Casting a number to a string in TypeScript
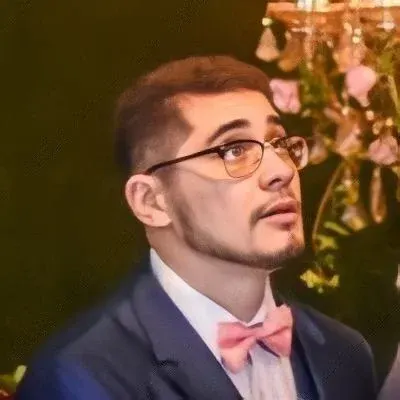
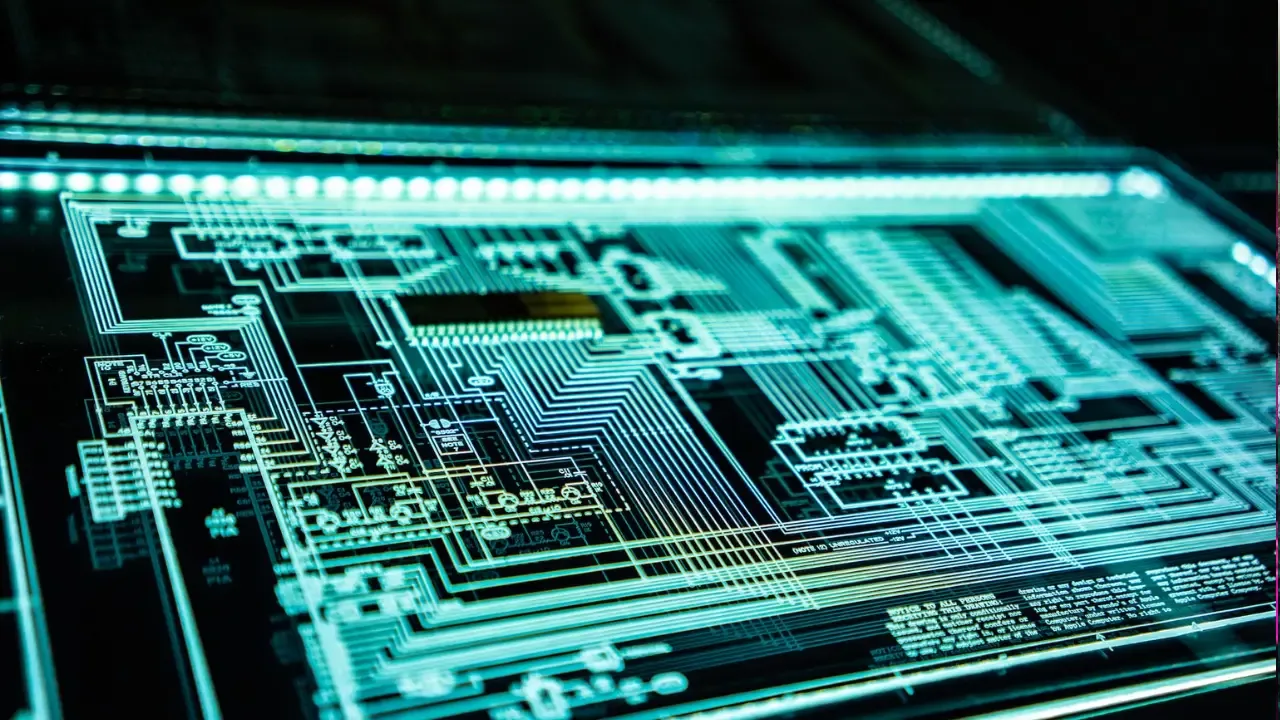
📖 Blog Post: Casting a Number to a String in TypeScript
Have you ever encountered the need to convert a number into a string in TypeScript? Perhaps you came across an error message like "Type 'number' is not assignable to type 'string'". Don't worry, you're not alone! In this blog post, we will explore different ways to effectively cast a number to a string in TypeScript and provide you with easy solutions to overcome this common issue. Let's dive in! 💦
The Problem: Assigning a Number to a String
Consider the following scenario, where you want to assign a number value to the window.location.hash
property which expects a string.
var page_number: number = 3;
window.location.hash = page_number; // Error: Type 'number' is not assignable to type 'string'
Here, the TypeScript compiler throws an error since it detects an attempt to assign a numeric value to the location.hash
, which only accepts strings.
