Array.push() if does not exist?
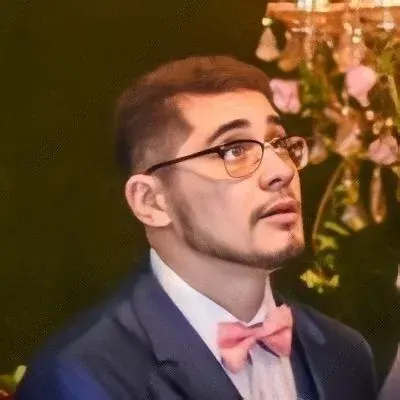
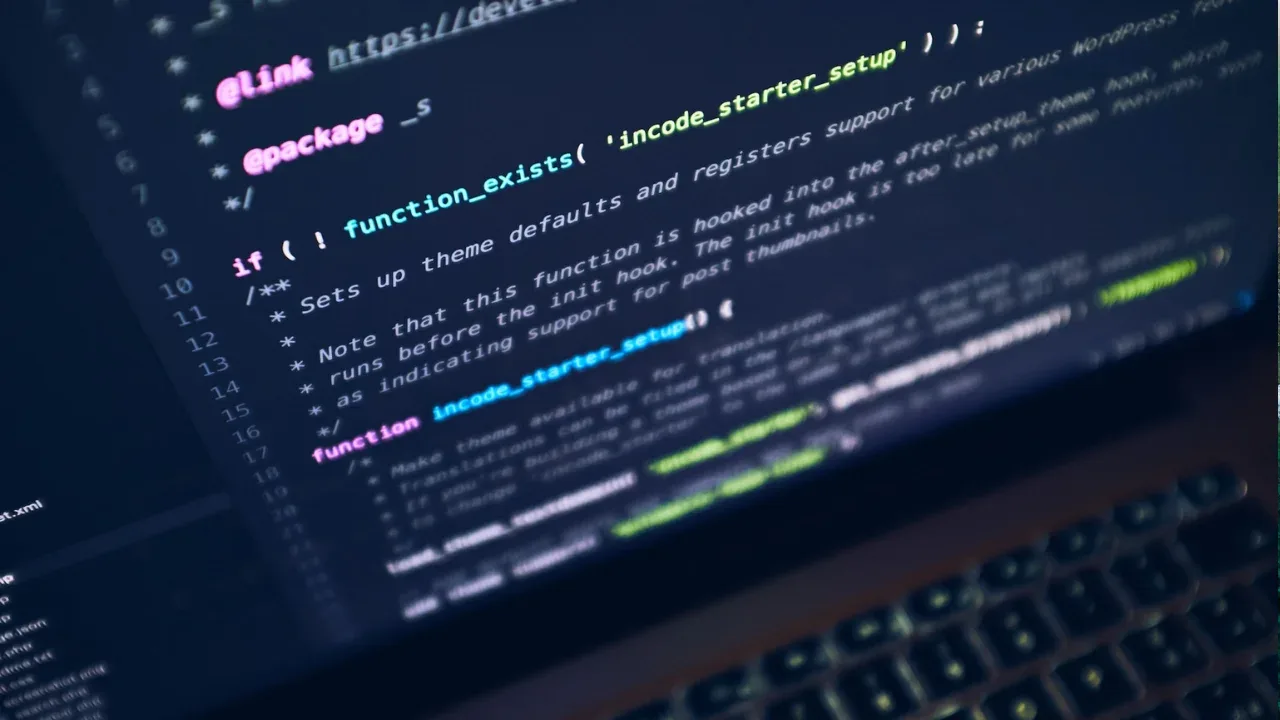
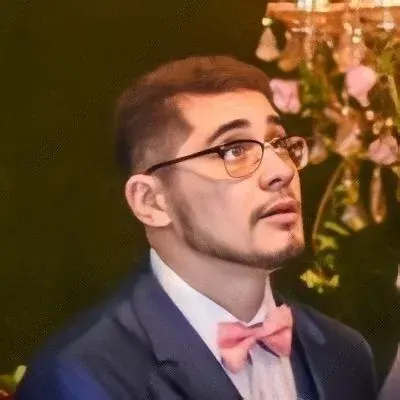
🚀 Push into an Array only if Values do not Exist!
So you want to push new objects into an array, but only if the values of those objects do not already exist? No worries, we've got you covered! In this guide, we'll show you an easy and straightforward solution to solve this problem. Let's dive right in! 💪
First off, let's take a look at the context of the problem. You have an array containing objects with the properties "name" and "text". You want to push new objects into this array, but only if neither of the existing objects in the array has the same values for "name" and "text". Sounds simple, right? Let's see how we can achieve this using JavaScript. 🤔
Solution: The Array.push() Method with a Twist!
To accomplish this task, we can utilize the powerful Array.push()
method with an added condition to check if the values already exist in the array. Here's the code snippet that does the trick:
const myArray = [
{ name: "tom", text: "tasty" },
{ name: "tom", text: "tasty" },
{ name: "tom", text: "tasty" },
{ name: "tom", text: "tasty" },
{ name: "tom", text: "tasty" }
];
const newObj = { name: "jerry", text: "delicious" };
const result = myArray.some(obj => obj.name === newObj.name && obj.text === newObj.text);
if (!result) {
myArray.push(newObj);
}
console.log(myArray);
Let's break it down step by step:
We declare the original array,
myArray
, that contains the existing objects.We define the new object we want to push into the array,
newObj
, with your desired values for "name" and "text".Next, we use the
Array.some()
method to check if there is at least one object inmyArray
that has the same values for "name" and "text" asnewObj
.If the result of the condition is
false
, indicating that there are no matching objects in the array, we pushnewObj
intomyArray
usingArray.push()
.Finally, we log the contents of
myArray
to the console to see the updated array.
Putting it into Action!
Let's run this code and see the output:
[
{ name: "tom", text: "tasty" },
{ name: "tom", text: "tasty" },
{ name: "tom", text: "tasty" },
{ name: "tom", text: "tasty" },
{ name: "tom", text: "tasty" },
{ name: "jerry", text: "delicious" }
]
As you can see, the new object { name: "jerry", text: "delicious" }
was successfully pushed into myArray
, as none of the existing objects had the same values for "name" and "text" as the new object. 🎉
Action Time: Share Your Thoughts!
Now that you've learned a handy solution to push new objects into an array only if the values don't already exist, it's your turn to give it a try! 👩💻
We would love to hear your thoughts on this approach. Have you faced similar challenges before? How would you solve this problem differently? Share your experiences, ideas, and questions in the comments below! Let's grow together as tech enthusiasts! 🌟