Axios - DELETE Request With Request Body and Headers?
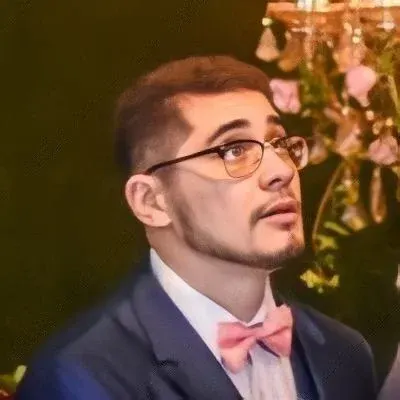
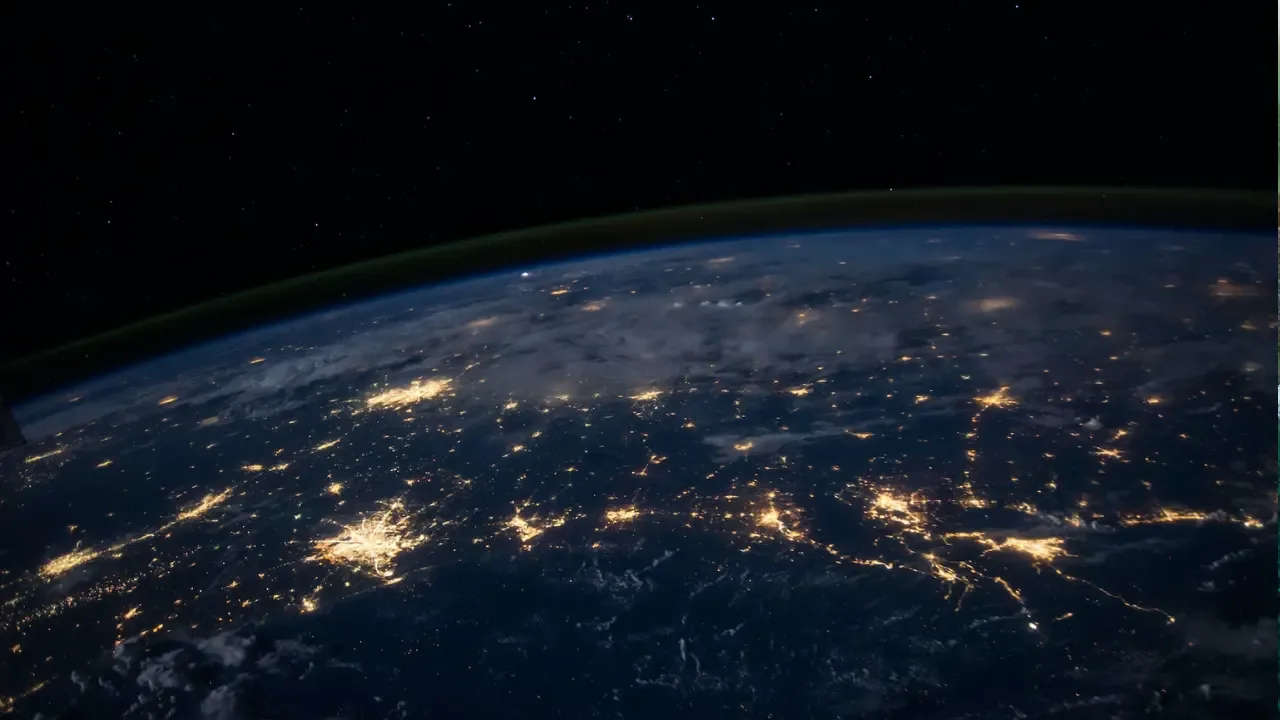
Axios - DELETE Request With Request Body and Headers?
š Hey there! Are you programming in ReactJS and trying to send a DELETE request to your server using Axios? š If you're struggling with adding headers and a request body to your DELETE request, you've come to the right place! š
Let's dive into the common issues and easy solutions for sending a DELETE request with headers and a body using Axios.
