Check if an element contains a class in JavaScript?
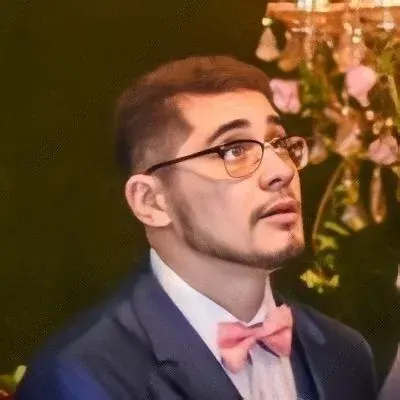
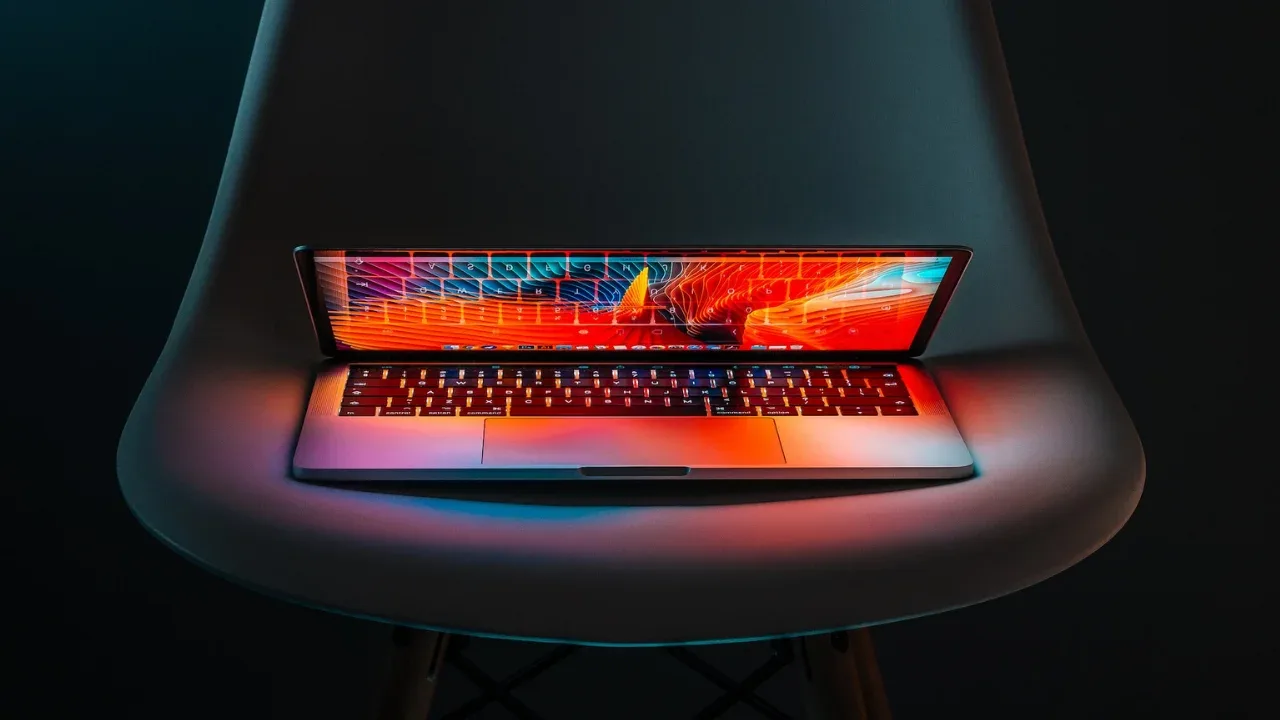
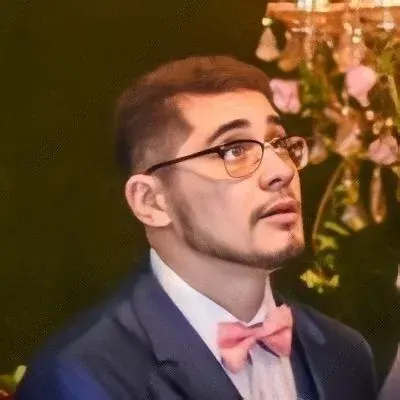
Check if an Element Contains a Class in JavaScript: A Simple Guide 🕵️♂️
Are you tired of not being able to easily check if an element contains a certain class in JavaScript? 🤔 Don't worry, we've got you covered! In this blog post, we'll walk you through the common issues faced by developers when trying to achieve this and provide you with some easy solutions. So, let's dive right in! 💪
The Problem 😫
Let's start by understanding the problem at hand. You might have a situation where you need to check if an element contains a specific class, but the class name alone doesn't cut it. You need an exact match for your code to work as expected. When a class is along with other classes, the exact match doesn't exist anymore, leading to unexpected results. 😱
Let's take a look at an example:
<div id="test" class="class1 class5"></div>
Using the following JavaScript code, you would expect the output to be "I have class1" since the <div>
element contains the .class1
class:
var test = document.getElementById("test");
var testClass = test.className;
switch (testClass) {
case "class1":
test.innerHTML = "I have class1";
break;
case "class2":
test.innerHTML = "I have class2";
break;
case "class3":
test.innerHTML = "I have class3";
break;
case "class4":
test.innerHTML = "I have class4";
break;
default:
test.innerHTML = "";
}
But unfortunately, the output will be an empty string (""
) because the exact match is not found. Pretty disappointing, right? 😢
The Solution 😎
To solve this issue, you need to update your approach. Fortunately, JavaScript provides a handy method called classList.contains()
that allows you to check if an element contains a specific class, regardless of other classes it may have. 🙌
Here's how you can modify your code to use classList.contains()
:
var test = document.getElementById("test");
if (test.classList.contains("class1")) {
test.innerHTML = "I have class1";
} else if (test.classList.contains("class2")) {
test.innerHTML = "I have class2";
} else if (test.classList.contains("class3")) {
test.innerHTML = "I have class3";
} else if (test.classList.contains("class4")) {
test.innerHTML = "I have class4";
} else {
test.innerHTML = "";
}
With this updated code, even if the element has additional classes, it will still recognize the presence of the desired class and display the correct output. 🎉
You can also simplify the code further by using a switch statement:
var test = document.getElementById("test");
switch (true) {
case test.classList.contains("class1"):
test.innerHTML = "I have class1";
break;
case test.classList.contains("class2"):
test.innerHTML = "I have class2";
break;
case test.classList.contains("class3"):
test.innerHTML = "I have class3";
break;
case test.classList.contains("class4"):
test.innerHTML = "I have class4";
break;
default:
test.innerHTML = "";
}
Wrap Up and Engage! 🎉
Congratulations on learning how to check if an element contains a class in JavaScript! Whether you're working on a personal project or tackling a task at work, this knowledge will come in handy. 🚀
Remember, using the classList.contains()
method ensures that you accurately check for the presence of a specific class, even in the presence of other classes. This way, you avoid unexpected bugs and achieve the desired outcome. 🎯
We hope this guide has been helpful to you! If you have any questions or face any issues while implementing this solution, don't hesitate to reach out. Let's get the conversation going! Drop a comment below or share your thoughts on social media using #CheckIfClassExistsInJavaScript. We'd love to hear from you! 😊