Check if a variable is a string in JavaScript
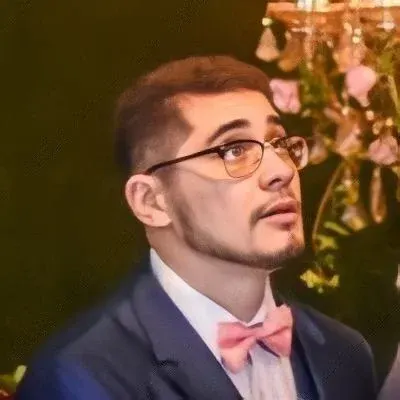

📝 Is it a String or Something Else in JavaScript? Get the Answer Here!
Are you stuck in JavaScript trying to figure out whether that variable is a string or something else entirely? Don't worry, we've got your back! 💪 In this blog post, we will delve into this common issue and provide you with easy solutions to help you overcome this hurdle. So, let's dive in and unravel this mystery together! 🚀
Common Issue: Checking if a Variable is a String
One of the most frequently encountered problems in JavaScript development is determining the type of a variable. In our case, we specifically want to check if a variable is a string or something else, like a number or an object.
Solution 1: typeof Operator 🕵️♀️
To address this issue, JavaScript provides us with the typeof
operator. This operator returns a string that represents the data type of the variable being evaluated. Here's how you can use it to check if a variable is a string:
const myVariable = "Hello, world!";
if (typeof myVariable === "string") {
console.log("It's a string!");
} else {
console.log("It's something else!");
}
In this example, when myVariable
is a string, it will log "It's a string!" to the console. Otherwise, it will log "It's something else!".
Solution 2: Object.prototype.toString() Method 🧱
Another way to determine if a variable is a string is by utilizing the .toString()
method. When called on an object, this method returns a string representing the object's class. By invoking this method on our variable, we can check if it belongs to the String
class:
const myVariable = "Hello, world!";
if (Object.prototype.toString.call(myVariable) === "[object String]") {
console.log("It's a string!");
} else {
console.log("It's something else!");
}
If myVariable
is indeed a string, the code will log "It's a string!" to the console. Otherwise, it will log "It's something else!".
Final Thoughts 💡
Now that you know two easy ways to check if a variable is a string in JavaScript, you can confidently handle similar situations in your coding journey. Remember to select the solution that best suits your specific needs.
So, the next time you find yourself uncertain about the type of a variable, fear not! You have the power to tackle this issue head-on. 🔥
If you found this blog post helpful, feel free to share it with your fellow developers. Also, let us know in the comments if you have any other JavaScript-related questions or if there are specific topics you'd like us to cover in future posts. Your engagement fuels our passion for writing and sharing knowledge! Happy coding! 💻
🎁 If you enjoyed this blog post, check out our latest JavaScript tips and tricks in our weekly newsletter! ➡️ Subscribe 💌
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
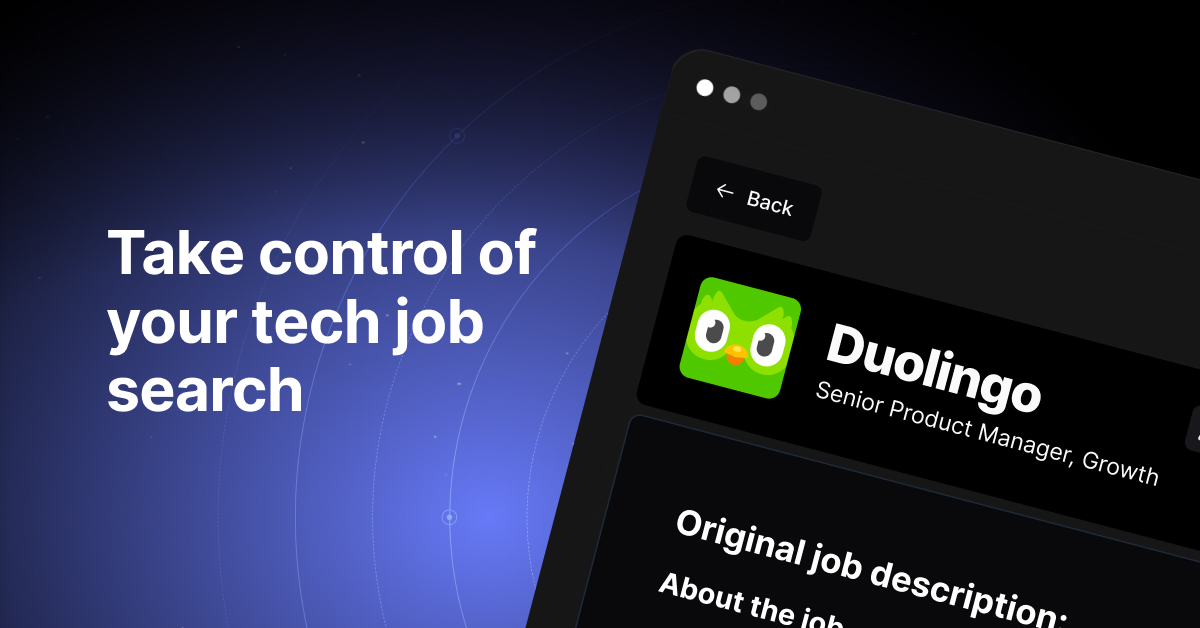