Call child method from parent
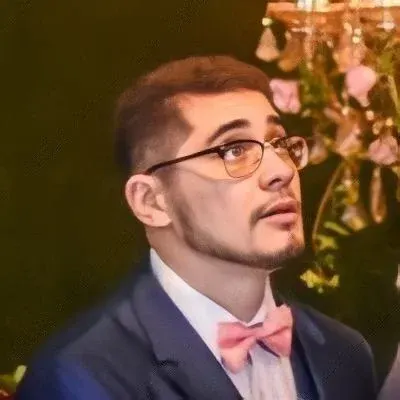
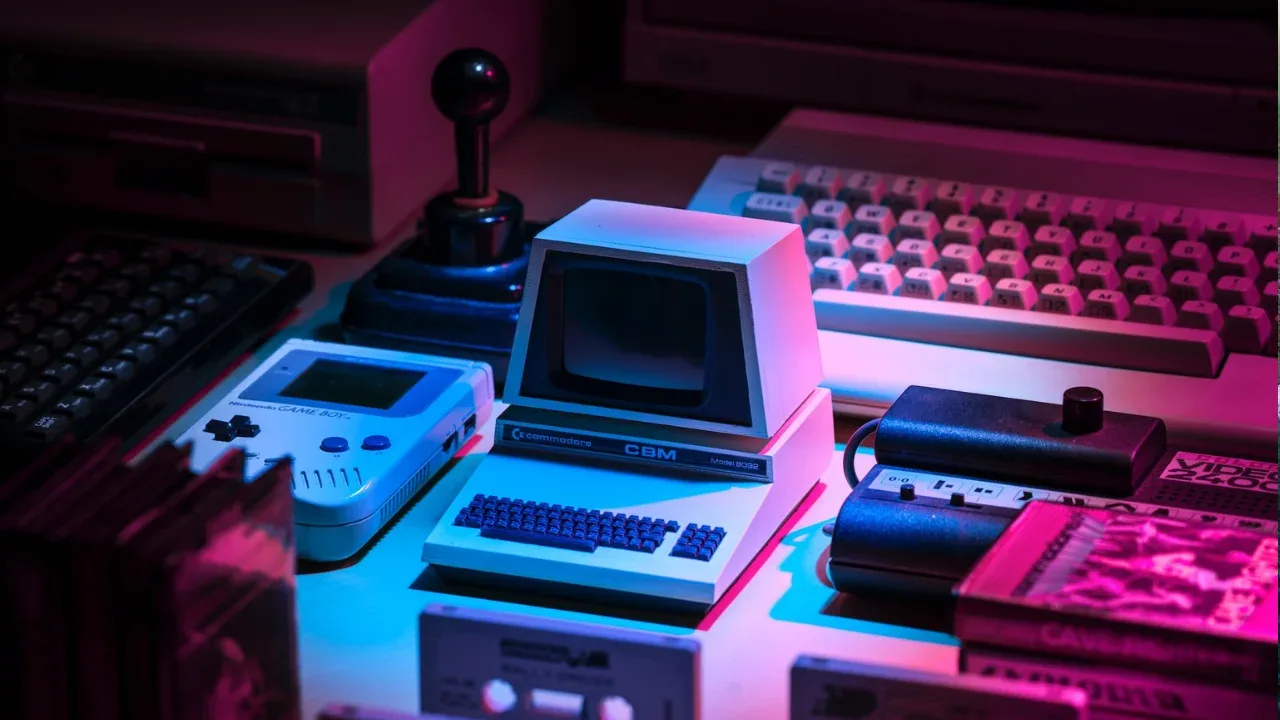
Calling a Child Method from Parent: The Ultimate Guide! 📞
As a developer, you may come across situations where you need to call a method defined in a child component from its parent component. This can be a bit tricky, but fear not! In this guide, we will explore common issues, provide easy solutions, and empower you to make those cross-component method calls with ease. Let's dive in! 💪
Understanding the Challenge
In the provided example, we have a Parent component and a Child component. The goal is to call the getAlert
method defined in the Child component when a button is clicked in the Parent component. However, the attempted solution did not yield the desired result.
Common Pitfalls and Solutions
Pitfall 1: Incorrect Syntax
The first pitfall in the provided code is the incorrect syntax used to call the getAlert
method in the Child component. Let's take a look at the problematic line:
<button onClick={Child.getAlert()}>Click</button>
In this line, Child.getAlert()
is immediately invoked, meaning it will execute the method during the rendering phase instead of when the button is clicked. To fix this, we need to pass a reference to the getAlert
method without invoking it immediately.