The Challenge
As you mentioned, Axios DELETE requests traditionally don't allow a request body, and the request parameters are passed differently compared to other HTTP methods. However, there are workarounds to achieve what you need!
The Solution
To send a DELETE request with headers and a body using Axios, we need to use a technique called "method tunneling". We'll convert our DELETE request into a POST request, add the necessary headers, and include the payload in the request body. Let's break it down step by step:
Convert DELETE to POST:
axios.post(URL, payload, { headers: header, _method: 'DELETE' });
Modify your server to recognize the _method parameter as a DELETE request:
app.use((req, res, next) => { if (req.body._method === 'DELETE') { req.method = 'DELETE'; req.url = req.path; } next(); });
With these changes, we're effectively tunneling our DELETE request through a POST request, while still maintaining the desired functionality.
Example
Let's put it all together with a concrete example:
const deleteUser = (userId) => {
const payload = { user: userId };
const headers = { Authorization: 'Bearer your_token' };
axios.post('/api/users', payload, { headers, _method: 'DELETE' })
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
};
In this example, we're using axios.post
instead of axios.delete
, passing the payload
, headers
, and _method: 'DELETE'
as options.
Call to Action
Now that you have a solution for sending a DELETE request with headers and a body using Axios, it's time to give it a try! š Update your code, test it out, and see if it works for you.
If you found this guide helpful or have any questions, let us know in the comments below! We'd love to hear your thoughts and provide further assistance if needed.
Happy coding! š»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
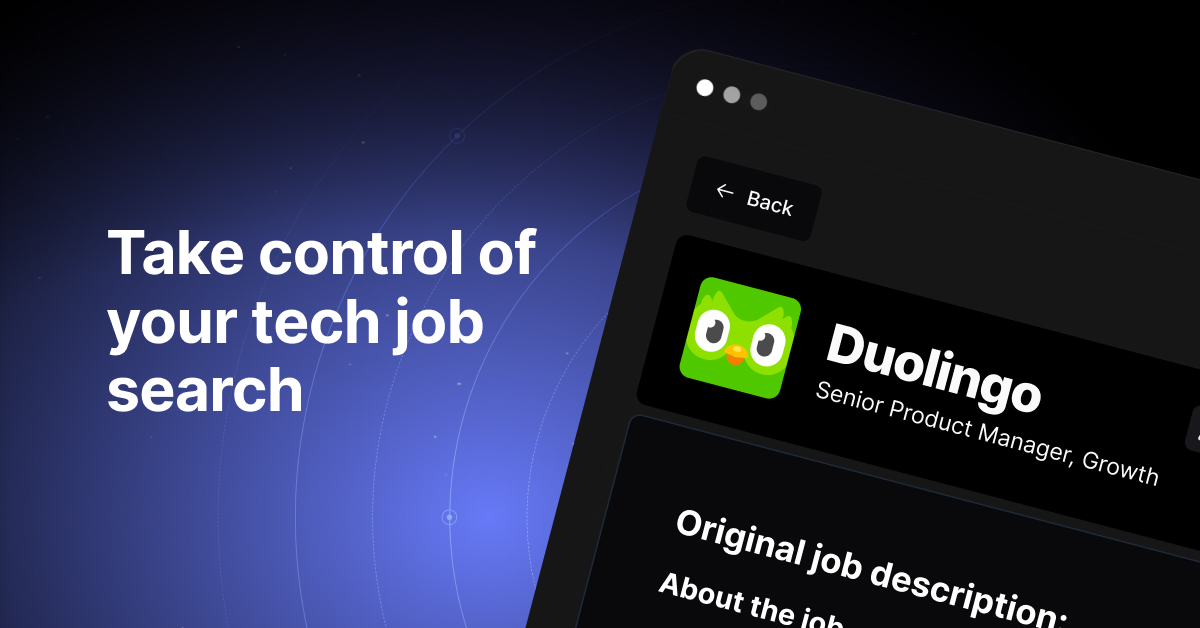