Can"t perform a React state update on an unmounted component
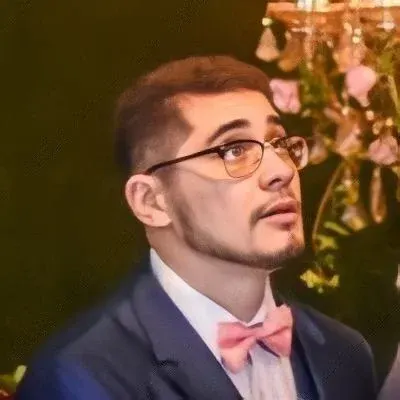
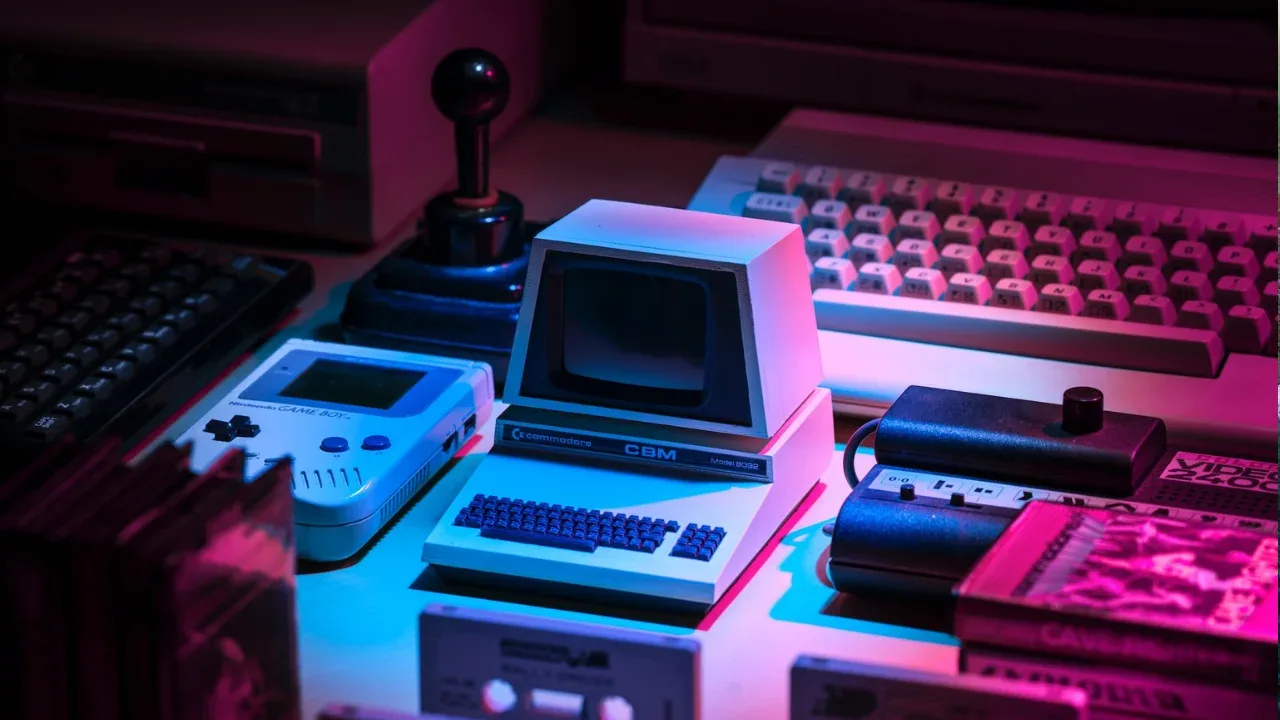
🚀 How to Fix the React State Update Issue on an Unmounted Component
Are you encountering the dreaded "Can't perform a React state update on an unmounted component" error in your React application? Don't worry, you're not alone! This error occurs when you try to update the state of a component that has already been unmounted.
In this blog post, we'll dive deep into this common issue and provide you with easy solutions to fix the problem. Let's get started! 💪
🤔 Understanding the Problem
Before we jump into the solutions, let's take a closer look at what causes this error. Typically, it occurs when you call setState()
after the componentWillUnmount()
lifecycle method has been called.
In the provided code snippet, the error originates from the AutoWidthPdf
component, specifically in the onDocumentComplete()
method of the Book
component.