Easy Solutions: Casting Methods
Luckily, TypeScript provides us with multiple ways to cast a number to a string. Let's explore two common methods to accomplish this casting:
Casting using the
""
Literal
By concatenating the empty string ""
with the number, we can easily convert it to a string.
window.location.hash = "" + page_number; // Casting using "" literal
In this method, the empty string acts as a catalyst, triggering the conversion of the number to a string. This simple yet effective approach quickly solves the casting issue.
Casting using the
String()
Function
Alternatively, you can also use the String()
function to explicitly convert the number to a string.
window.location.hash = String(page_number); // Casting using the String() function
By passing the number as an argument to the String()
function, TypeScript converts it to a string representation. This method provides a more explicit way of casting and is equally effective in resolving the problem.
Which Method is Better?
Both casting methods mentioned above are valid and will successfully cast a number to a string in TypeScript. The choice depends on your personal preference and the specific requirements of your codebase. Some developers prefer the syntactic simplicity of using " "+number
, while others prefer the explicitness of the String()
function. Ultimately, the two methods achieve the same result.
📣 Call to Action: Share Your Preferred Method
What casting method do you prefer when converting a number to a string in TypeScript? Do you have any additional tips or insights related to this topic? We would love to hear from you! Share your thoughts in the comments below and let's engage in a lively discussion. Together, we can learn and grow as TypeScript developers! 👩💻👨💻
Remember, casting a number to a string in TypeScript doesn't have to be a daunting task. With the help of these easy solutions, you can resolve common issues and write clean, error-free code. Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
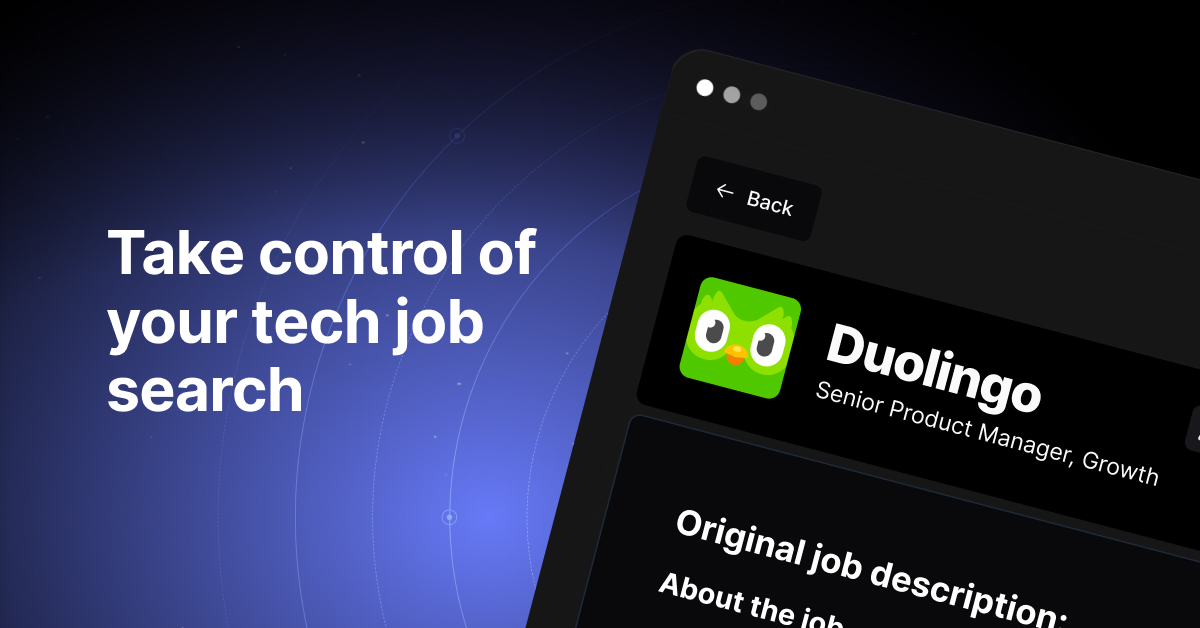