Can I execute a function after setState is finished updating?
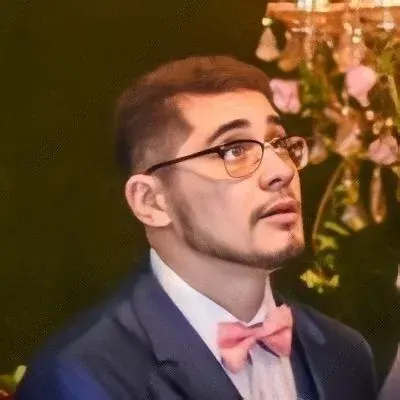
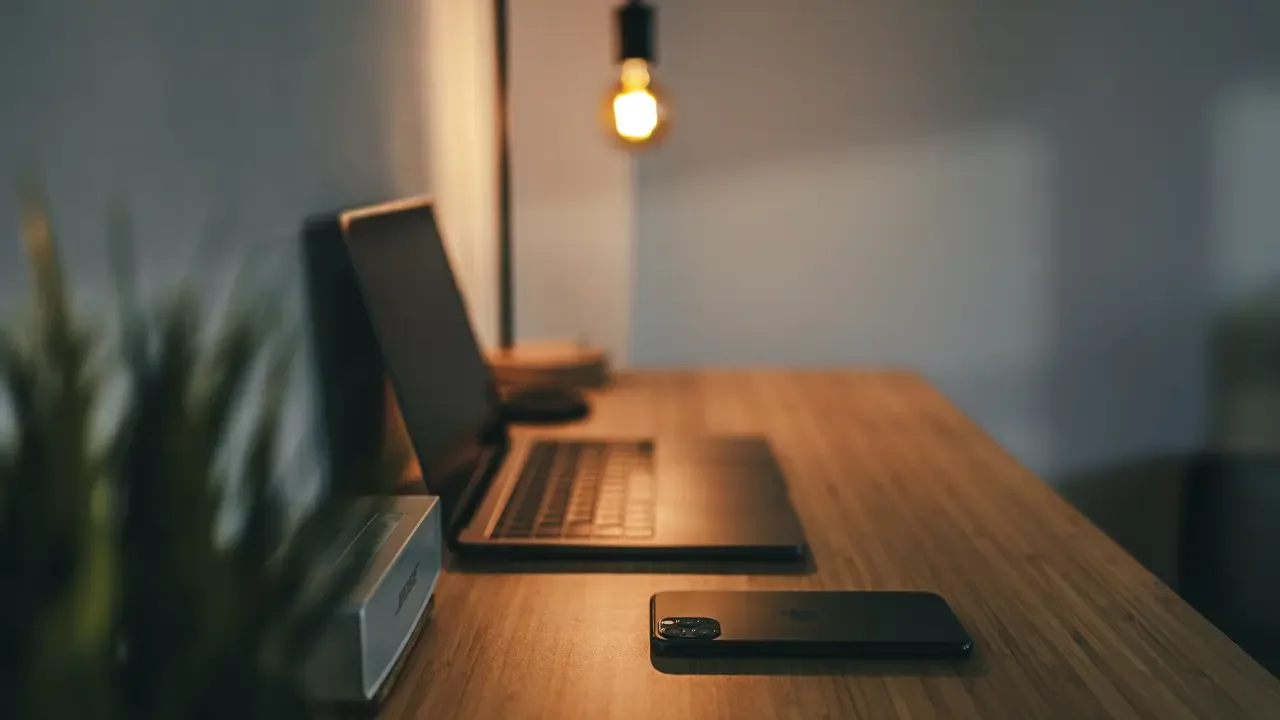
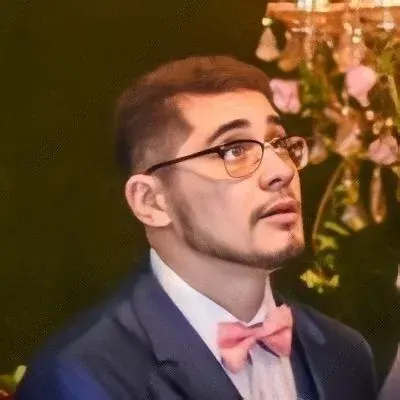
How to Execute a Function After setState
is Finished Updating in ReactJS
š Hey there, fellow ReactJS beginner! Don't worry, we've all been there. Understanding how setState
works can be a bit tricky at first, especially when it comes to executing functions after the state has finished updating. But fear not, because in this blog post, we're going to dive deep into this issue and provide you with easy solutions. So let's get started! šŖ
The Problem: Function Execution Before setState
Completes
In the provided code and context, you mentioned that when you change the number of rows or columns with the dropdown, the grid doesn't update correctly. It seems that the this.drawGrid()
function is executing before setState
is complete. This issue occurs because setState
is an asynchronous operation, meaning it doesn't immediately update the state. As a result, calling functions right after setState
might result in unexpected behavior.
Solution 1: setState
Callback Function
To ensure that a function executes only after setState
is complete, React provides us with a callback function as the second argument to setState
. This callback will be invoked right after the state has been updated. Let's modify your code to reflect this solution:
updateNumRows: function(event) {
this.setState({ rows: event.target.value }, this.drawGrid);
},
updateNumCols: function(event) {
this.setState({ cols: event.target.value }, this.drawGrid);
},
Now, whenever setState
finishes updating the state, it will automatically call the drawGrid
function for us. This will ensure that the grid is redrawn with the new row and column values.
Solution 2: componentDidUpdate
Lifecycle Method
Another way to tackle this issue is by utilizing the componentDidUpdate
lifecycle method. This method is invoked after each update to the component's state or props. By comparing the current and previous state values, we can determine whether the state has changed and then execute the desired function. Here's how we can implement this solution:
componentDidUpdate(prevProps, prevState) {
if (prevState.rows !== this.state.rows || prevState.cols !== this.state.cols) {
this.drawGrid();
}
}
With this solution, the drawGrid
function will only be called when the row or column values have changed, ensuring that it runs after the state has been updated.
Conclusion
š Congratulations on reaching the end! Now you know two different approaches to execute a function after setState
is finished updating in ReactJS. Feel free to experiment with both solutions and choose the one that best fits your needs.
If you have any more questions or need further assistance, don't hesitate to ask. We're here to help! Happy coding! š
Share Your Experience!
Have you ever encountered issues with setState
in ReactJS? How did you handle the situation? Share your thoughts, tips, or any funny stories in the comments section below. Let's learn from each other and keep the conversation going! š¬