ASP.NET MVC JsonResult Date Format
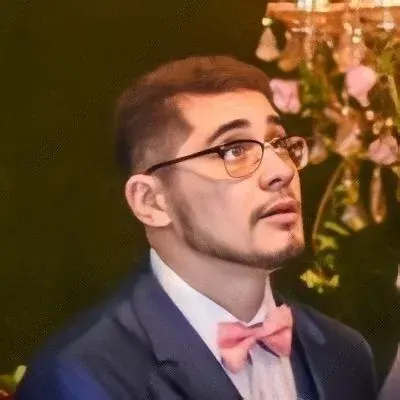
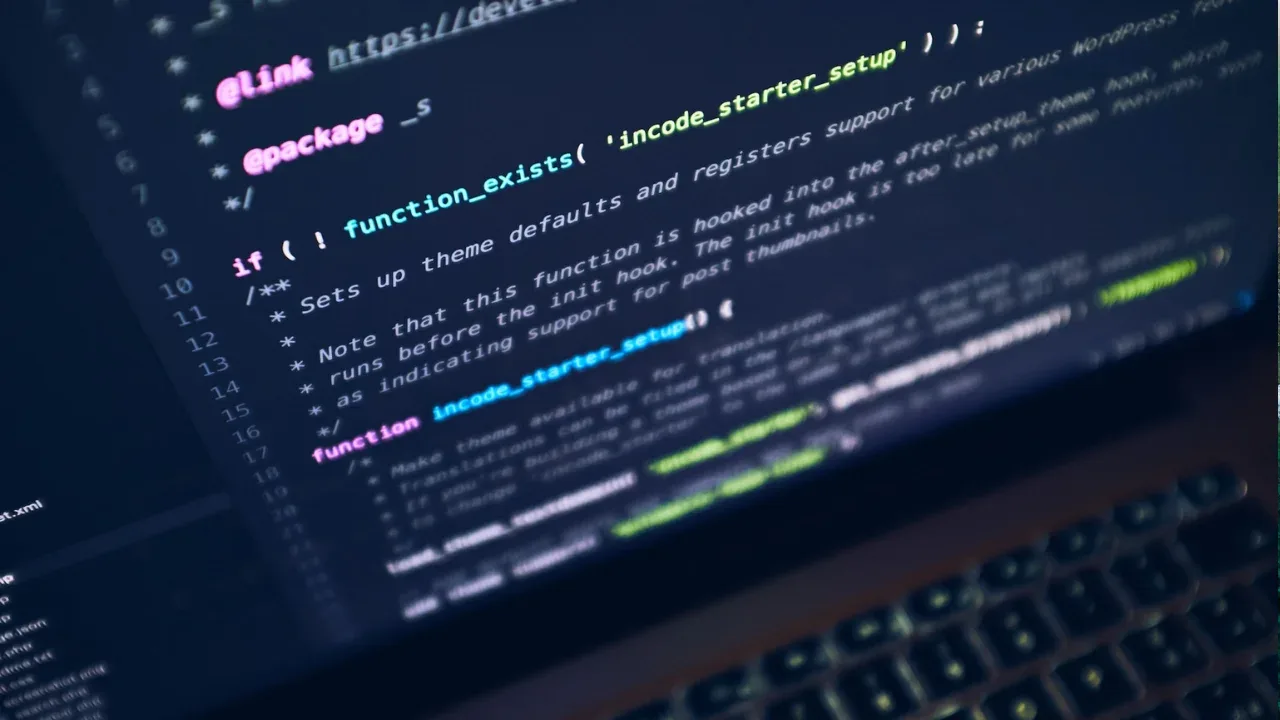
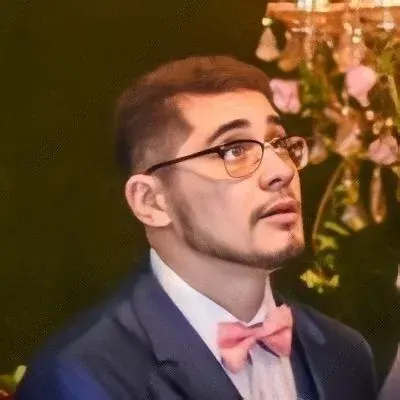
๐ Solving Date Format Issue with ASP.NET MVC JsonResult ๐
Are you facing an issue with the date format while using the JsonResult
in your ASP.NET MVC application? Don't worry, we've got you covered! ๐คฉ
๐ The Problem
You have a JsonResult
in your controller action that returns your model. However, when you receive the JSON result, you notice that the date property is in a strange format like "\/Date(1239018869048)\/"
. This format might not be useful for your specific requirements or may be difficult to handle in your client-side script. So, how can you deal with this issue? Let's find out! ๐
๐ ๏ธ Solution 1: Custom Date Formatting in ASP.NET MVC Controller
One way to solve this issue is by providing a custom date format in your ASP.NET MVC controller action. You can do this using the JsonRequestBehavior
enumeration and the JsonSerializerSettings
class. Let's have a look at the code:
public ActionResult MyAction()
{
MyModel myModel = // Retrieve your model here
JsonSerializerSettings jsonSettings = new JsonSerializerSettings
{
DateFormatString = "yyyy-MM-dd" // Customize the date format as per your requirements
};
return Json(myModel, JsonRequestBehavior.AllowGet, jsonSettings);
}
In the above code, we create an instance of JsonSerializerSettings
and set the DateFormatString
property to the desired format, such as "yyyy-MM-dd"
. This way, the date in your model will be serialized using the specified format in the JSON result.
๐งช Example
Let's say you have a DateTime
property named BirthDate
in your MyModel
class. When you use the custom date format as mentioned above, the JSON result will look like this:
{
"BirthDate": "1990-05-10"
}
Now, you can easily handle this formatted date in your client-side script without any hassle. ๐
๐ Solution 2: Formatting Dates in JavaScript
If you prefer to format the date on the client-side using JavaScript, we have a solution for you too! There are several libraries available, like Moment.js, Luxon, or the built-in JavaScript Date
object. Let's see an example using the Date
object:
var dateValue = new Date(1239018869048);
var formattedDate = dateValue.toISOString();
console.log(formattedDate); // Output: "2009-04-06T19:01:09.048Z"
As shown in the above code snippet, we create a new Date
object using the timestamp provided in the JSON result. Then, we can use the toISOString
method to get the date in a standard ISO format. Feel free to format the date as per your requirements using various methods or libraries.
๐ฌ Join the Conversation!
We hope this guide helped you solve the date format issue with JsonResult
in your ASP.NET MVC application. Now, it's your turn! Share your experience or thoughts in the comments below. Have you faced any similar issues? How did you solve them? Let's discuss and help each other out! ๐
Remember, stay updated with our blog for more exciting tech tips and tricks. Happy coding! ๐