Check if a value is an object in JavaScript
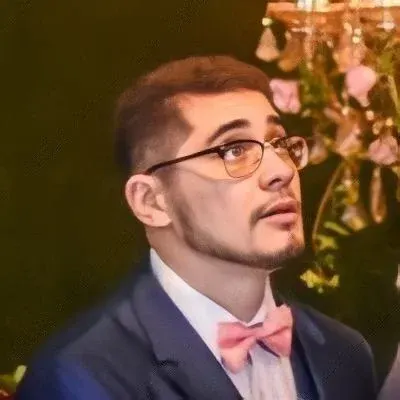
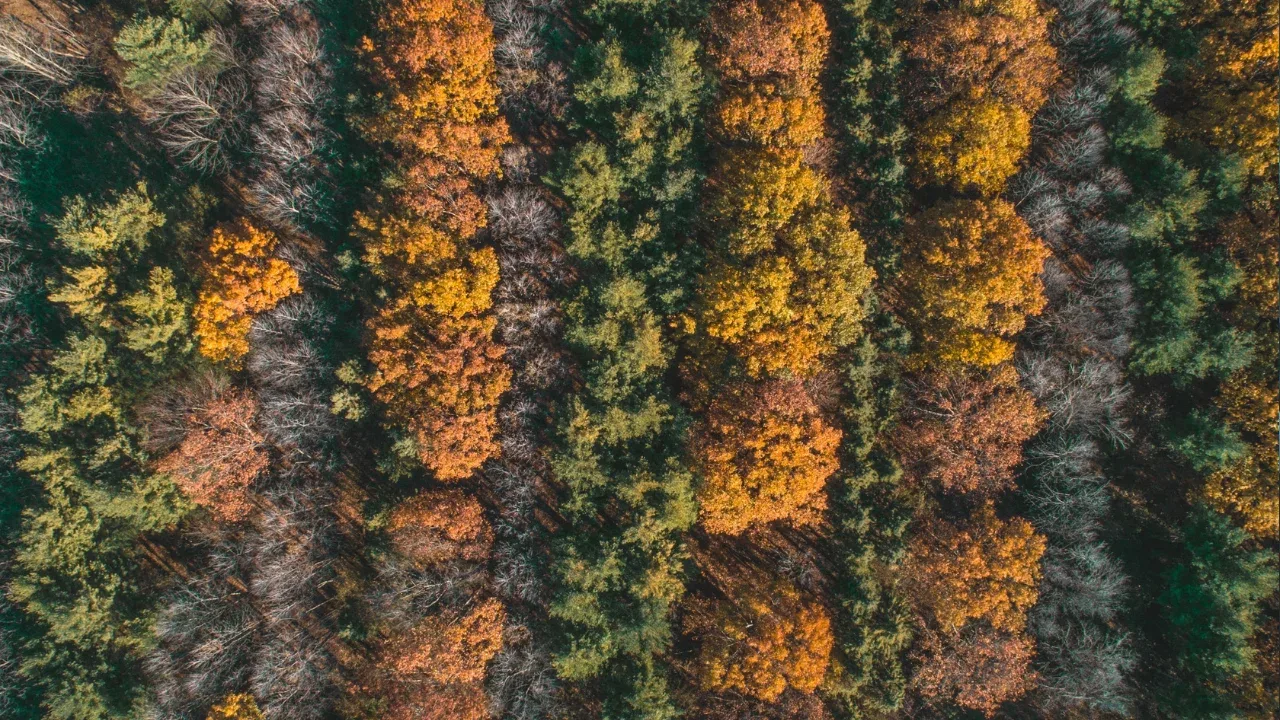
🤔 How to Check if a Value is an Object in JavaScript
JavaScript is a versatile programming language that allows flexibility in data types. When working with code, you may come across situations where you need to check if a value is an object. This can become a bit tricky, as there are different data types and ways to represent objects in JavaScript.
In this blog post, we will explore common issues around checking if a value is an object in JavaScript and provide easy solutions to overcome them. Let's dive in! 💪
📝 The Problem
The question was simple but not as straightforward as it seemed: "How do you check if a value is an object in JavaScript?".