Solution:
To pass a reference to the getAlert
method, we can update the problematic line as follows:
<button onClick={() => this.childRef.getAlert()}>Click</button>
Here's what's happening:
We create an arrow function that, when executed, will call the
getAlert
method on the child component.We access the child component using a ref, which we'll set up in the Parent component.
Pitfall 2: Unavailable Child Component
Another challenge arises when trying to access the Child component from the Parent component. In the provided code, the Child and Parent components are in separate files, but they still need a way to communicate.
Solution:
To establish communication between the Parent and Child components, we can make use of refs.
In the Parent component, declare a ref and attach it to the Child component:
<Child ref={(ref) => (this.childRef = ref)} />
Here, we create a ref called childRef
and assign it to the Child component using the ref
attribute.
In the Child component, utilize the ref to access the
getAlert
method:
getAlert() {
alert('clicked');
}
With the ref established, we can now call the getAlert
method on the Child component from the Parent component.
Bringing it All Together
After applying the solutions to the aforementioned pitfalls, our updated code should look like this:
class Parent extends Component {
render() {
return (
<Child ref={(ref) => (this.childRef = ref)} />
<button onClick={() => this.childRef.getAlert()}>Click</button>
);
}
}
class Child extends Component {
getAlert() {
alert('clicked');
}
render() {
return (
<h1 ref="hello">Hello</h1>
);
}
}
Time to Rock 'n' Roll! 🎸
With the correct syntax and the establishment of communication between the Parent and Child components, you can now confidently call the getAlert
method residing within the Child component by clicking the button in the Parent component. Congratulations, you've unlocked the power of cross-component method calls! 🎉
Share Your Success! 🎉
We hope this guide helped you overcome the challenges of calling a child method from a parent component. If you found this article useful, don't hesitate to share it with fellow developers. Also, feel free to leave any questions or comments below. We'd love to hear about your experiences and provide support. Happy coding! 💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
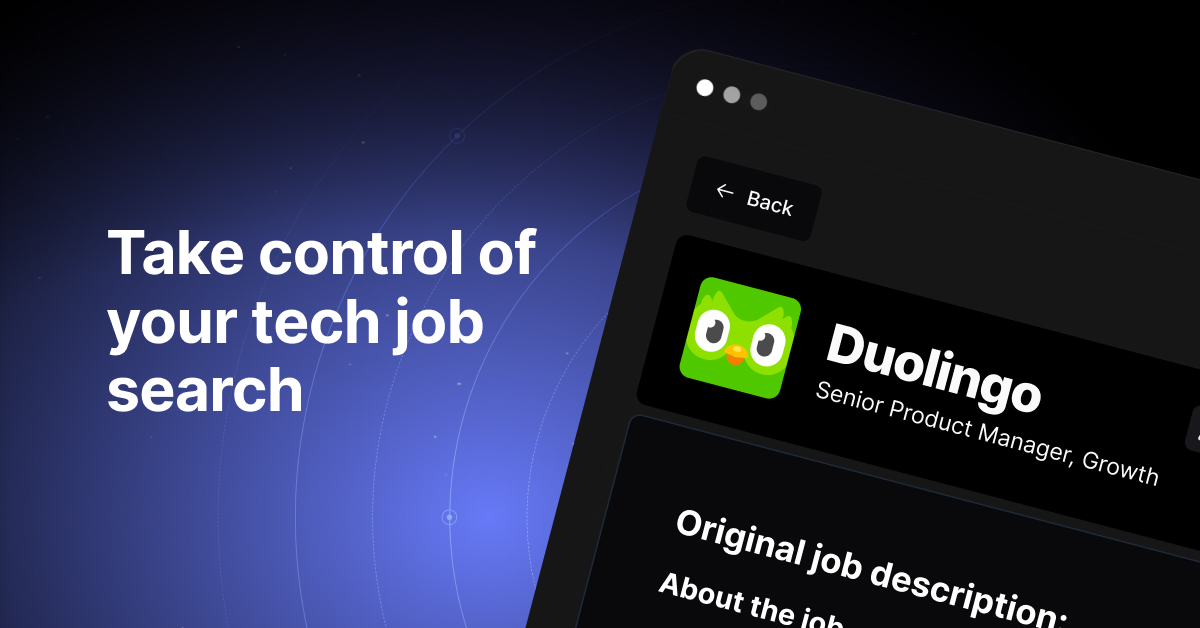