Check if an element is present in an array
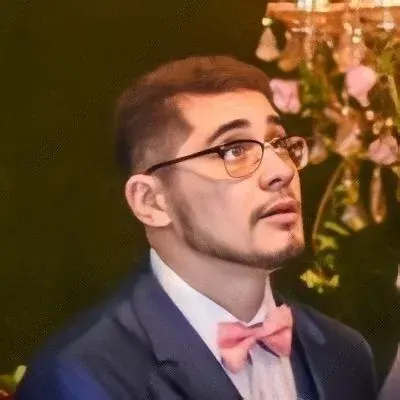
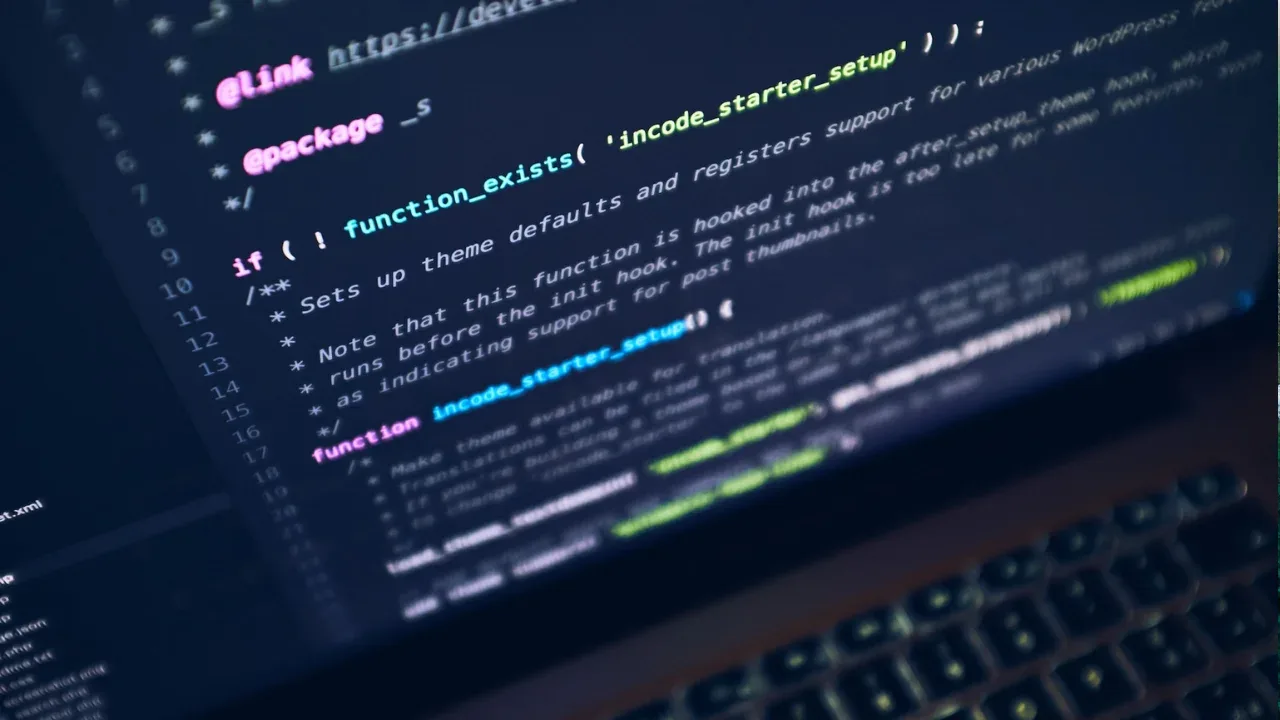
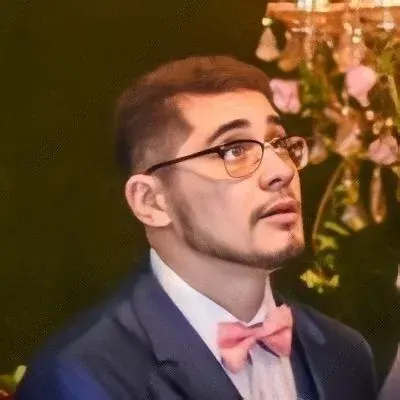
📝🔍✨ Title: How to Check if an Element is Present in an Array - Easy Solutions!
Introduction: Hey there, tech enthusiasts! 👋 In this blog post, we'll tackle a common question: "How can we check if an element is present in an array?" 🔍 We'll explore an existing solution and evaluate if there's a better way to achieve our goal. Let's dive in! 💡
The Existing Solution:
The provided code snippet demonstrates a commonly used function called inArray(needle, haystack)
. Let's take a closer look at how it works:
function inArray(needle, haystack) {
var count = haystack.length;
for(var i = 0; i < count; i++) {
if(haystack[i] === needle) {
return true;
}
}
return false;
}
This function iterates through each element in the haystack
array and checks if any element matches the needle
. If a match is found, it returns true
; otherwise, it returns false
. Simple, right? 😄 Well, let's see if we can improve it!
A Better Solution:
To make our lives easier, JavaScript provides an array method called includes()
. This method can be used to determine if an array includes a certain element, without any need for a loop. Let's check out how we can utilize it:
var haystack = [1, 2, 3, 4, 5];
var needle = 3;
if (haystack.includes(needle)) {
console.log("Element", needle, "is present in the array!");
} else {
console.log("Element", needle, "is not found. 😔");
}
By simply using the includes()
method, we can determine if the needle
exists in the haystack
array. It provides a concise and readable solution, reducing the need for additional code and increasing efficiency. Awesome, right? 🚀
Your Turn: Now that you have a better understanding of how to check if an element is present in an array, go ahead and give it a try! Feel free to apply this knowledge to your own projects and see the magic happen. ✨
Remember, there's always more to discover in the world of technology! So stay curious and keep exploring! 🌟
Conclusion:
In our journey to check if an element is present in an array, we explored an existing solution and identified a better alternative using the includes()
method. This new method not only simplifies our code but also improves its readability and efficiency. 🙌
So why not embrace these improvements and enhance your coding skills? Share this blog post with your fellow tech enthusiasts, and let's make checking elements in an array easier than ever before! 💪🔥
Happy coding! 💻💡