Changing the image source using jQuery
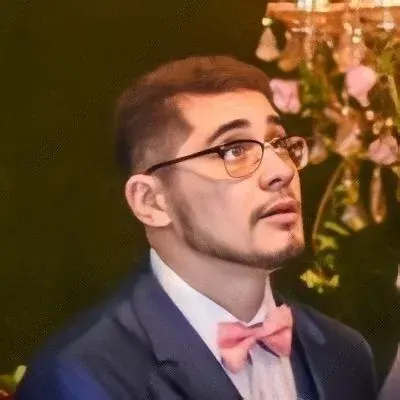
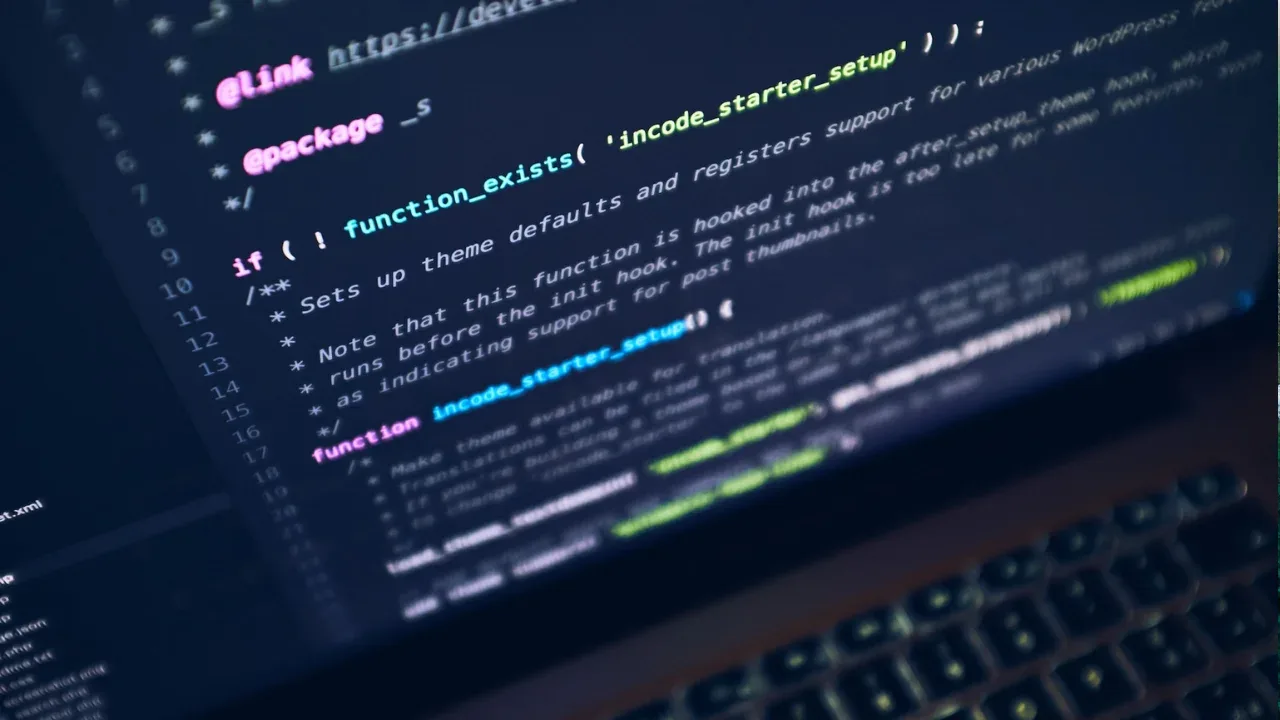
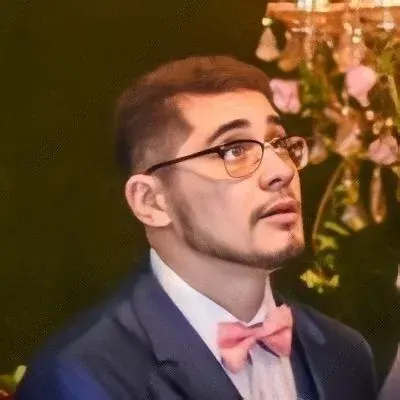
How to Change the Image Source using jQuery
š· š
Have you ever wanted to change the image source dynamically in your web page? Maybe you have a gallery where each image has a different state, and you want to update the source of the image when a user interacts with it. Well, look no further! With jQuery, you can easily achieve this without resorting to CSS. Let's dive into the solution.
The Problem
Let's take a look at the example code snippet you provided:
<div id="d1">
<div class="c1">
<a href="#"><img src="img1_on.gif"></a>
<a href="#"><img src="img2_on.gif"></a>
</div>
</div>
The goal is to change the image source when a user clicks on it. Specifically, we want to replace the "img1_on.gif"
and "img2_on.gif"
with "img1_off.gif"
and "img2_off.gif"
respectively.
The Solution
Using jQuery, we can easily achieve this by attaching a click event listener to each image using the click()
method. Within the event callback function, we can select the clicked image and update its source attribute using the attr()
method. Here's the code:
$(document).ready(function() {
$('img').click(function() {
var imgSrc = $(this).attr('src');
var newImgSrc = imgSrc.replace('_on', '_off');
$(this).attr('src', newImgSrc);
});
});
In the code above, we first select all <img>
elements using the $()
function and attach a click event listener to them using the click()
method. Inside the event callback function, we retrieve the current image source using the attr()
method and store it in the variable imgSrc
. We then replace the substring '_on'
with '_off'
in the source string to get the new image source, which we assign back to the src
attribute using the attr()
method again.
Cool, right? But...
There's one important thing to note. If you have other <img>
elements that you don't want to modify, make sure to add a specific class or ID to the images you want to target. Then, update the selector in the click event listener accordingly. For example:
<a href="#"><img class="image-to-change" src="img1_on.gif"></a>
<a href="#"><img class="image-to-change" src="img2_on.gif"></a>
$(document).ready(function() {
$('.image-to-change').click(function() {
// ...
});
});
Join the Image Source Changing Party!
Now that you know how to change the image source using jQuery, it's time to incorporate this cool feature into your web projects. Whether you want to build an interactive image gallery or have any other use case, jQuery comes to the rescue! Give it a try š
Do you have any other tips, tricks, or suggestions for working with images in jQuery? Share your thoughts in the comments below. Let's learn and grow together! š