change values in array when doing foreach
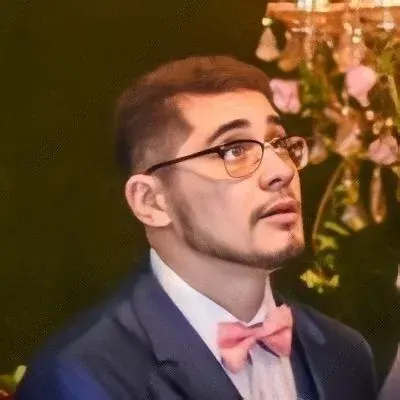
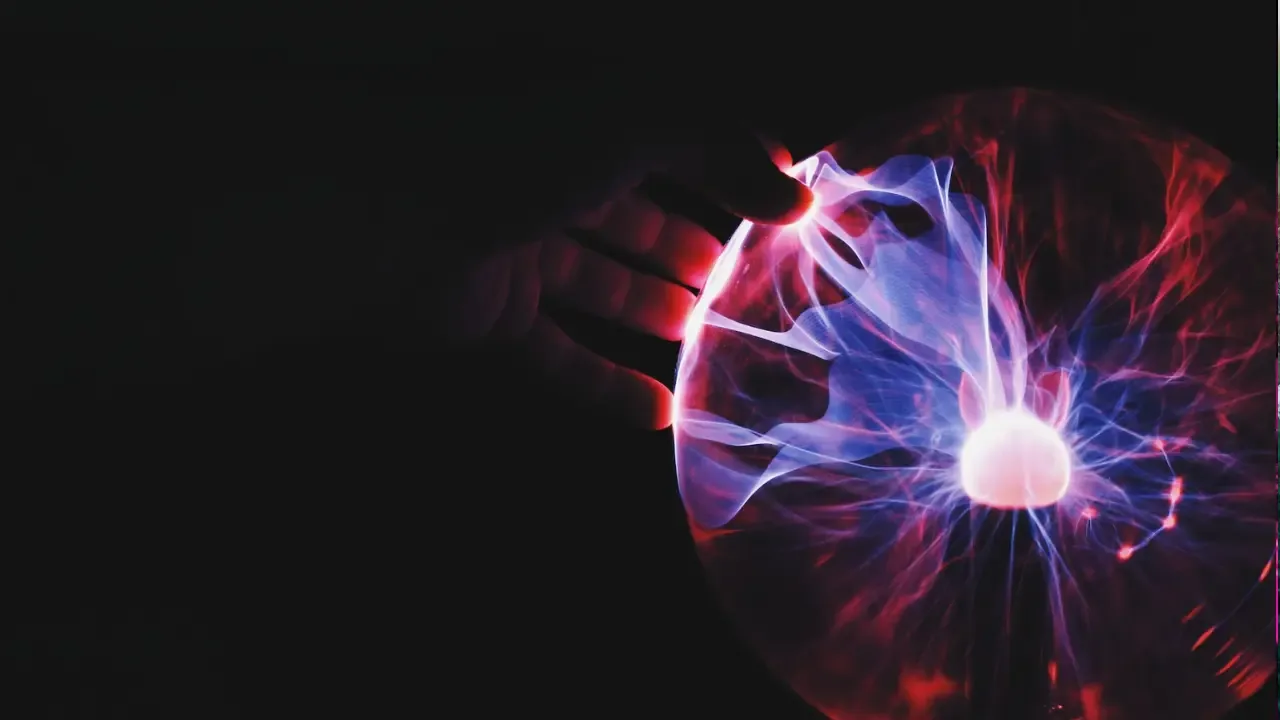
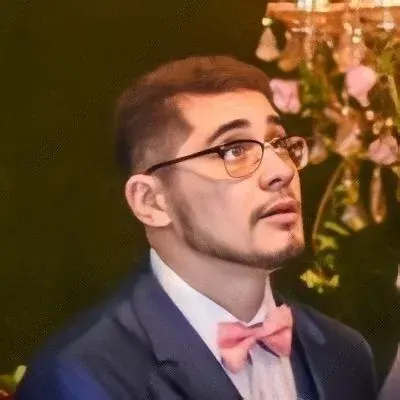
🔄 Changing Values in an Array with foreach - The Easy Way!
Are you tired of trying to change the values in an array within a foreach loop, only to find that the original values remain untouched? 😫 Don't worry, we have a solution for you! In this blog post, we will address this common issue and provide you with easy solutions to change array values while iterating. 🛠️
The Problem
Imagine you have an array with some values that you want to update while looping through it using the forEach
function. Let's take a look at the following example:
var arr = ["one", "two", "three"];
arr.forEach(function(part){
part = "four";
return "four";
});
alert(arr);
In this example, we have an array arr
with three elements: "one", "two", and "three". The forEach
function is used to iterate over each element and update its value to "four". However, when we alert the array after the forEach
loop, we still see the original values instead of the updated ones.
The Explanation
The issue here is that the part
variable inside the forEach
loop is a copy of the array's element rather than a reference to it. When you assign a new value to part
, it only affects the local copy and doesn't update the original array.
The Solution
To change the values in an array while using forEach
, we need to find a way to modify the original array directly. One option is to use the index of each element to update the corresponding value. Here's how you can do it:
var arr = ["one", "two", "three"];
arr.forEach(function(part, index){
arr[index] = "four";
});
alert(arr);
In this updated example, we use the index
parameter of the forEach
function to access and update the values in the original array. Now, when we alert the array after the loop, we can see that all the values have been changed to "four".
The Call-to-Action
Now that you know how to change values in an array while iterating with forEach
, why not give it a try in your own code? Share your experience with us by leaving a comment below, and don't hesitate to ask any questions you may have. Happy coding! 🚀✨