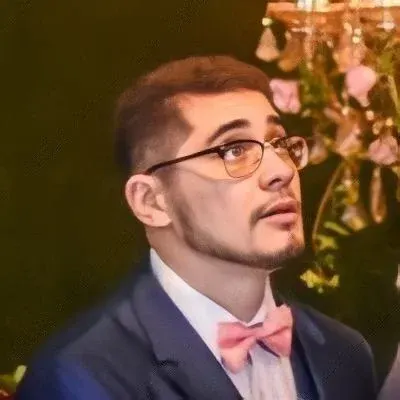
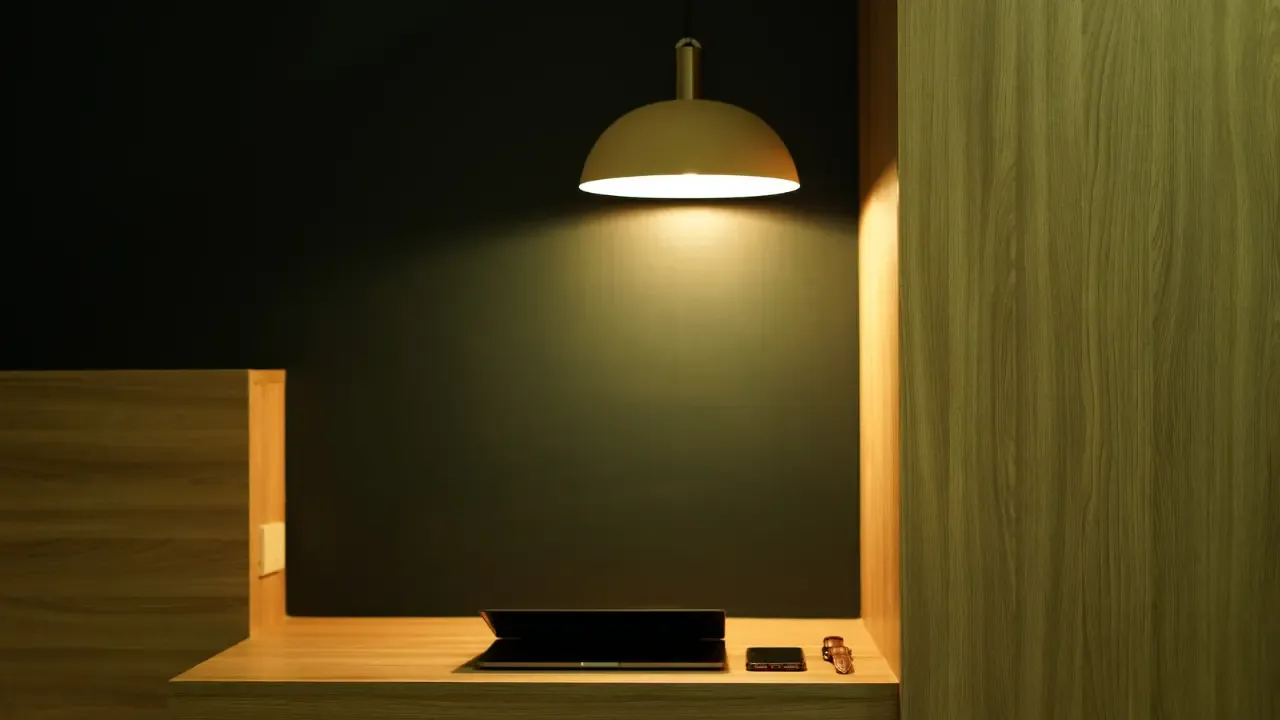
🔍 Comparing Dates with JavaScript: An Easy Guide! 👩💻📅
So, you're stuck in a web development project and need to compare two dates with JavaScript? 🤔 Don't worry, my friend! I've got you covered! In this article, we'll explore common issues, provide straightforward solutions, and help you become a JavaScript date comparison ninja! 💪
⚡ The Problem: Comparing Dates ⚡
The challenge here is to determine whether one date is greater than, less than, or not in the past compared to another date. This is particularly useful when dealing with user input from text boxes or other form elements. Let's dive into some solutions, shall we? 🏊♂️
🧠 Solution 1: Converting Strings to Date Objects 📝
To properly compare dates in JavaScript, we'll need to convert the input strings into Date
objects. The Date
object provides a variety of methods and properties to work with. Here's how we can tackle this:
const date1 = new Date('2022-01-01');
const date2 = new Date('2022-02-01');
Great! Now we have two Date
objects representing date1
and date2
. But how do we compare them? 🧐
🔀 Solution 2: Using Comparison Operators 🎯
JavaScript's comparison operators (<
, >
, <=
, and >=
) come to the rescue! We can directly compare our Date
objects using these operators to determine their relationship. Let's check out some examples below:
// Comparing if date1 is greater than date2
if (date1 > date2) {
console.log('date1 is later than date2');
}
