Cannot update a component while rendering a different component warning
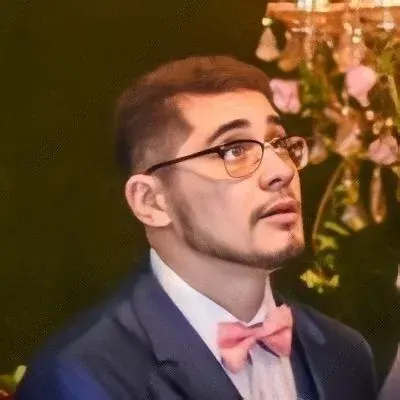
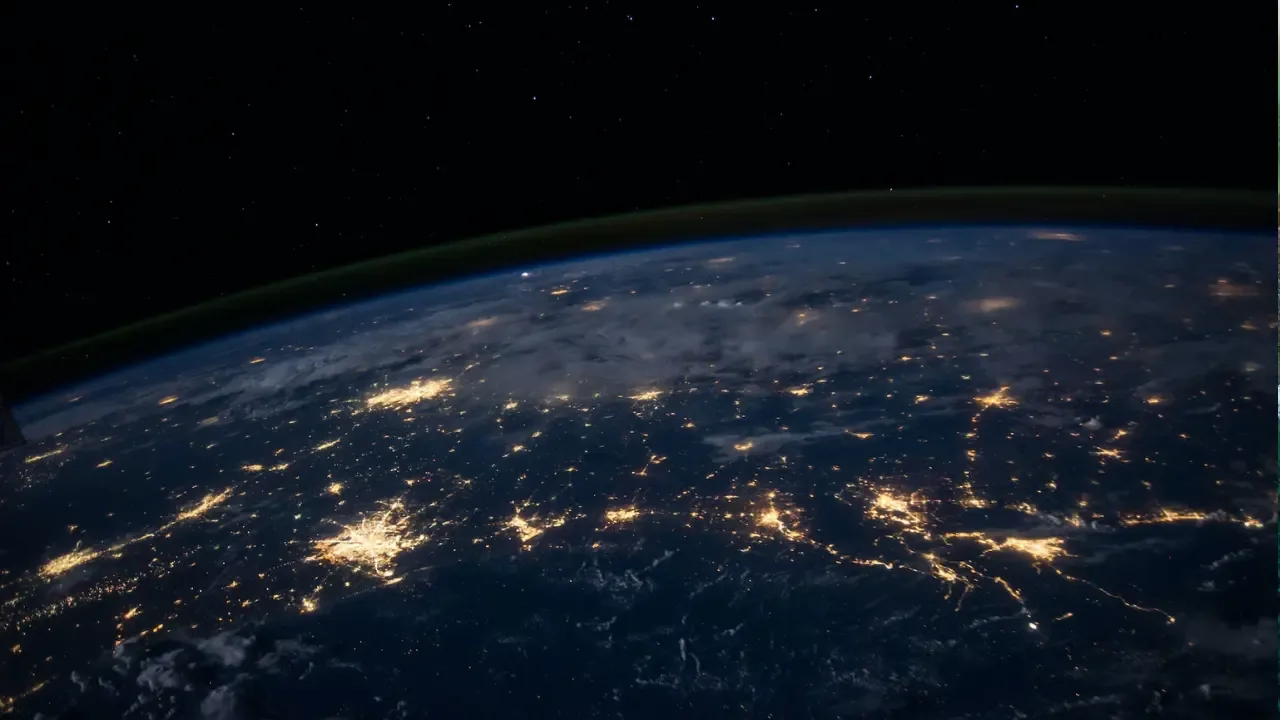
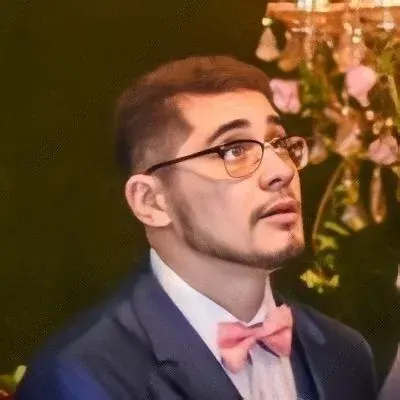
⚠️ Cannot update a component while rendering a different component warning
So you're getting the dreaded "Cannot update a component while rendering a different component" warning in your React application. Don't panic! This warning typically occurs when you're trying to update the state of a component while rendering a different component at the same time. 👀
Common Issues 🤔
One common cause of this warning is when you're using Redux and dispatching actions inside your components. Looking at your code snippet, we can see that the culprit might be the resetRegisterStatus()
action being dispatched inside the render
method of your Register
component. When you call this.props.dispatch(resetRegisterStatus())
inside the render
method, it triggers a state update while the component is being rendered. This violates React's rendering lifecycle and causes the warning to be thrown. 😱
Easy Solutions 💡
To resolve this issue, we need to ensure that state updates are not triggered during the rendering process. Here are a few possible solutions:
Move the state update outside the
render
method:Instead of calling
this.props.dispatch(resetRegisterStatus())
insiderender
, move it to another lifecycle method such ascomponentDidUpdate
or trigger it in response to user actions.
Use
setState
asynchronously:Make use of React's
setState
method to update the state asynchronously and avoid triggering state updates during rendering. For example, you can update the state in thehandleSubmit
method of yourRegisterForm
component usingthis.setState({errorMsg: this.props.registerError})
.
Consider refactoring your code structure:
If the above solutions don't work, it might be worth reconsidering the overall structure of your components and their responsibilities. It's generally a good practice to keep your components focused and responsible for a specific task.
Call-to-Action 📣
Have you encountered the "Cannot update a component while rendering a different component" warning in your React application? What solutions have you tried? Share your experiences and tips in the comments below! Let's help each other overcome this common React challenge. 🔥
Remember, keeping your state updates separate from the rendering process is key to avoiding this warning. Happy coding! 💻💪
References:
🚀🔥💻