AngularJS toggle class using ng-class
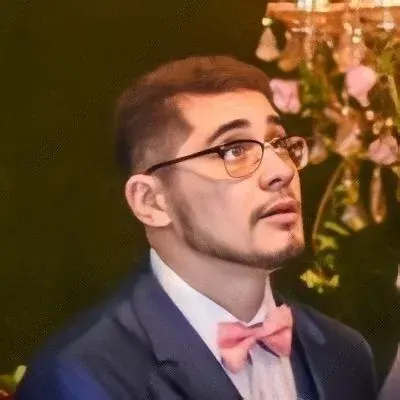
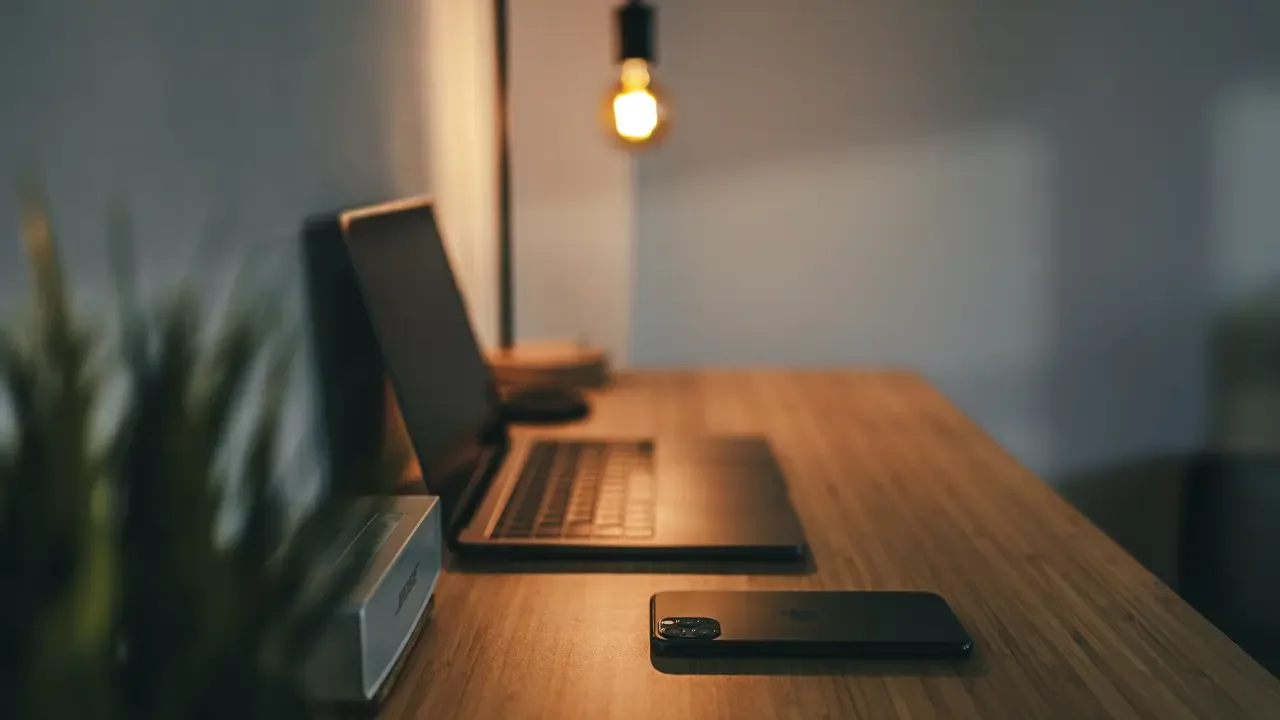
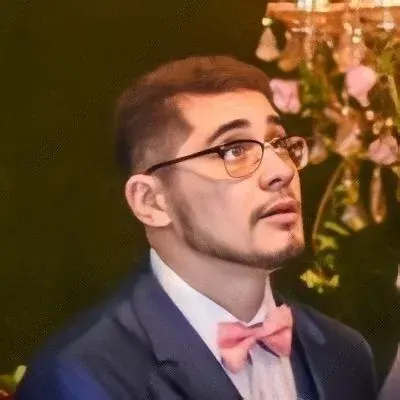
AngularJS Toggle Class Using ng-class
š š”
Are you trying to toggle the class of an element using ng-class in AngularJS? It can be a bit tricky, but don't worry, we've got you covered! In this blog post, we will address the common issues and provide easy solutions to help you achieve the desired result. šÆ
The syntax you provided seems to be correct at first glance. However, it seems like you're encountering an error in the console. Let's take a closer look at the issue and find a solution together. šŖ
The error you're seeing in the console is:
Error: Lexer Error: Unexpected next character at columns 18-18 [?] in expression [{(isAutoScroll()) ? 'icon-autoscroll' : 'icon-autoscroll-disabled'}].
This error usually occurs when there's a syntax error or an unexpected character in the expression. To fix this, let's try some alternative solutions. š
Solution 1: Using ng-click
One approach to toggle the class is by utilizing the ng-click directive. We can bind a click event to the button and update the value of $scope.autoScroll
. Here's an example:
<button class="btn" ng-click="toggleScroll()">
<i ng-class="{'icon-autoscroll': autoScroll, 'icon-autoscroll-disabled': !autoScroll}"></i>
</button>
In your controller, you can define the toggleScroll
function like this:
$scope.toggleScroll = function() {
$scope.autoScroll = !$scope.autoScroll;
}
In this solution, when the button is clicked, the toggleScroll
function will be called, flipping the value of $scope.autoScroll
. Depending on the value, the appropriate class will be applied using ng-class
.
Solution 2: Using Ternary Operator
Another way to toggle the class is by using a ternary operator directly in the ng-class expression. Here's an example:
<button class="btn" ng-click="autoScroll = !autoScroll">
<i ng-class="autoScroll ? 'icon-autoscroll' : 'icon-autoscroll-disabled'"></i>
</button>
In this solution, we directly use the ternary operator within the ng-class expression itself. If autoScroll
is true
, the class icon-autoscroll
will be applied. Otherwise, the class icon-autoscroll-disabled
will be applied.
Solution 3: The Most Preferred Approach
As of now, the most standard and preferred approach is Solution 3, provided by Stewie. It is the easiest to read and understand. Here's the updated solution:
<button class="btn" ng-click="autoScroll = !autoScroll">
<i class="icon" ng-class="autoScroll ? 'icon-autoscroll' : 'icon-autoscroll-disabled'"></i>
</button>
This solution is similar to Solution 2 but utilizes a single class icon
for the <i>
element. Based on the value of autoScroll
, it will have either the icon-autoscroll
or icon-autoscroll-disabled
class.
We hope one of these solutions helps you resolve the issue and toggle the class successfully using ng-class in AngularJS! Let us know if you have any further questions or need additional assistance. š
Now it's your turn to give it a try! Choose the solution that best fits your requirements and start toggling those classes like a pro. Don't hesitate to share your success in the comments below. Happy coding! š