AngularJS $http and $resource
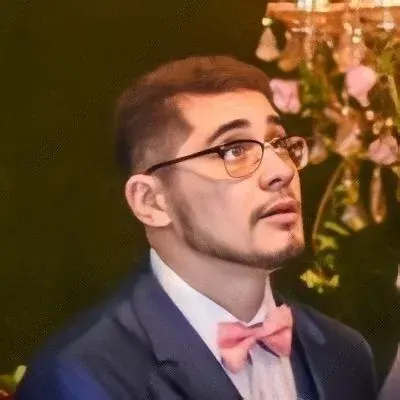
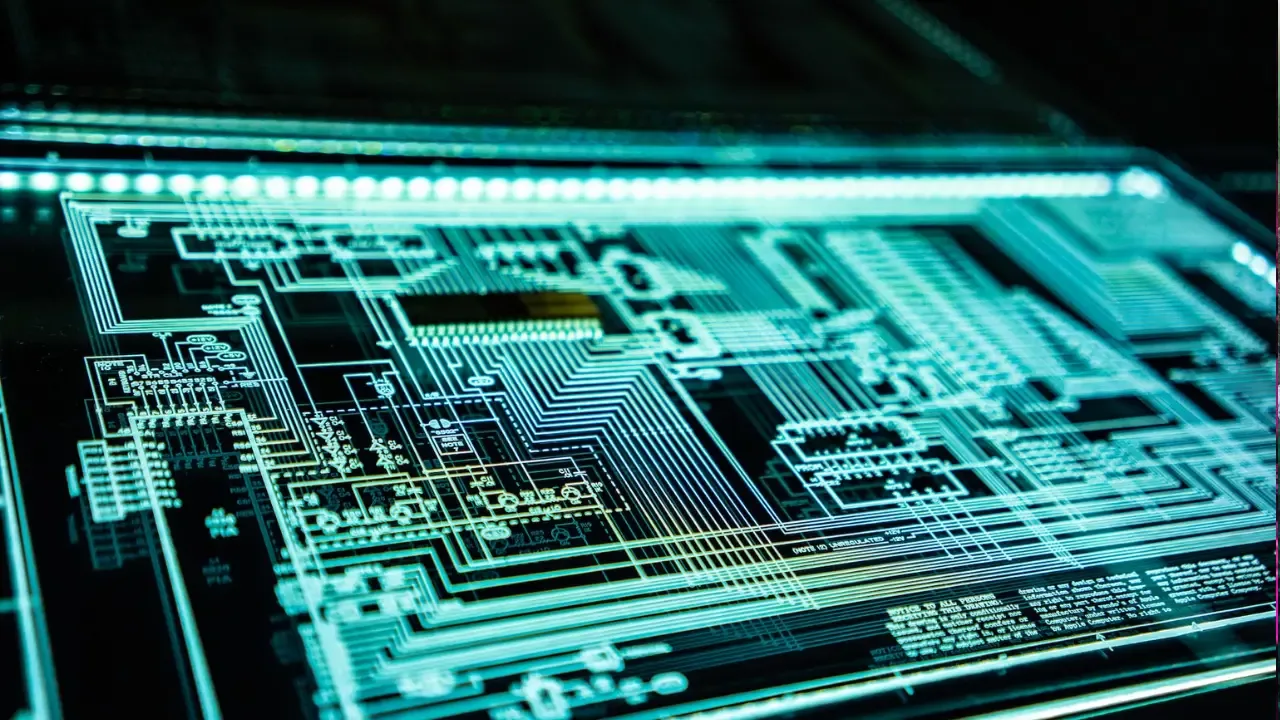
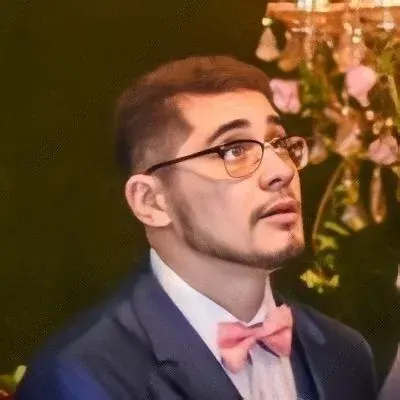
AngularJS $http and $resource: Demystified! 😎💪🚀
So, you're faced with a dilemma: Should you use $resource
or $http
in your AngularJS project? 🤔 Don't worry, my friend! I'm here to break it down for you in plain English, and show you when and how to use each one. Let's dive in! 💥
The Difference between $http and $resource
$http: This is the core AngularJS service for making HTTP requests. It provides a simpler and lower-level interface compared to
$resource
. You can think of it as a reliable workhorse that covers all your basic needs when interacting with RESTful APIs.$resource: This is a higher-level service built on top of
$http
. It provides a more powerful and convenient way to interact with RESTful APIs. It allows you to define resource objects that map to server-side resources, making it easier to perform CRUD operations (Create, Read, Update, Delete) on those resources.
When to Use $http
Let's start with $http
. Use $http
when:
You need to perform simple HTTP requests without any extra functionality.
You want fine-grained control over request and response handling.
You prefer a lighter and more flexible approach, without the added abstraction of resource objects.
Here's an example of how to use $http
to perform a GET request:
$http.get('/api/users')
.then(function(response) {
// Handle the successful response here
})
.catch(function(error) {
// Handle errors here
});
When to Use $resource
Now, let's talk about $resource
. Choose $resource
when:
You're working with a RESTful API and want to leverage its resource-based structure.
You want a higher-level interface that simplifies common CRUD operations.
You appreciate the automatic transformation of JSON responses into JavaScript objects.
Here's an example of how to use $resource
to interact with a user resource:
// Define the User resource
var User = $resource('/api/users/:id');
// Fetch a single user
var user = User.get({ id: 123 }, function() {
// Access the user properties here
});
// Update a user
user.name = 'John Doe';
user.$save(function() {
// Handle the successful update here
});
// Delete a user
user.$delete(function() {
// Handle the successful deletion here
});
The Power of $resource
One of the biggest advantages of $resource
is how it handles responses. When you call a method like .get()
, it automatically transforms the JSON response into a JavaScript object. This makes it super easy to work with the data, as if it were a regular JavaScript object. How cool is that? 😎
In Conclusion
To sum it up:
Use
$http
for simple HTTP requests and fine-grained control.Use
$resource
for resource-based CRUD operations with automatic object transformation.
Remember, both $http
and $resource
are incredibly powerful tools in your AngularJS arsenal. It ultimately depends on your project requirements and personal preference. Choose wisely, my friend! 💪
Have any more questions or want to share your AngularJS experiences? Feel free to leave a comment below! Let's keep the conversation going! 📝💬
Happy coding! 🚀✨