change url without redirecting using javascript
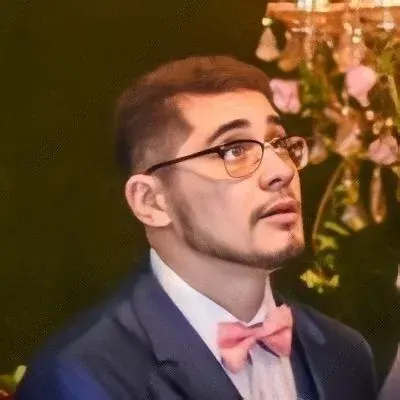
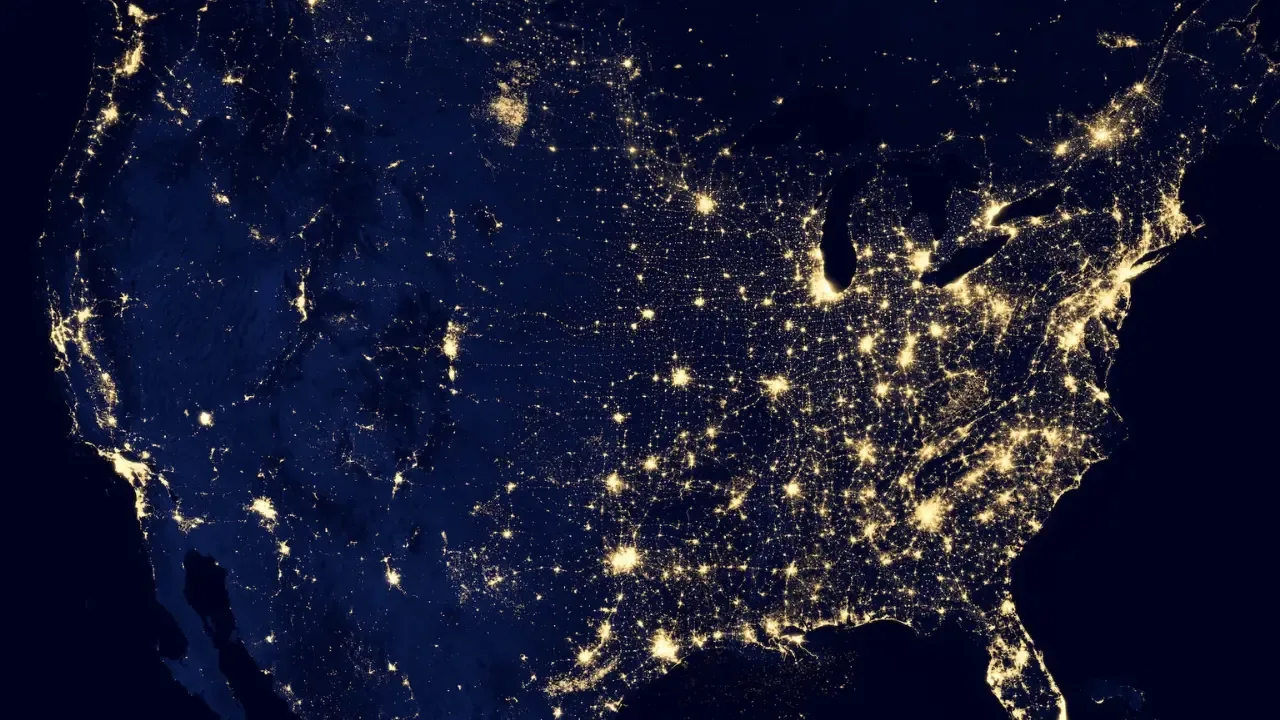
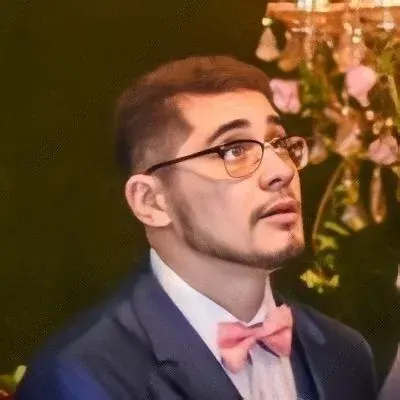
🌟 Changing URL Without Redirecting Using JavaScript 🌟
Ever wonder how some websites magically change the URL in the address bar without refreshing the whole page? Well, you're in luck because today we'll dive into this fascinating topic and provide you with easy solutions. Let's get started! 🚀
🔍 The Problem: Changing URL without Redirecting The challenge is to modify the URL without causing a complete page reload. This can be useful when you want to enhance user experience, create smooth transitions, or maintain a clean URL structure.
💡 The Solution: Leveraging Browser History To change the URL without refreshing the page, we can make use of the HTML5 History API. This API provides methods and events that allow us to modify the browser's history and URL without triggering a full-page reload.
Here's a step-by-step guide on how to achieve this:
Step 1: Pushing State To change the URL, we first need to push a new state to the browser's history using the
pushState
method. This method takes three arguments:state
(an object that represents the new state of the page),title
(a title for the new state), andurl
(the new URL we want to set).window.history.pushState(state, title, url);
Step 2: Listening for Popstate Event We also need to listen for the
popstate
event, which is triggered whenever the user navigates through the browser's history (e.g., clicks the back or forward button). When this event occurs, we can handle updating the page content based on the current URL.window.addEventListener('popstate', function(event) { // Handle URL change here });
Step 3: Handling URL Change Inside the event listener, we can extract the current URL using
window.location.href
and update the page content accordingly based on the URL.window.addEventListener('popstate', function(event) { var url = window.location.href; // Update page content based on the URL });
🔧 Example Implementation: Dekho.com.pk Ads Page You mentioned the website dekho.com.pk as an example. Let's see how they might have implemented changing the URL without redirecting.
// Pushing state to change URL
window.history.pushState(null, null, '/ads-in-lahore');
// Listening for URL change
window.addEventListener('popstate', function(event) {
var url = window.location.href;
// Update page content based on the new URL
});
By pushing a new state with the desired URL and handling the popstate
event, they can update the page content dynamically without performing a full reload.
📣 Your Turn: Get Creative! Now that you have the knowledge, it's time to experiment and implement URL changes without redirects in your own projects. Think about how you can enhance your user interfaces, create smoother transitions, or improve the overall user experience.
🙌 Share Your Experiences Have you encountered fascinating implementations of changing URLs without redirects? Do you have any tips or tricks to add? We'd love to hear from you! Share your thoughts, ideas, and experiences in the comments below and let's keep the conversation going. Happy coding! 😄