AngularJS : Factory and Service?
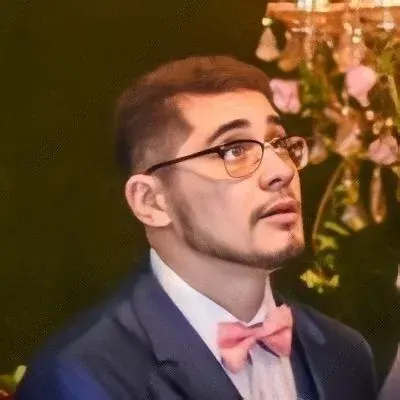
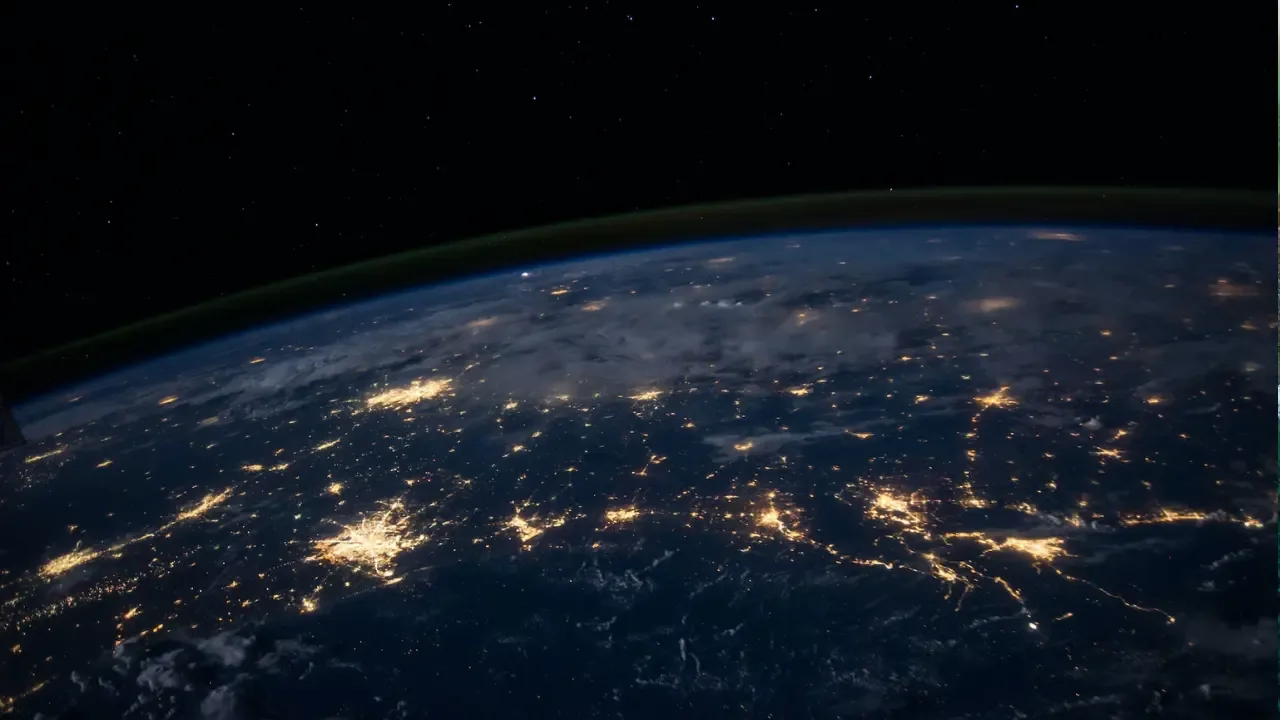
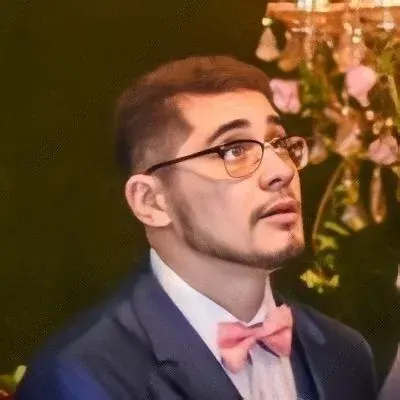
AngularJS: Factory and Service?
Are you struggling to understand the difference between AngularJS factory and service? 🤔 Don't worry, you're not alone! Many developers have faced this confusion. In this blog post, we will dive deep into the functionality and use cases of both factory and service in AngularJS, provide easy-to-understand examples, and help you decide when to use each of them. Let's get started!
Understanding the Context
Before we jump into the comparison, let's quickly review the context of this question. The original post is from someone who was confused about the functional difference between AngularJS factory and service. They had read an article and tried some code examples, but still couldn't see a clear distinction. They even went as far as changing their code from factory to service, and surprisingly, it worked the same way. So, what's the deal?
Factory and Service, What's the Difference?
The confusion around factory and service in AngularJS is quite common. 🤷♀️ To understand the difference, let's take a closer look at how each is implemented.
Factory
In AngularJS, a factory is a function that returns an object. This object can have properties, functions, or any other data that you need. When you inject a factory into a controller or service, AngularJS instantiates the factory and provides you with the object it returned.
Service
On the other hand, a service in AngularJS is a constructor function. It is instantiated using the 'new' keyword, just like any regular JavaScript constructor. When you inject a service into a controller or service, AngularJS creates a new instance of the service using the 'new' keyword and provides it to you.
Example Time!
Now that we have a basic understanding of factory and service, let's look at some examples to illustrate the difference.
Suppose we have an app that needs a "VaderService" to create a Darth Vader object. Here's how we can implement it using factory and service:
Factory Example
var module = angular.module('MyApp.services', []);
module.factory('VaderService', function () {
var VaderClass = function (padawan) {
this.name = padawan;
this.speak = function () {
return 'Join the dark side ' + this.name;
};
};
return VaderClass;
});
Service Example
var module = angular.module('MyApp.services', []);
module.service('VaderService', function () {
var VaderClass = function (padawan) {
this.name = padawan;
this.speak = function () {
return 'Join the dark side ' + this.name;
};
};
return new VaderClass();
});
In both examples, we define a "VaderClass" constructor function with "name" and "speak" properties. The key difference lies in how we return the object. In the factory example, we return the constructor function itself, while in the service example, we return a new instance of the constructor function.
So, What's the Big Deal? 🤔
You might be wondering, if both factory and service provide the same functionality, why bother with two different concepts? The answer lies in the use cases and flexibility.
Factory Use Cases
Factories are widely used when you need multiple instances of an object to be created. Since factories return an object, you can have multiple instances with different states. This can be very useful in scenarios where you need to reuse a set of functionalities for different instances.
Service Use Cases
Services are great when you need a singleton object throughout your application. Since a new instance of the service is created only once and then shared, it can maintain state across different components. Services are ideal for maintaining shared data and functionalities.
Conclusion and Call-to-Action
To wrap up, the main difference between AngularJS factory and service lies in how objects are instantiated and shared. While factories return an object that can create multiple instances, services are instantiated once and shared throughout the app.
Now that you understand the difference, it's time to put your knowledge into action. Analyze your application's requirements and decide whether you need multiple instances (factory) or a singleton (service). Choose the one that fits your use case and start coding!
We hope this blog post helped you clarify the confusion around factory and service in AngularJS. If you found this post helpful, share it with your developer friends who might also be struggling with this concept. Comment below and let us know your thoughts and experiences with AngularJS factories and services. Happy coding! 👩💻👨💻