Check if an array contains any element of another array in JavaScript
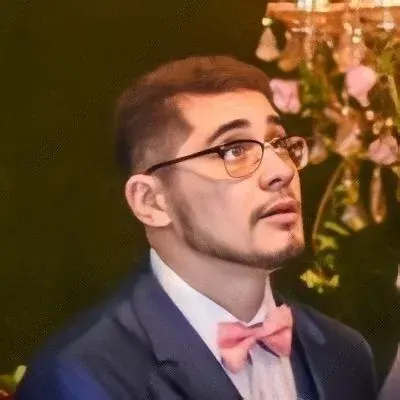
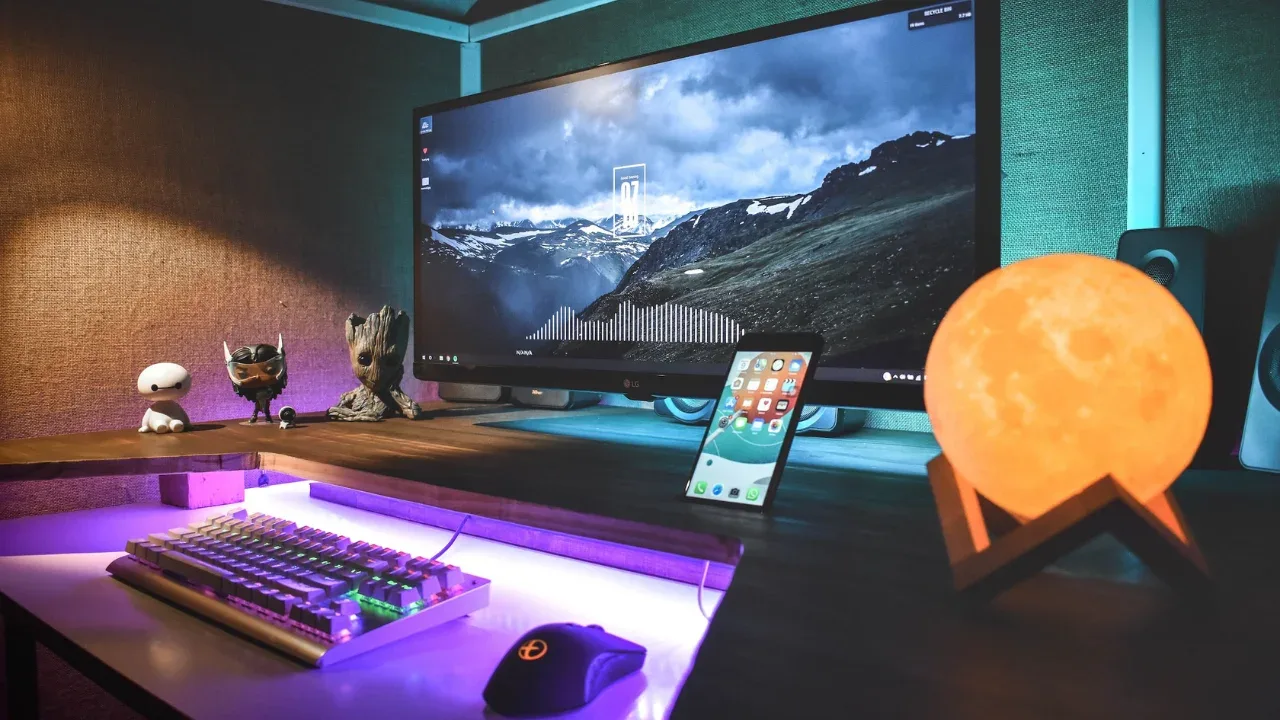
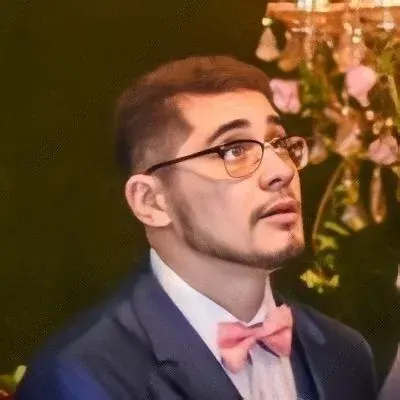
🌟 Easy JavaScript Solution to Check if an Array Contains Any Element of Another Array 🌟
Are you tired of manually searching through arrays to check if they contain any elements from another array? Look no further! In this blog post, we'll explore a simple and efficient solution to solve this problem using JavaScript.
📜 The Problem:
You have a target array ["apple","banana","orange"]
, and you want to determine if any other arrays contain any one of the elements in the target array. For example:
["apple","grape"] // should return true;
["apple","banana","pineapple"] // should return true;
["grape", "pineapple"] // should return false;
💡 The Solution:
To solve this problem, we can leverage the power of JavaScript's built-in array methods. The most straightforward approach is to iterate through each array and check if any element from the target array is present in the current array.
Here's the step-by-step solution:
Start by defining the target array and the other arrays you want to check.
const targetArray = ["apple", "banana", "orange"]; const arraysToCheck = [["apple", "grape"], ["apple", "banana", "pineapple"], ["grape", "pineapple"]];
Next, iterate through each array using the
Array.some()
method. This method stops the iteration as soon as it finds a matching element.const result = arraysToCheck.some(array => { // Check if any element from the targetArray is present in the current array return targetArray.some(element => array.includes(element)); });
Finally, log the result to the console.
console.log(result); // true, true, false
And voilà! With just a few lines of code, you can easily check if any element from the target array exists in the other arrays.
🚀 Try It Yourself:
Feel free to experiment with the code and test it with your own arrays. You can modify the targetArray
and add or remove elements from the arraysToCheck
as needed. Make sure to log the result to see the outcome!
🙌 Your Engagement Matters:
Did you find this solution helpful? Do you have any alternative approaches or more efficient methods? Feel free to share your thoughts, code examples, and suggestions in the comments section below. Let's learn from each other and make coding more fun! 🎉
So, next time you're faced with the challenge of checking if an array contains any element of another array in JavaScript, don't panic! Use this guide as your go-to resource and impress your peers with your coding skills! Happy coding! 💻
🎓 Learn more about JavaScript and other exciting topics on our tech blog. Stay tuned for more insightful articles and tutorials. Don't forget to subscribe to our newsletter! 📬