babel-loader jsx SyntaxError: Unexpected token
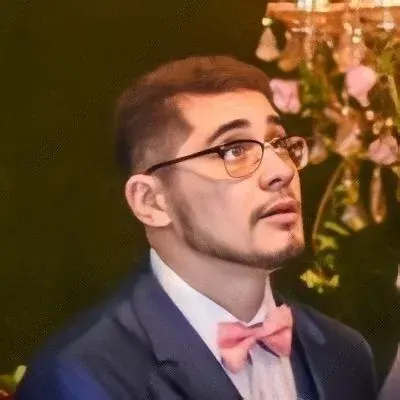
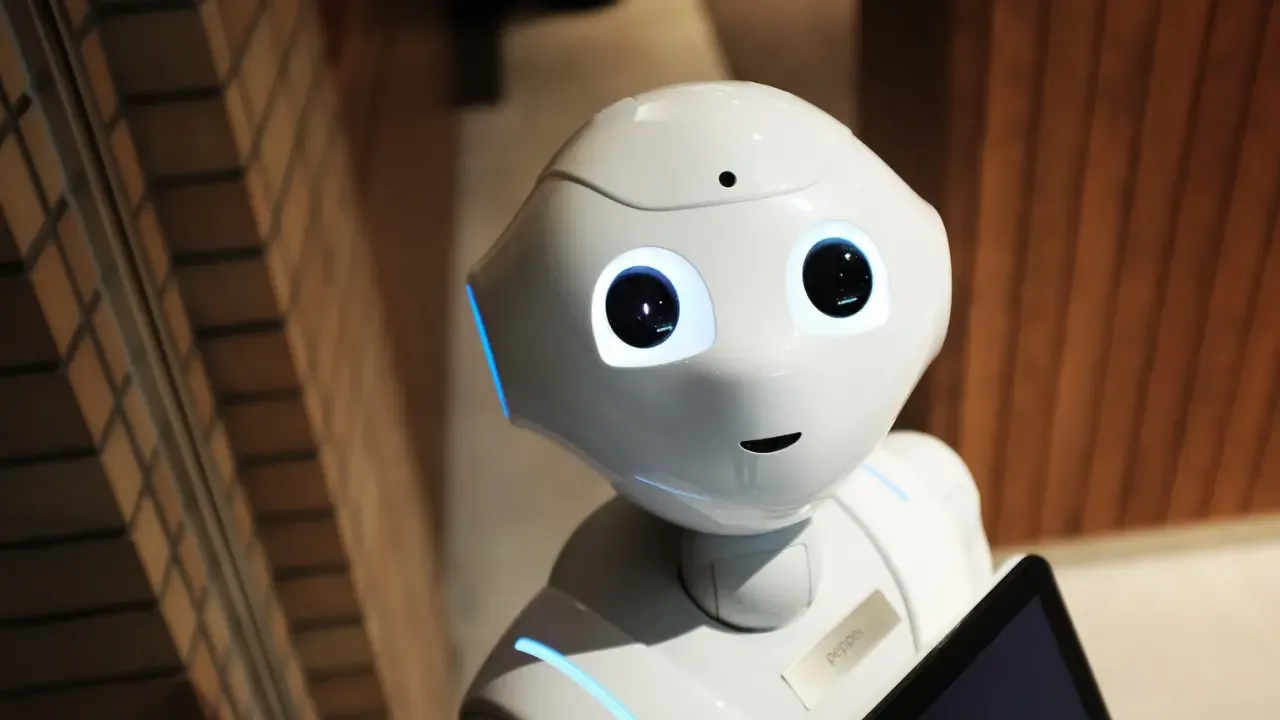
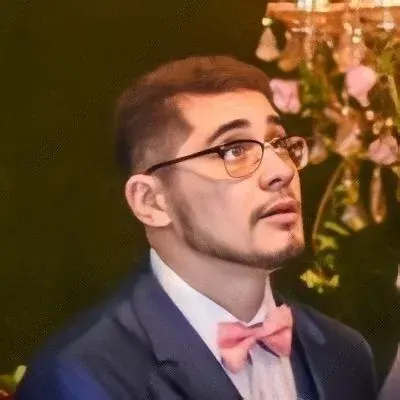
📝 Fixing the Babel-Loader JSX SyntaxError: Unexpected Token
Are you a beginner in React + Webpack and encountering a weird error in your hello world web app? Don't worry, I've got you covered! 🤓
The error you're facing is related to the babel-loader not being able to understand the JSX syntax. JSX is a syntax extension for JavaScript that's used with React to write HTML-like code within your JavaScript files.
Here's a step-by-step guide on how to fix the "Unexpected token" error you encountered:
Step 1: Check your dependencies
Make sure you have the necessary dependencies installed in your project. In your case, you should have the following devDependencies installed:
"babel-core": "^6.0.14",
"babel-loader": "^6.0.0",
"webpack": "^1.12.2",
"webpack-dev-server": "^1.12.1"
And the following dependency:
"react": "^0.14.1"
If any of the dependencies are missing or have incompatible versions, you can encounter issues with JSX syntax.
Step 2: Configure your webpack.config.js
In your webpack.config.js
file, you need to make sure that the babel-loader
is configured correctly to handle JSX files. The module
section of your webpack configuration is responsible for defining loaders. Add the following configuration to handle .js
files and convert JSX syntax:
module: {
loaders: [
{
test: /\.js$/,
exclude: /node_modules/,
loader: "babel-loader"
}
]
}
This configuration tells webpack to use the babel-loader
for all JavaScript files except those in the node_modules
directory.
Step 3: Update your app/main.js file
Now, let's fix the issue in your app/main.js
file. The React.render
method is deprecated in newer versions of React. Instead, you should use the ReactDOM.render
method. Modify your app/main.js
file as follows:
import React from "react";
import ReactDOM from "react-dom";
ReactDOM.render(<h1>hello world</h1>, document.getElementById("app"));
By importing ReactDOM
and using the correct render
method, you'll resolve the error.
Step 4: Run webpack
After making these changes, save your files and run webpack again. This should successfully compile your code without any "Unexpected token" errors.
And voila! 🎉 You've fixed the babel-loader JSX SyntaxError: Unexpected Token issue.
If you still encounter any issues, please let me know in the comments section below. I'll be happy to help you further.
✍️ Don't forget to share this post with your fellow React + Webpack beginners to help them out as well. Happy coding! 💻🚀