Capture HTML canvas as GIF/JPG/PNG/PDF?
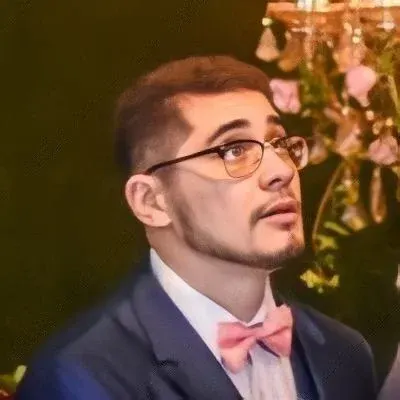
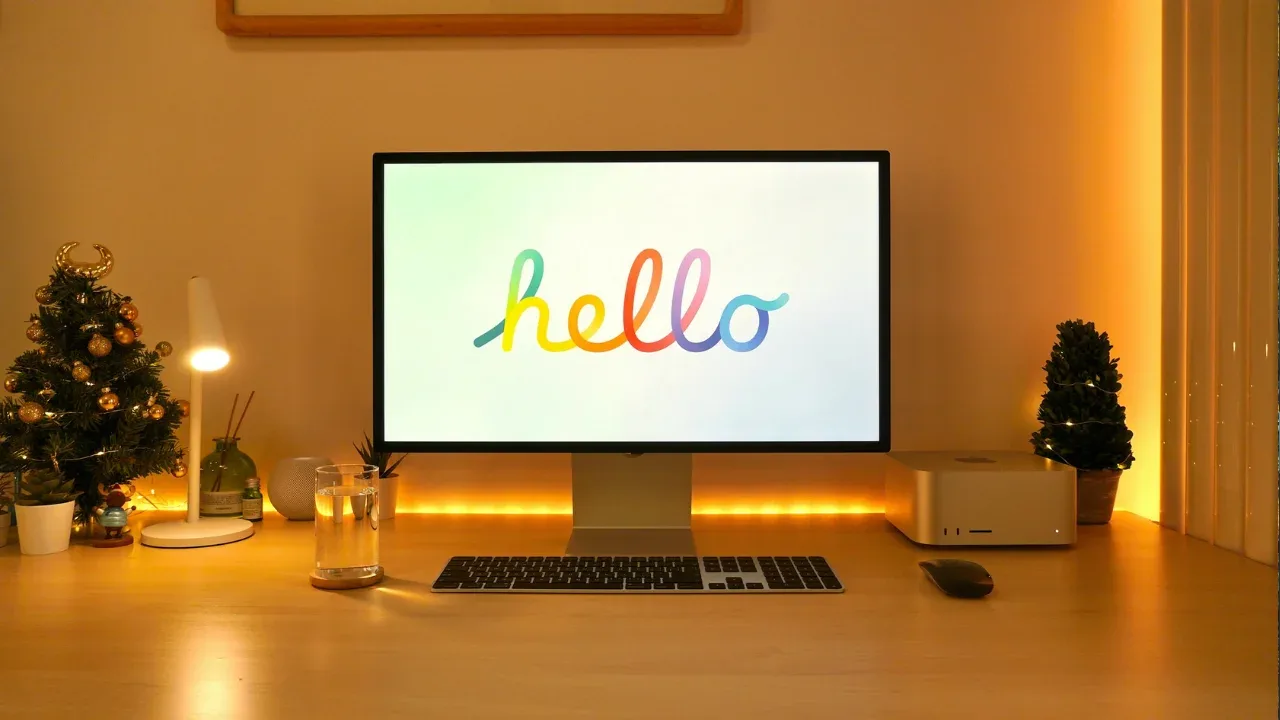
How to Capture HTML Canvas as GIF/JPG/PNG/PDF?
š Do you want to capture or print what's displayed in an HTML canvas as an image or PDF? It might seem like a challenging task, but fear not! We've got you covered with this complete guide. š
The Challenge
šŖļø "Is it possible to generate an image via canvas and convert it into a PNG?" This is a common question many web developers ask. Fortunately, the answer is a resounding YES! š
The Solution
š” To capture the content of an HTML canvas, you need to follow a few steps. Let's explore three different methods for capturing the canvas as a GIF, JPG, PNG, or PDF. Choose the method that suits your needs best. šŖ
Method 1: Using toDataURL()
šØ The toDataURL()
method allows you to convert the contents of a canvas into a Data URL. It returns a base64-encoded string representing the image. To capture the canvas as an image, follow these steps:
const canvas = document.getElementById('myCanvas');
const image = new Image();
image.src = canvas.toDataURL('image/png'); // Replace 'image/png' with 'image/jpeg' for JPG
document.body.appendChild(image);
š„ This method creates an Image
object with the source set to the Data URL of the canvas. You can also change the image format by modifying the argument passed to toDataURL()
.
