Commonly accepted best practices around code organization in JavaScript
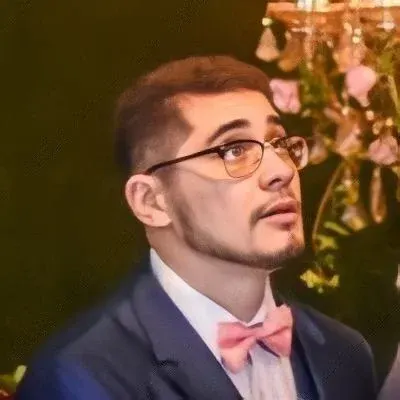
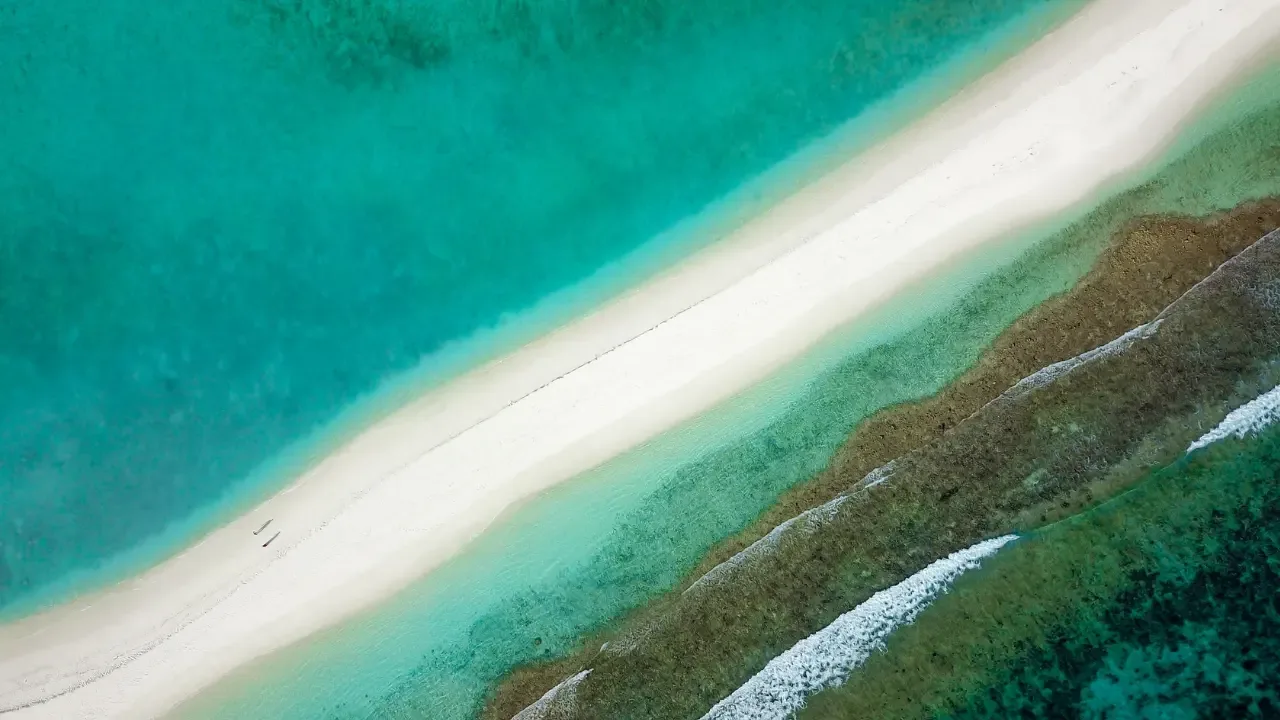
Best Practices for Organizing JavaScript Code
As JavaScript continues its dominance in web development, it's crucial to maintain clean and organized code to ensure maintainability and scalability. In this article, we'll explore some commonly accepted best practices for code organization in JavaScript, addressing the common issue of managing and finding specific code within a project.
The Challenge of Code Organization 😱
With the increasing complexity of JavaScript projects, it's easy for code to become a tangled mess. Here are some common struggles and questions developers often face:
Handler Placement: Should all event handlers be in one spot, or scattered throughout the code?
Function/Class Wrapping: Is it better to wrap functionality in functions or classes for better organization?
Too Many Options: There are multiple ways to achieve the same outcome in JavaScript. What are the current best practices?
Maintainability: How can we organize code to improve readability and make maintenance easier?
1. Utilize Modules or IIFE 📦
One widely accepted best practice is to use modules or Immediately Invoked Function Expressions (IIFE) to encapsulate code. Modules allow you to break down your code into reusable and manageable components.