🔍 Identifying the Culprit
The stack trace in the browser console can be overwhelming, but let's decipher it together. From the stack trace, we can see that the component responsible for the error is TextLayerInternal
. It is likely triggered by an asynchronous task or event handler.
💡 Solution: Preventing the Error
To fix the error and prevent it from occurring, we need to ensure that any running asynchronous tasks or event handlers are properly canceled or cleaned up when the component is unmounted.
Solution 1: Clear Async Tasks and Event Listeners
In the componentWillUnmount()
method of the Book
component, remove the event listener and cancel any running asynchronous tasks. Here's an updated version of the code:
componentWillUnmount = () => {
window.removeEventListener("resize", this.setDivSizeThrottleable);
this.setDivSizeThrottleable.cancel();
};
Solution 2: Check if Component is Mounted
In the onDocumentComplete()
method, you can add a simple check to see if the component is still mounted before updating the state. Here's an example:
onDocumentComplete = () => {
if (this.isComponentMounted) {
this.setState({ hidden: false });
this.setDivSizeThrottleable();
}
};
To implement this solution, add the isComponentMounted
property to the Book
class and set it to true
in componentDidMount()
, and false
in componentWillUnmount()
.
🚀 Take Action!
Now that you have learned how to fix the React state update issue on an unmounted component, it's time to apply these solutions to your own code. Remember to analyze the stack trace, clean up async tasks, and add the necessary checks to avoid updating the state on unmounted components.
If you found this blog post helpful, share it with your fellow developers! Let's spread the knowledge and overcome React challenges together. 🌟
If you have any questions or other React-related topics you'd like us to cover, drop us a comment below. We'd love to hear from you and continue guiding you on your coding journey. Happy coding! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
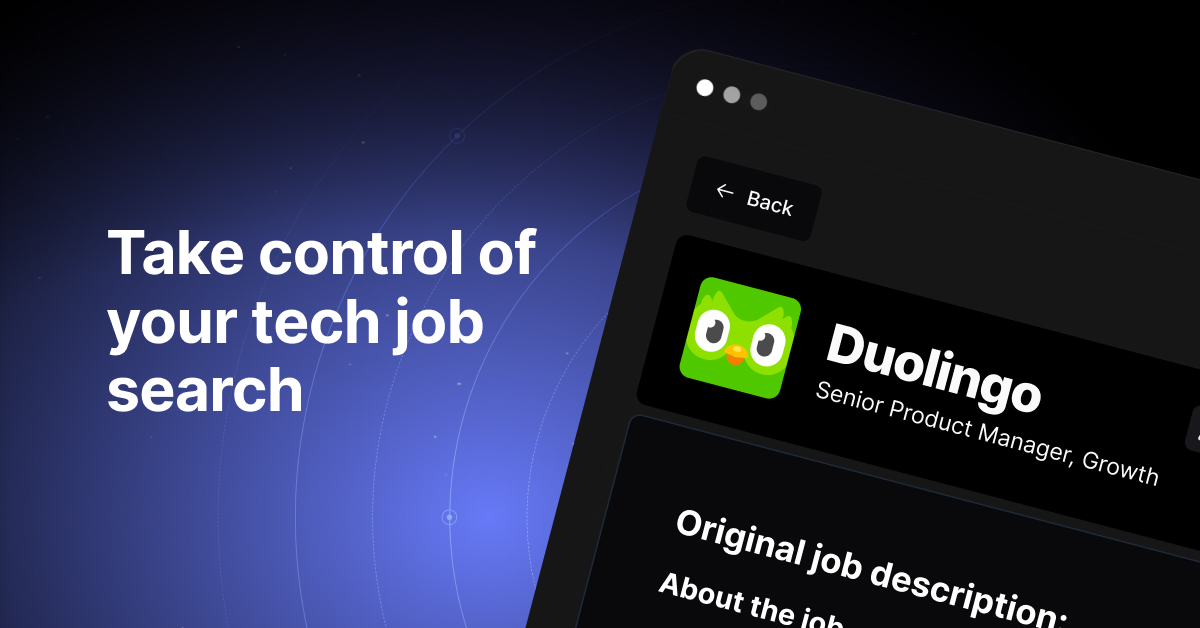