Call async/await functions in parallel
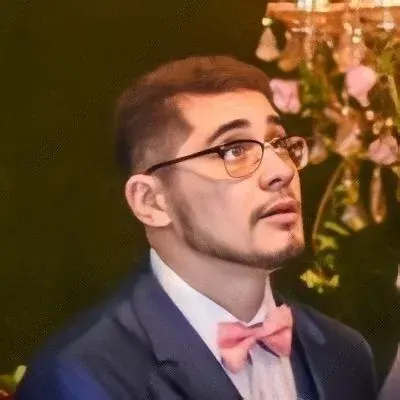
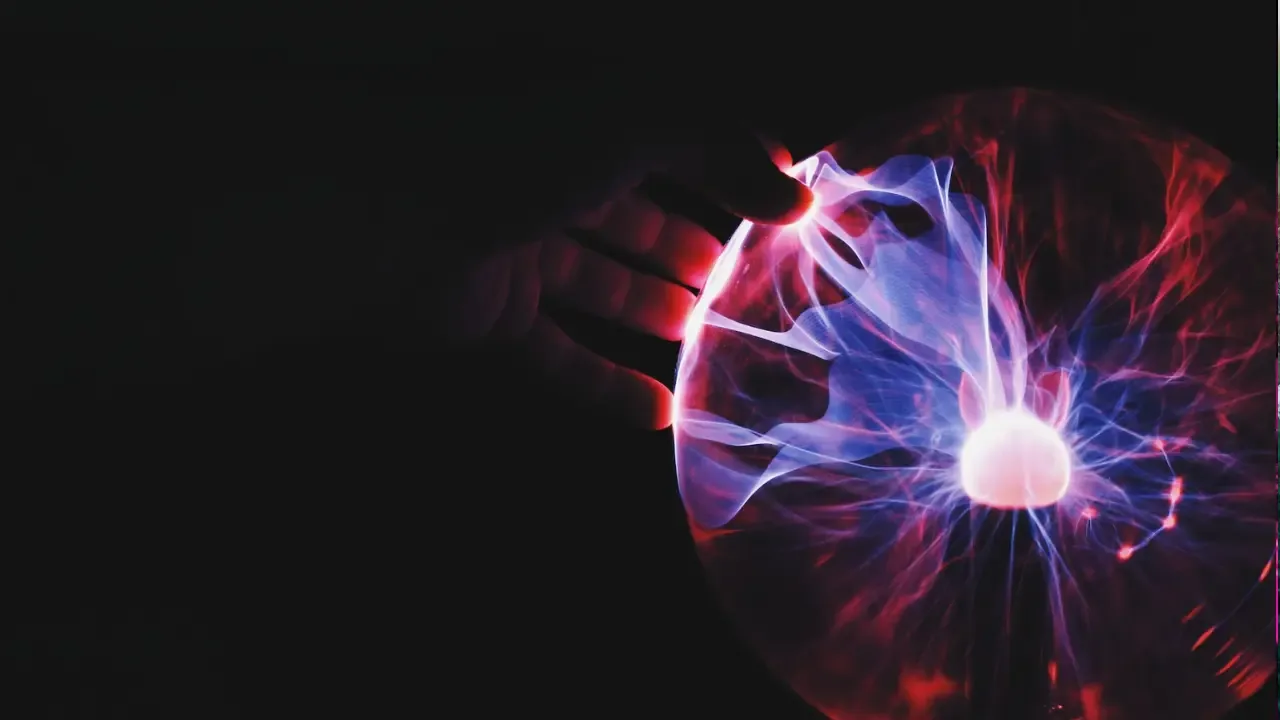
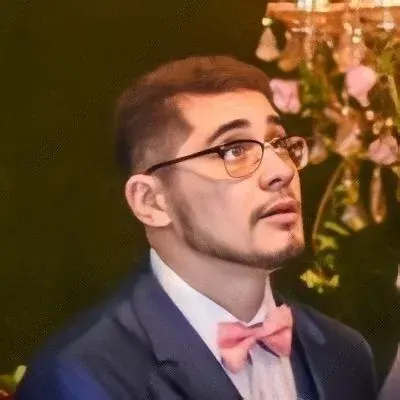
Calling async/await Functions in Parallel: A Complete Guide
š¤ Have you ever wondered how to call async/await functions in parallel instead of one after the other? If so, you're in the right place! In this guide, we'll explore common issues, provide easy solutions, and offer a compelling call-to-action to encourage your engagement.
Understanding the Issue
The code snippet you provided demonstrates sequential execution of async/await functions. The second function, anotherCall()
, is called only when the first function, someCall()
, is completed. This behavior is similar to chaining promises with .then()
, which ensures one function finishes before the next one starts.
Calling Functions in Parallel
To achieve parallel execution, we need to find an alternative approach. Fortunately, the JavaScript ecosystem offers several solutions. One popular option is to use the Promise.all()
method.
await Promise.all([someCall(), anotherCall()]);
By passing an array of promises to Promise.all()
, each promise will execute simultaneously. The await
keyword pauses execution until all promises within the array are resolved or rejected.
Leveraging the Power of the Async Library
If you're working with Node.js and prefer a solution involving the async library, let's explore an example.
First, make sure to install the async library by running the following command:
npm install async
Now, let's see how to call functions in parallel using the async.parallel()
method:
const async = require('async');
async.parallel([someCall, anotherCall], () => {
// Code to run after both functions have completed
});
In this example, the async.parallel()
method takes an array of functions as the first parameter. Each function represents the async/await call you want to execute in parallel. The second parameter is a callback function that will be invoked once both functions have completed.
š” Pro Tip: Handling Errors
When calling async/await functions in parallel, it's crucial to handle potential errors properly. Fortunately, both Promise.all()
and the async.parallel()
method handle errors in a convenient way.
With
Promise.all()
, if any of the promises within the array rejects, the overall promise will be rejected. In this case, you can use a try/catch block to handle the error gracefully.When using the async library, the callback function passed as the second parameter to
async.parallel()
will be called with an error as the first argument if any of the functions encounter an error. Ensure you have appropriate error-handling logic in place.
Your Engagement Matters! š£
Now that you have a clear understanding of how to call async/await functions in parallel, it's time to put your knowledge into practice. Experiment with the provided solutions, share your experience in the comments section, and let's make parallel execution a breeze!
Have you encountered any other challenges related to async/await functions? Share your questions and let's dive deeper together.
Remember, sharing is caring! If you found this guide helpful, remember to share it with your fellow developers and spread the knowledge!
Happy coding! šš