Method 2: Using toBlob()
š¦ The toBlob()
method allows you to create a Blob object from the contents of a canvas. This isn't supported in all browsers, so be sure to check browser compatibility. Here's how you can capture the canvas as an image file using toBlob()
:
const canvas = document.getElementById('myCanvas');
canvas.toBlob(function (blob) { // Callback function receives the Blob object
const link = document.createElement('a');
link.href = URL.createObjectURL(blob);
link.download = 'canvas.png'; // Change the filename as needed
link.click();
});
š±ļø This method generates a Blob object from the canvas and creates a download link dynamically. When the link is clicked, it triggers the download of the captured canvas as an image file.
Method 3: Using a Library
š If you don't want to reinvent the wheel, you can use existing libraries like html2canvas or dom-to-image. These libraries provide additional features and cross-browser support for capturing the canvas as an image or PDF.
š” Remember to include the library script in your HTML, follow their documentation, and adapt the code according to your needs.
š Harness the Power of HTML Canvas
šø With these methods at your fingertips, you can now capture the content of an HTML canvas as a GIF, JPG, PNG, or even a PDF! šØš
šŖ Choose the method that best suits your requirements and get creative. Whether you're creating a signature pad, a chart, or a dynamic visualization, the possibilities are endless when you harness the power of HTML canvas.
š Share your thoughts and experiences in the comments below. We'd love to hear how you've used canvas captures in your projects! š¬š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
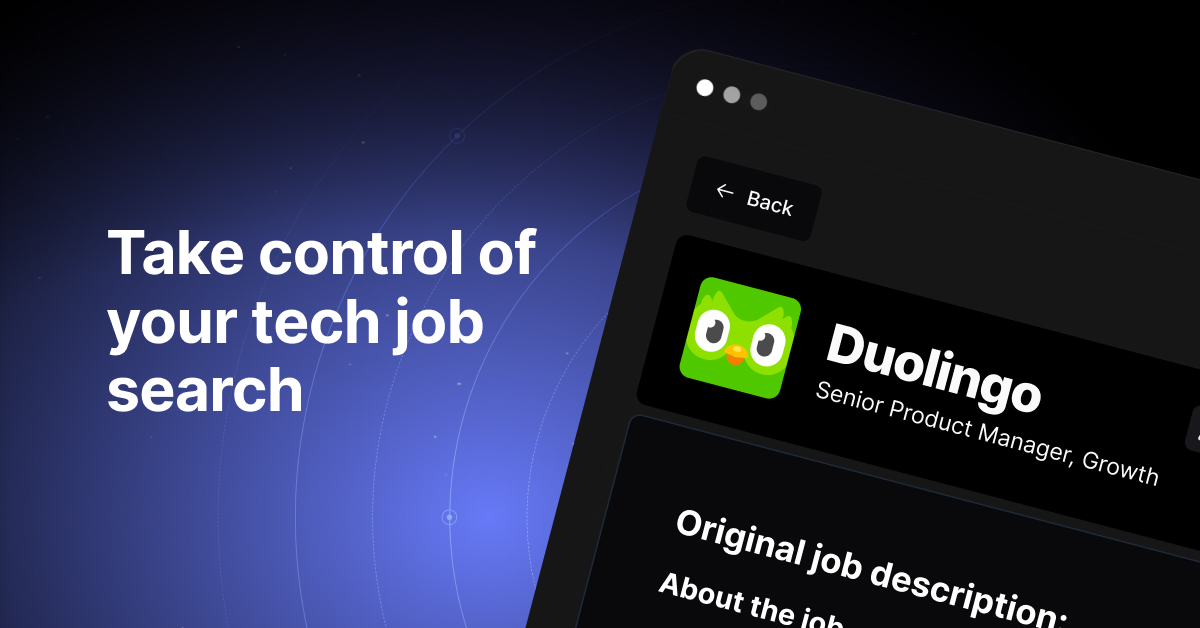