Example:
// app.js
const myModule = (function() {
// Private variables and functions
const privateVar = 'I am private';
function privateFunc() {
console.log('This is a private function');
}
// Public methods
return {
publicMethod() {
privateFunc();
console.log(`Accessing private variable: ${privateVar}`);
}
};
})();
myModule.publicMethod(); // Output: "This is a private function" and "Accessing private variable: I am private"
By wrapping our code within modules or IIFE, we keep the code organized and hide the implementation details, making it easier to maintain and avoid conflicts with other code.
2. Embrace Namespace Pattern 🗂️
Using the namespace pattern, you can group related code under a common namespace, preventing naming collisions and increasing code organization.
Example:
// myApp.js
const myApp = {
utils: {
formatData(data) {
// code for formatting data
},
validateData(data) {
// code for data validation
}
},
modules: {
// additional modules...
}
};
myApp.utils.formatData(myData);
By structuring code using namespaces, we achieve better organization and logical separation of concerns.
3. Employ a Module Bundler 📦
When working on larger scale projects, employing a module bundler like Webpack or Rollup is highly recommended. These tools allow you to split your code into modules and handle dependencies efficiently. They also optimize the code for better performance and facilitate the separation of concerns.
4. Consistent Code Style ✨
Maintaining a consistent code style across your project is crucial for readability and collaboration. Adopting a linter like ESLint helps enforce a consistent coding style and catches potential errors before they cause issues.
Furthermore, adopting a style guide such as Airbnb JavaScript Style Guide or Google JavaScript Style Guide provides your team with a set of best practices and coding conventions, ensuring a unified approach to code organization.
Conclusion and Call-to-Action 🚀
Organizing JavaScript code is essential for project maintainability and scalability. By utilizing modules, namespaces, module bundlers, and maintaining consistent code styles, you can overcome the challenge of managing and finding code within your project.
We encourage you to share your own experiences and best practices for organizing JavaScript code in the comments section below. Let's collaborate and help each other write better code!
👉 What's your favorite way to organize JavaScript code? Drop your thoughts in the comments! Let's start a conversation!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
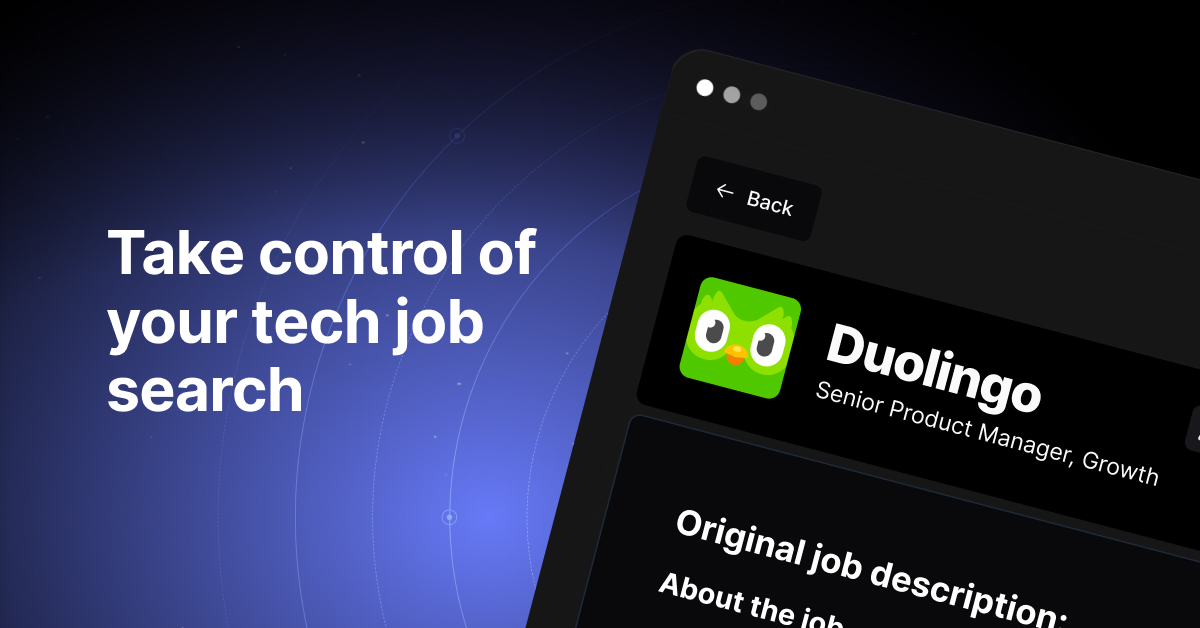