Check if object is a jQuery object
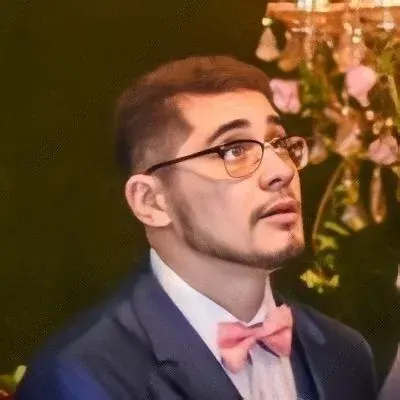
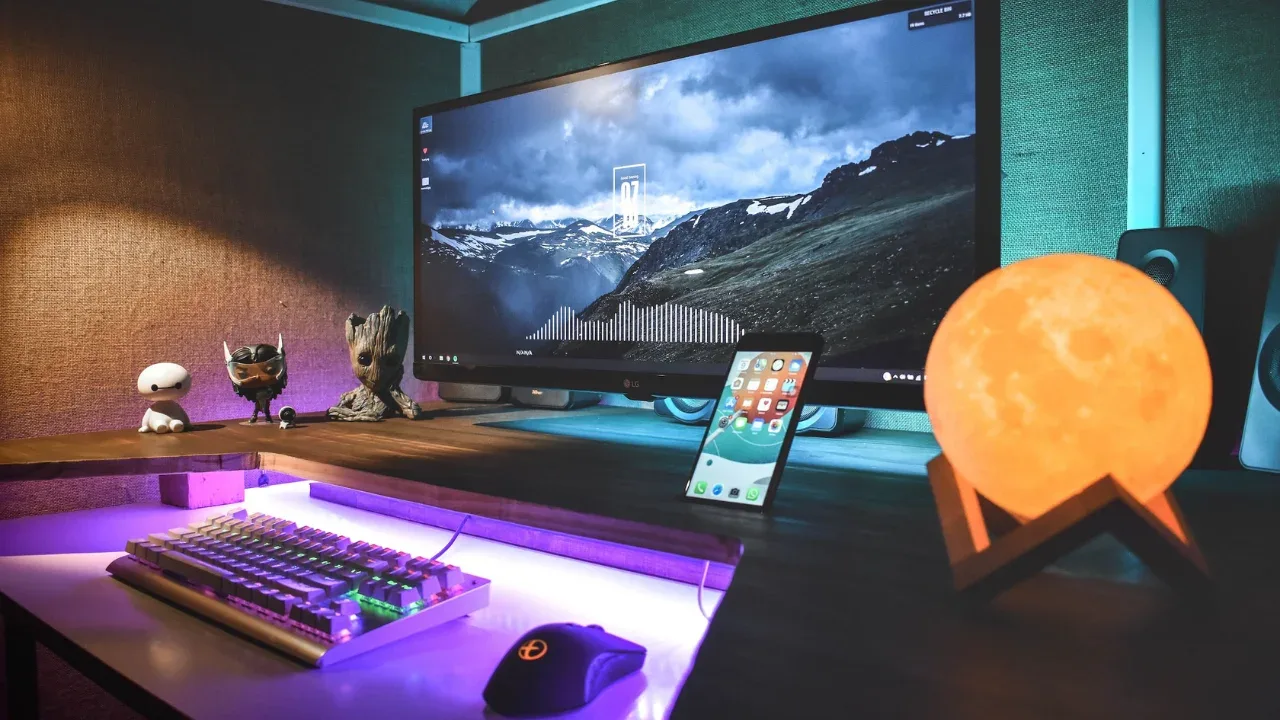
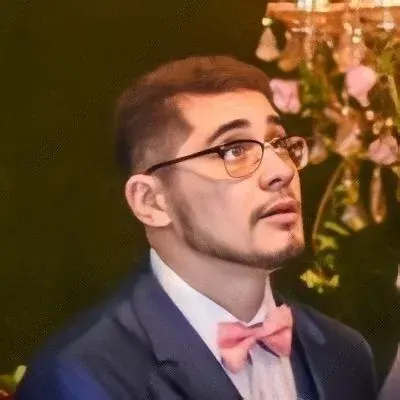
Is it a jQuery Object? Here's How to Check!
π Hey there, tech enthusiasts! Today, we're going to tackle a common issue faced by developers: checking if an object is a jQuery object or a native JavaScript object. π€
Before we dive into the nitty-gritty, let's take a look at the scenario mentioned in the question. We have an object o
and a jQuery object e
, and we want to identify whether they are of different types. Let's see what we have initially:
var o = {};
var e = $('#element');
function doStuff(o) {
if (o.selector) {
console.log('object is jQuery');
}
}
doStuff(o);
doStuff(e);
The code above indeed gets the job done, but it's not entirely safe. π« You could potentially add a selector
key to the o
object and get the same "object is jQuery" result. So, is there a better and more reliable way to determine if an object is a jQuery object? π‘
You might be thinking, "Something like (typeof obj == 'jquery')
should do the trick, right?" π€ Unfortunately, that's not the correct way to handle this situation. The typeof
operator in JavaScript only returns basic data types like 'string'
, 'number'
, 'boolean'
, 'object'
, 'function'
, and 'undefined'
. It cannot specifically detect a jQuery object. π
But don't you worry, my friend! We have a fantastic solution for you. Drumroll, please... π₯ Enter the instanceof
operator! π
The All-Powerful instanceof
The instanceof
operator is what we'll be using to check if an object is a jQuery object. π¦ΈββοΈ Simply follow these steps to put this operator into action:
Firstly, if you haven't already, make sure you have the jQuery library included in your project. If not, you can include it by using a
<script>
tag with the jQuery source CDN or by downloading the library and linking to it locally.Once you have jQuery available, you can start using the
instanceof
operator to check if an object is a jQuery object. Here's how you do it:
if (yourObject instanceof jQuery) {
console.log('You have yourself a jQuery object! π');
} else {
console.log('Oops! It seems like this is a regular JavaScript object. π€');
}
It's as simple as that! Now you can confidently identify a jQuery object using the instanceof
operator. π
Let's Put It to the Test!
To ensure you understand the concept thoroughly, let's go back to the initial example and modify it slightly to incorporate the instanceof
operator. Here's the updated code:
var o = {};
var e = $('#element');
function doStuff(o) {
if (o instanceof jQuery) {
console.log('You have yourself a jQuery object! π');
} else {
console.log('Oops! It seems like this is a regular JavaScript object. π€');
}
}
doStuff(o);
doStuff(e);
By executing the modified code, you will now see more accurate results. π― And most importantly, the chance of mistakenly identifying a regular JavaScript object as a jQuery object is significantly reduced. π ββοΈ
Call to Action: Engage and Share Your Insights!
Now that you've learned about the reliable way to check if an object is a jQuery object, it's time to put this knowledge to use! π
Have you come across any other approaches for verifying object types?
Do you know any potential pitfalls one should be aware of when using the
instanceof
operator?
Share your thoughts, tips, and other valuable insights in the comments below. Let's have a lively discussion and help each other grow as programmers! π€ And don't forget, sharing this blog post with your fellow developers will give them the opportunity to join the conversation too. π
Happy coding! π»