// Comparing if date1 is less than or equal to date2
if (date1 <= date2) {
console.log('date1 is sooner than or equal to date2');
}
And voilà! You've just compared two dates like a JavaScript pro! 🚀
🌟 Bonus Tip: Comparing Dates with User Input 🤓
To make things more practical, let's consider comparing dates entered by the user in text boxes. We can use the value
property of the text box to retrieve the input value. Here's a quick example:
<input type="text" id="dateInput" />
<script>
const dateInputValue = document.getElementById('dateInput').value;
const userInputDate = new Date(dateInputValue);
if (userInputDate < new Date()) {
console.log('Your date is in the past!');
} else {
console.log('Your date is in the future!');
}
</script>
Now, your code is ready to handle user input and determine whether the provided date is in the past or in the future. 📆
📣 Engage with us! 📣
Congratulations, my friend! You've mastered the art of comparing dates with JavaScript! 🎉 I hope this guide was helpful in resolving your date comparison dilemmas. If you have any questions or need further assistance, feel free to leave a comment below. Let's keep the conversation going! 💬✨
⭐ Your Turn! ⭐
What are your experiences with comparing dates in JavaScript? Have you ever encountered any challenges? We'd love to hear your thoughts and stories! Share them with us in the comments section below and let's geek out together! 🤓💬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
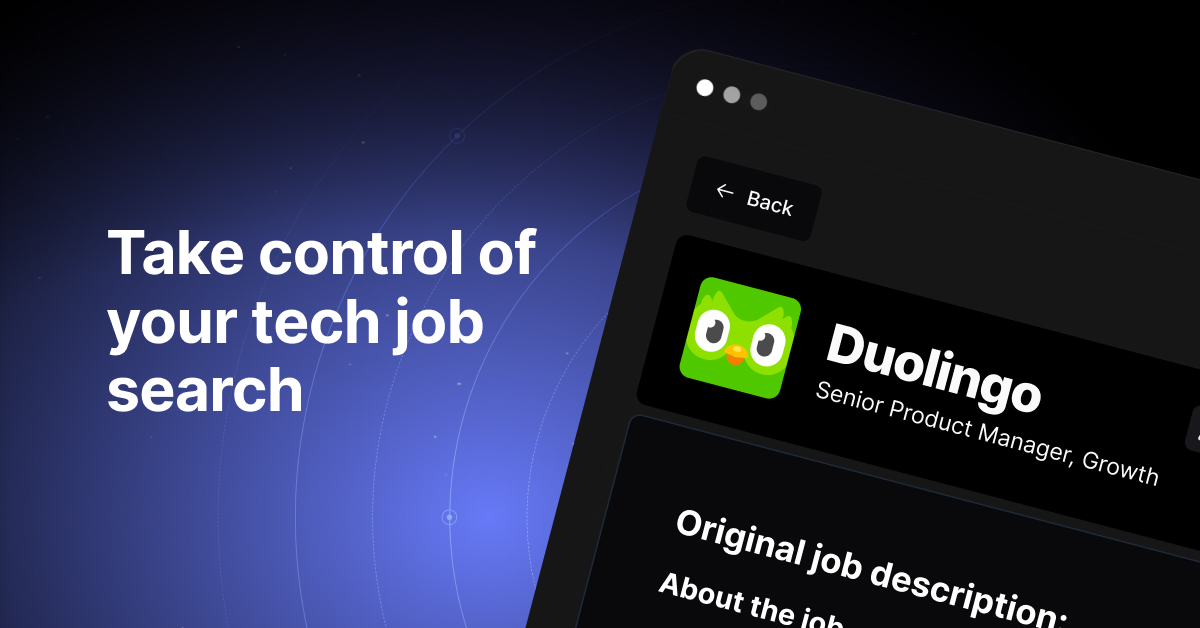