Can I update a component"s props in React.js?
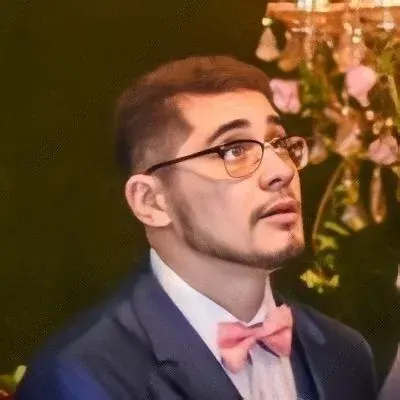
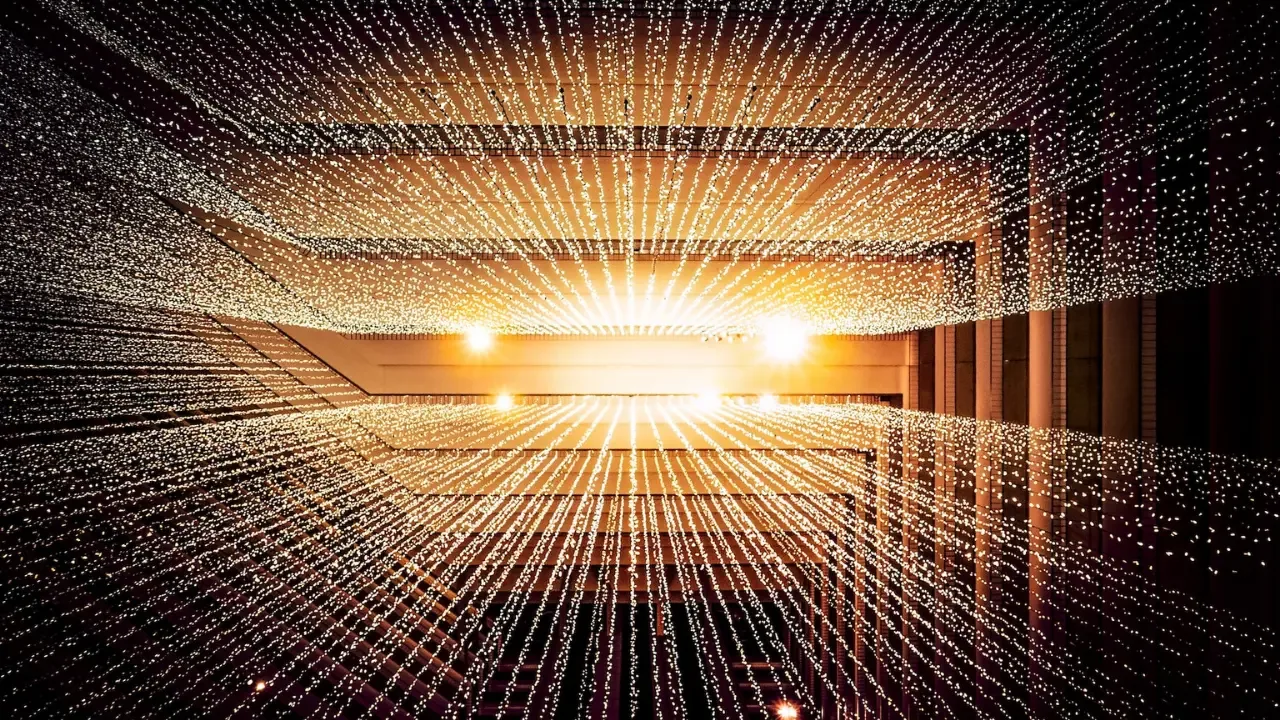
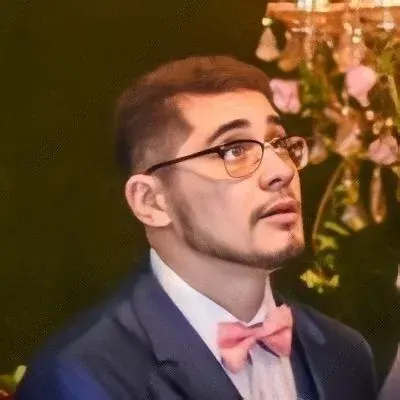
📝 Updating Component's Props in React.js
If you're starting to work with React.js, you may wonder how to update a component's props. Props are typically considered static, passed in from the parent component, while state changes based on events. However, React does provide a way to update props in certain scenarios.
Understanding componentWillReceiveProps
In the React documentation, there is a reference to the componentWillReceiveProps
lifecycle method, which is invoked when a component is receiving new props. Here's an example:
componentWillReceiveProps: function(nextProps) {
this.setState({
likesIncreasing: nextProps.likeCount > this.props.likeCount
});
}
This code snippet updates the component's state based on the comparison of nextProps
to this.props
. It indicates that props can indeed change, but there are some important points to consider.
When Can Props Change?
Props in React are usually passed down from parent components, and they should be treated as read-only within the child component. However, there are specific scenarios where props can be updated:
When a parent component re-renders: If a parent component re-renders due to changes in its own state or props, the child component will receive the updated props.
When parent component forces a re-render: The parent component can explicitly re-render the child component by passing new props to it.
In both cases, the componentWillReceiveProps
method will be called with the new props, allowing you to update the component's state based on the new props.
Caveats and Best Practices
While updating props is possible, it's important to note a few caveats and best practices:
Don't rely on props for local state: Generally, it's recommended to use props for one-time initialization and rely on state for managing component-specific changes.
Avoid excessive prop updates: Updating props too frequently can lead to performance issues. Only update props when necessary, and use shouldComponentUpdate lifecycle method to optimize rendering.
Consider using React hooks: If you're using React version 16.8 or above, using hooks such as
useState
anduseEffect
can simplify state management and reduce the need to update props directly.
Takeaways
Props in React are primarily intended to be passed down from parent components and treated as read-only within child components. However, React provides the componentWillReceiveProps
method to update state based on changes in props. It's important to use this feature judiciously and consider other alternatives such as managing state locally or utilizing React hooks.
Remember, React is all about creating reusable and composable components, so make sure to design your components in a way that minimizes prop updates and optimizes performance.
Now that you have a better understanding of updating props, go ahead and build amazing React applications with ease!
🙌 Have you encountered situations where you needed to update props in React? Share your experiences and thoughts in the comments below! Let's learn together! 😊