appending array to FormData and send via AJAX
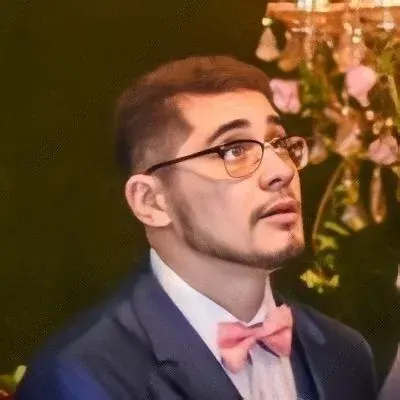
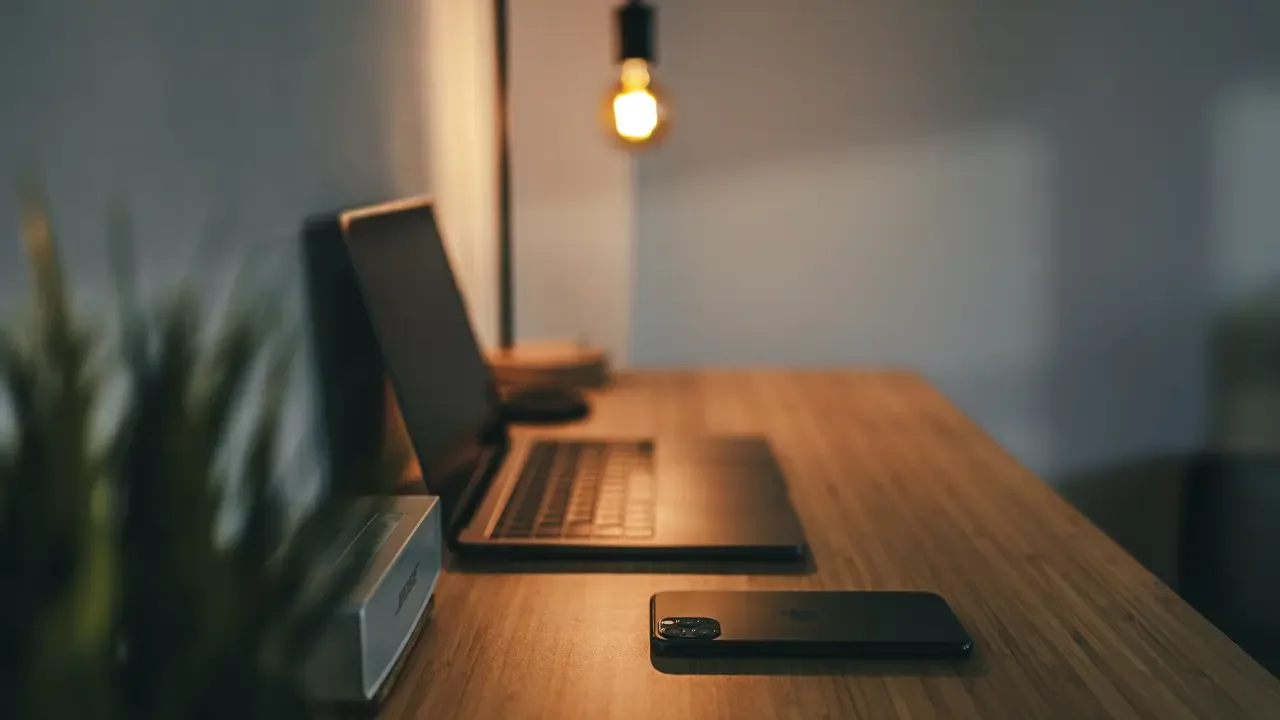
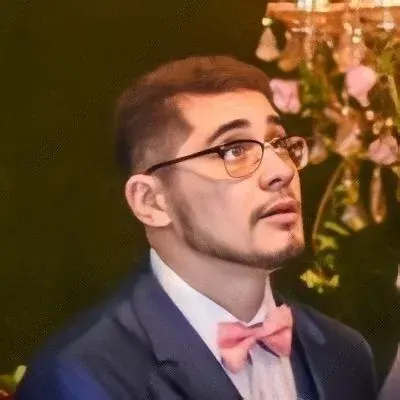
πTech Tips: How to Append an Array to FormData and Send via AJAX π
Are you struggling with appending an array to FormData and sending it via AJAX? π€ Don't worry, you're not alone! Many developers face this challenge when trying to submit multipart forms with arrays, text fields, and files seamlessly. π
In this blog post, we'll address this common issue and provide easy solutions to help you achieve your desired result. π‘ So, let's dive in and find out how to solve this problem! πͺ
The Context π
Let's start by understanding the context in which this problem arises. Below is an example of how the data is being appended to the FormData object and sent via AJAX:
var attachments = document.getElementById('files');
var data = new FormData();
for (var i = 0; i < attachments.files.length; i++) {
data.append('file', attachments.files[i]);
console.log(attachments.files[i]);
data.append('headline', headline);
data.append('article', article);
data.append('arr', arr);
data.append('tag', tag);
}
$.ajax({
type: "post",
url: 'php/submittionform.php',
cache: false,
processData: false,
contentType: false,
data: data,
success: function(request) {
$('#box').html(request);
}
});
The Issue π«
The problem occurs on the PHP side when the arr
variable, which is initially an array, appears as a string. This happens when the data is sent via AJAX using FormData. π€―
Interestingly, if you send the data using the simple $.POST
option instead of FormData, the arr
variable arrives correctly as an array on the PHP side. However, this approach doesn't allow you to send files simultaneously. π’
The Solutions π
Solution 1: JSON.stringify
One easy solution is to JSON.stringify the array before appending it to FormData:
data.append('arr', JSON.stringify(arr));
And on the PHP side, you can use json_decode to convert the received string back to an array:
$arr = json_decode($_POST['arr'], true);
Solution 2: Converting the Array to Multiple FormData Instances
Another workaround is to convert the array into multiple instances of FormData. You can append each element of the array individually to a separate FormData instance, and then append all the instances to another FormData that will be sent via AJAX. The PHP side will handle it as an array accordingly.
for (var i = 0; i < arr.length; i++) {
var tempData = new FormData();
tempData.append('arr', arr[i]);
data.append('arr[]', tempData.get('arr'));
}
On the PHP side, you can retrieve the array as follows:
$arr = $_POST['arr'];
The Call-to-Action π£
Now that you have learned how to overcome the hurdles of appending an array to FormData and sending it via AJAX, it's time to put this knowledge into action! β¨
Try implementing the provided solutions and see which one works best for your specific use case. Don't forget to share your experiences and tips with the community! π¬
If you have any other questions or need further assistance, feel free to leave a comment below or reach out to us. We're here to help! π€
Happy coding! π»π
Do you have any other tech-related questions or challenges? Let us know in the comments! π