AngularJS ng-click stopPropagation
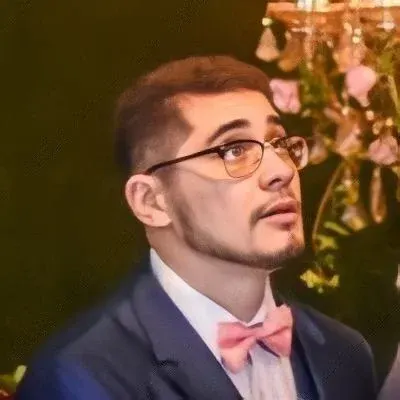

AngularJS ng-click stopPropagation
: A Guide to Prevent Event Propagation in AngularJS
Have you ever encountered a situation in your AngularJS application where clicking a button inside a table row triggers the click event on the row itself? 🤔 Frustrating, right? But don't worry, we've got you covered! In this guide, we'll explore how to prevent event propagation using the ng-click stopPropagation
directive.
Understanding the Problem
Let's take a closer look at the code snippet you provided:
<tbody>
<tr ng-repeat="user in users" class="repeat-animation" ng-click="showUser(user, $index)">
<td>{{user.firstname}}</td>
<td>{{user.lastname}}</td>
<td>{{user.email}}</td>
<td><button class="btn red btn-sm" ng-click="deleteUser(user.id, $index)">Delete</button></td>
</tr>
</tbody>
As you mentioned, when you click the delete button, the click event on the table row (ng-click="showUser(user, $index)"
) is also triggered. This is because events in JavaScript have a concept called event propagation.
Event propagation occurs in two phases: capturing and bubbling. During the capturing phase, the event travels from the outermost container down to the target element. In the bubbling phase, the event travels back up from the target element to the outermost container. By default, events in AngularJS propagate in both phases.
The Solution: ng-click stopPropagation
To prevent the click event from propagating to the table row when clicking the delete button, you can use the ng-click stopPropagation
directive. This directive stops the event from propagating further up the DOM tree.
To apply ng-click stopPropagation
, modify your code as follows:
<tbody>
<tr ng-repeat="user in users" class="repeat-animation">
<td>{{user.firstname}}</td>
<td>{{user.lastname}}</td>
<td>{{user.email}}</td>
<td>
<button class="btn red btn-sm" ng-click="deleteUser(user.id, $index); $event.stopPropagation()">Delete</button>
</td>
</tr>
</tbody>
In the modified code, we removed the ng-click
directive from the table row (ng-click="showUser(user, $index)"
) and placed it directly in the delete button's ng-click
directive. Additionally, we added $event.stopPropagation()
to the delete button's ng-click
directive. This instructs AngularJS to stop the event propagation when the delete button is clicked.
With these changes, the click event on the delete button will no longer trigger the click event on the table row, providing the desired behavior.
Takeaway
Preventing event propagation in AngularJS can be achieved through the ng-click stopPropagation
directive. By utilizing this directive and placing it in the appropriate event handler, you can control the flow of events in your application and avoid unwanted interactions.
Next time you encounter event propagation issues in your AngularJS application, remember to reach for ng-click stopPropagation
and tame those unruly events! 😎
If you found this guide helpful, be sure to share it with your fellow AngularJS developers! Let us know in the comments below if you have any questions or if there are other AngularJS topics you'd like us to cover.
Happy coding! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
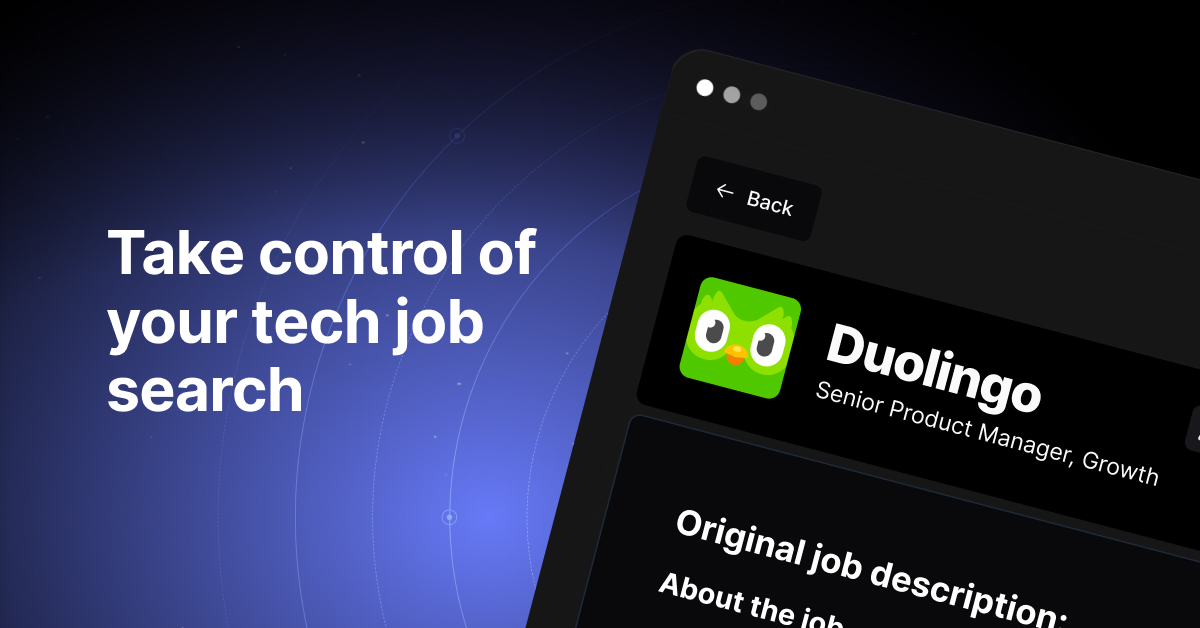