Checking if a key exists in a JavaScript object?
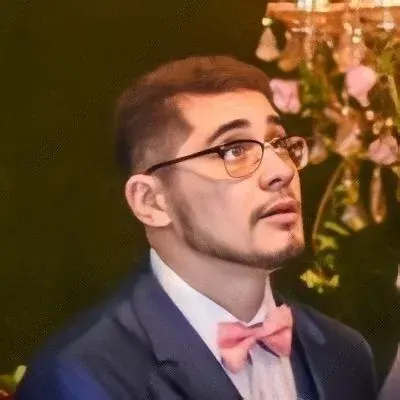
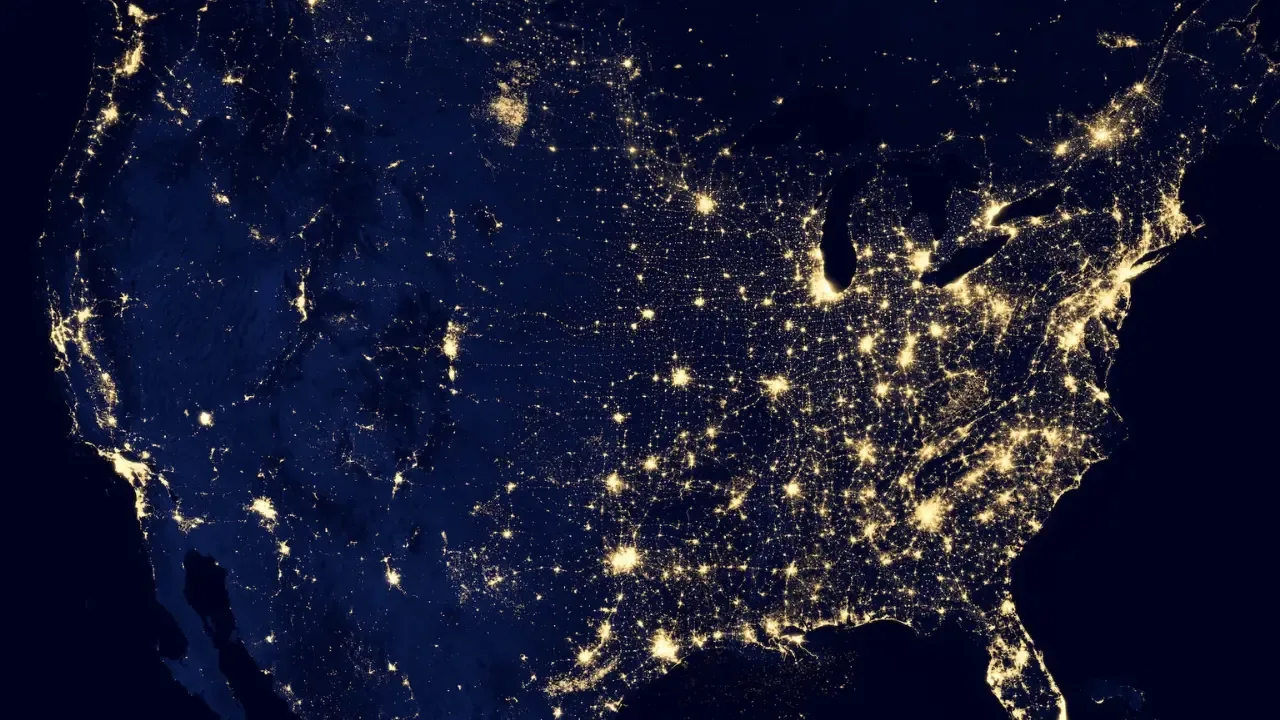
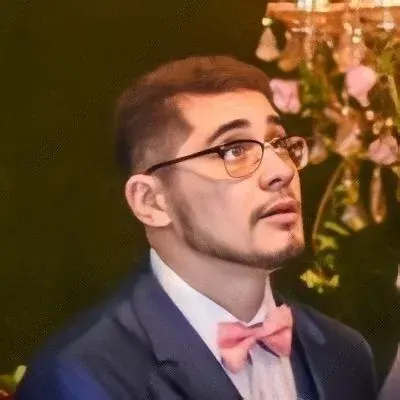
š Checking if a key exists in a JavaScript object?
So, you're working with JavaScript objects and arrays, and you need to know if a particular key exists in them. Don't worry, my friend, I've got you covered! š¤
š¤ The Dilemma: Does Accessing a Nonexistent Key Result in an Error?
When you access a nonexistent key, you might wonder if it returns false
or throws an error. It's a common question, and I'm here to clarify things for you. š§
š Checking for a Key's Existence
To check if a key exists in a JavaScript object or array, you can use the hasOwnProperty()
method or the in
operator. Let me demonstrate both options for you:
š Using the hasOwnProperty() Method:
const myObject = { foo: 'bar', baz: 'qux' };
const keyExists = myObject.hasOwnProperty('foo');
console.log(keyExists); // true
š Using the in Operator:
const myObject = { foo: 'bar', baz: 'qux' };
const keyExists = 'foo' in myObject;
console.log(keyExists); // true
As you can see, both methods return true
if the key exists, and false
if it doesn't.
š” Pro Tips:
Remember to use the object's name followed by the dot (
.
) before callinghasOwnProperty()
. For example:myObject.hasOwnProperty('foo')
.When using the
in
operator, you don't need to use the object's name beforein
. For example:'foo' in myObject
.
š„³ Engage with the Community!
Now that you know how to check for a key's existence in JavaScript objects and arrays, it's time to put your knowledge into practice! Share your favorite method or ask a question in the comments below. š
š” Remember that learning is a journey, and we're here to help each other grow! So let's engage, discuss, and conquer JavaScript together! šŖ
Happy coding! šš©āš»šØāš»