Imagine you have a variable called myValue
and you want to determine whether it contains an object or not. You may be tempted to use a simple comparison operator, like typeof
, to check its type:
const myValue = { name: 'John' };
if (typeof myValue === 'object') {
console.log('myValue is an object!');
}
But, hold your horses! This approach might not always work as expected. 😮
The typeof
operator in JavaScript returns 'object'
for objects, arrays, and even null values. So, even if myValue is an array or null, the condition will still be true. This can lead to unexpected behavior and potential bugs in your code.
💡 The Solution
To accurately check if a value is an object in JavaScript, we can use the following methods:
Object.prototype.toString.call()
method: This method returns a string representing the object's type. By callingtoString
on the object and then checking if it starts with'[object '
, we can verify if the value is an object.const myValue = { name: 'John' }; if (Object.prototype.toString.call(myValue).startsWith('[object ')) { console.log('myValue is an object!'); }
instanceof
operator: Theinstanceof
operator in JavaScript checks if an object is an instance of a particular constructor. By comparing the object against theObject
constructor, we can determine whether the value is an object or not.const myValue = { name: 'John' }; if (myValue instanceof Object) { console.log('myValue is an object!'); }
🙌 Another Approach
If you are using modern JavaScript (ES6+), you can use the typeof
operator with an additional check for null
:
const myValue = { name: 'John' };
if (typeof myValue === 'object' && myValue !== null) {
console.log('myValue is an object!');
}
This approach handles the null
scenario, but keep in mind that it still treats arrays as objects.
💡 Conclusion
Determining whether a value is an object in JavaScript requires careful consideration. Using the right method is crucial to avoid unexpected results. In this blog post, we explored different approaches to solve this problem, including using Object.prototype.toString.call()
, the instanceof
operator, and a modern JavaScript approach.
Remember to choose the method that best suits your needs and use it wisely in your code.
💡 Now it's your turn to apply these learnings! Try checking if a value is an object in your own JavaScript code and share your experience in the comments section below. Have any other tips or tricks? Let's discuss! 👇
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
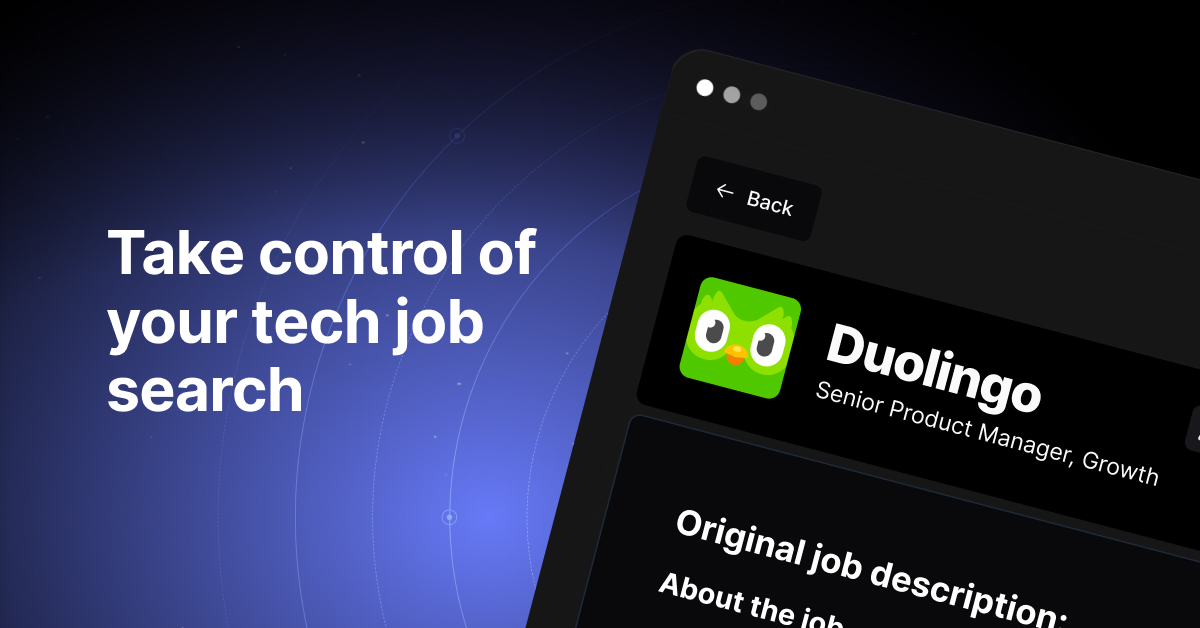