AngularJS : How do I switch views from a controller function?
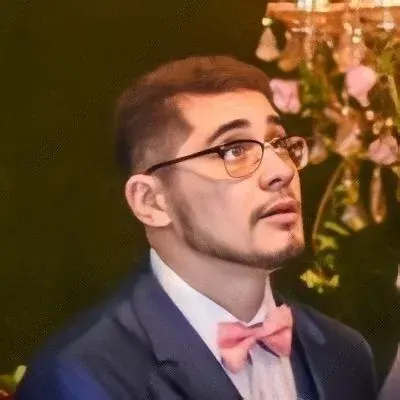
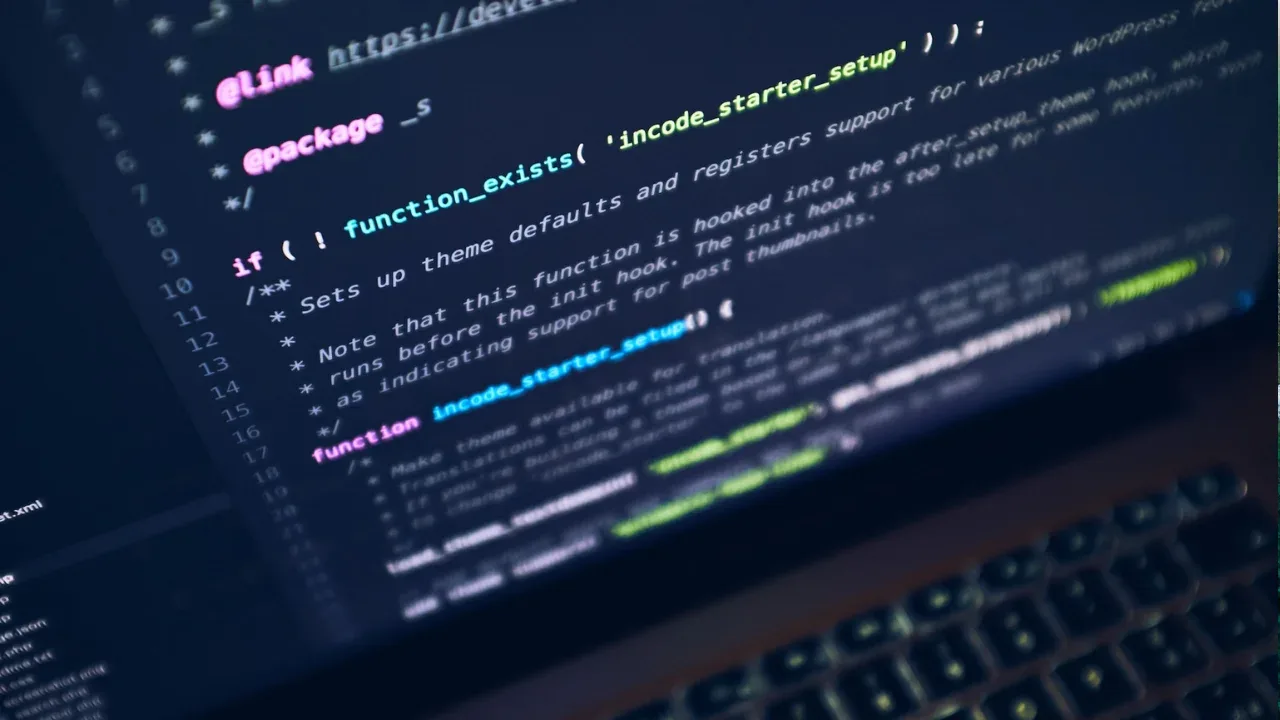
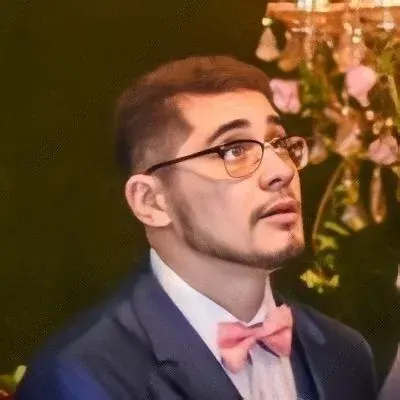
📢 Hey there, tech enthusiasts! Let's dive into the world of AngularJS and solve a common challenge: how to switch views from a controller function. 💡
So, you've got an AngularJS application with a controller and an HTML file. You want to switch views when a user clicks on a particular element. No worries, we've got your back! 🤗
In your index.html
file, you have a ng-click
attribute attached to a div
element, like this:
<div ng-controller="Cntrl">
<div ng-click="someFunction()">
click me
</div>
</div>
And there's your controller.js
file with the controller function:
function Cntrl($scope) {
$scope.someFunction = function(){
// code to change view?
}
}
Now, how do we change the view when someFunction()
is called? Here are a couple of simple ways to achieve that. Choose the one that suits your needs. 🤓
Option 1: Using $location
service
One way is to inject the $location
service into your controller and use its path()
method to change the URL. This will trigger AngularJS's routing mechanism and load the appropriate view.
Here's an example of how you can modify your controller function:
function Cntrl($scope, $location) {
$scope.someFunction = function(){
$location.path('/new-view');
}
}
In this example, when someFunction()
is called, the URL will change to http://yourapp/#!/new-view
. Make sure you have the necessary routing configuration set up in your application to handle this URL.
Option 2: Using $state
service
If you're using the UI Router module for your routing needs, you can also use the $state
service to switch views.
First, make sure you have the UI Router module included in your project. Then, inject the $state
service into your controller.
function Cntrl($scope, $state) {
$scope.someFunction = function(){
$state.go('newView');
}
}
In this example, when someFunction()
is called, the view with the newView
state will be loaded.
Choose the approach that best suits your application's routing configuration and needs. 💡
Now that you have the tools to switch views from your controller function, go ahead and create a seamless user experience for your users! 🚀
If you have any doubts or face any challenges, leave a comment below and we'll be more than happy to assist you.
So, what are you waiting for? Start implementing these solutions and rock your AngularJS app! 💪
Remember, sharing is caring! 👍 Share this blog post with your fellow developers and help them level up their AngularJS game.