AngularJS : Clear $watch
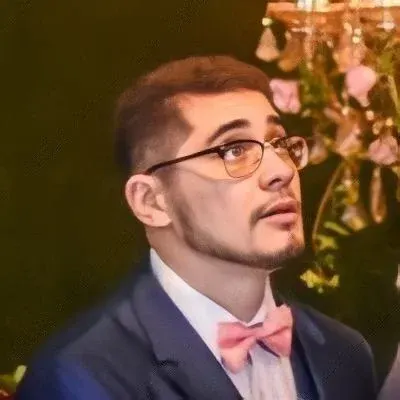
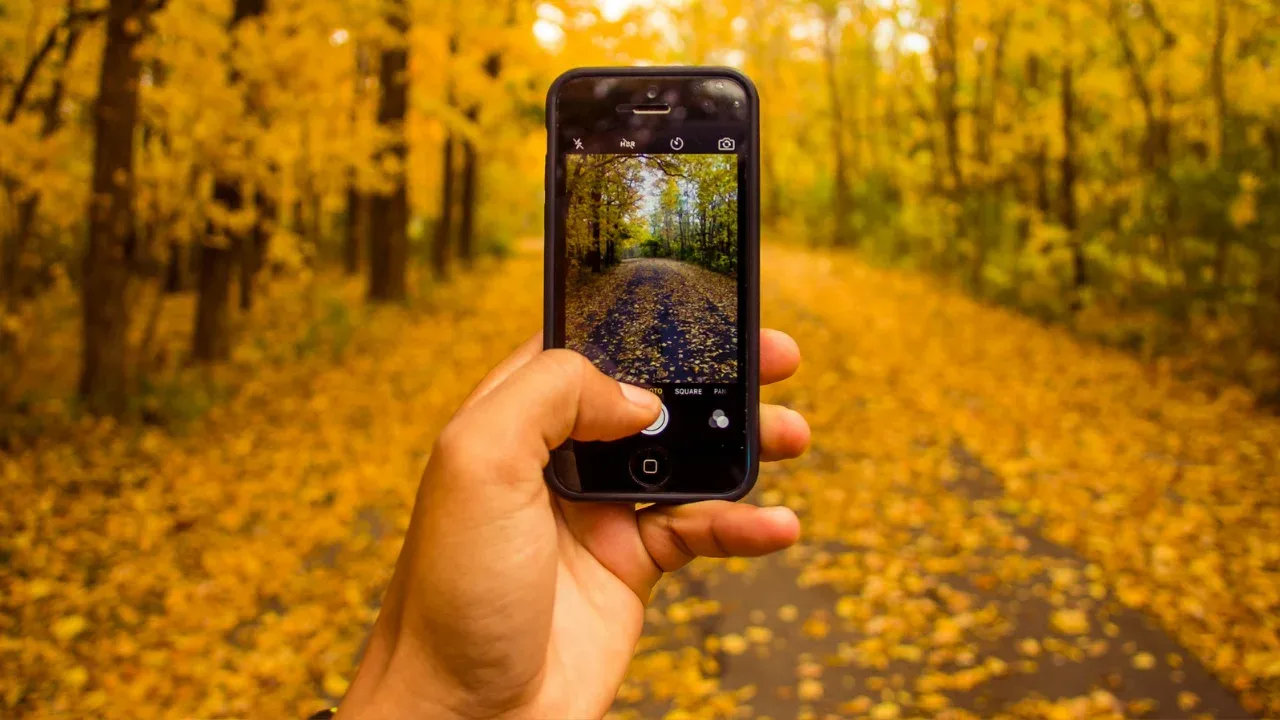
🌈AngularJS : Clear $watch🌈
So you have a watch function in your AngularJS application that is causing some trouble? We've all been there! But worry not, because in this guide, I'll show you how to clear that pesky $watch and get things back on track. 💪
🧐 The Problem
Let's dive into the issue you're facing. You have a watch function in your AngularJS application, like this:
$scope.$watch('quartzCrystal', function () {
// Do something awesome here
});
But there's a catch. After a certain condition is met, such as changing the page in your single-page application, you want to stop that watch. It's like clearing a timeout before it triggers. 🤔
🔍 The Solution
The good news is that there's a simple solution to clear that $watch. AngularJS provides a neat feature called deregistering the watch. This feature allows you to remove the watch function and prevent it from being triggered again. Here's how you can do it:
var unregister = $scope.$watch('quartzCrystal', function () {
// Do something awesome here
});
// Later when you want to clear the watch:
unregister();
In the above code, we store the return value of $scope.$watch
in a variable called unregister
. This value is actually a function that, when called, removes the watch. By invoking unregister()
, you clear the watch and stop it from executing any further. Pretty cool, right? 😎
💡 Example Usage
Now, let's see this solution in action with a practical example. Imagine you have a "Product Details" page in your AngularJS application. Upon navigating to a different page, you want to stop watching changes to the product's data.
function ProductDetailsController($scope) {
// ...
// Start watching changes to the product
var unregister = $scope.$watch('product', function () {
// Do something awesome here
});
// When navigating to a different page
$scope.navigateToOtherPage = function () {
// Clear the watch
unregister();
}
}
In the above code snippet, we define a controller for the "Product Details" page. When the page loads, we start watching changes to the product
variable. But when the user decides to navigate to a different page, we call unregister()
to clear the watch. This way, we prevent any unnecessary execution of the watch function and keep things running smoothly. 🏃♀️💨
🌟 Your Turn to Shine
Alright, now you have the knowledge to clear a $watch in AngularJS. Go ahead and implement this technique in your own codebase to overcome those watch-related challenges. Remember, deregistering the watch is your secret weapon! 🔥
If you have any questions or want to share your experience with clearing $watch, feel free to leave a comment below. Let's help each other build amazing AngularJS applications! 🚀
Don't forget to like and share this post if you found it helpful. 👍 Together, let's spread the word and make AngularJS development a breeze for everyone! 💙
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
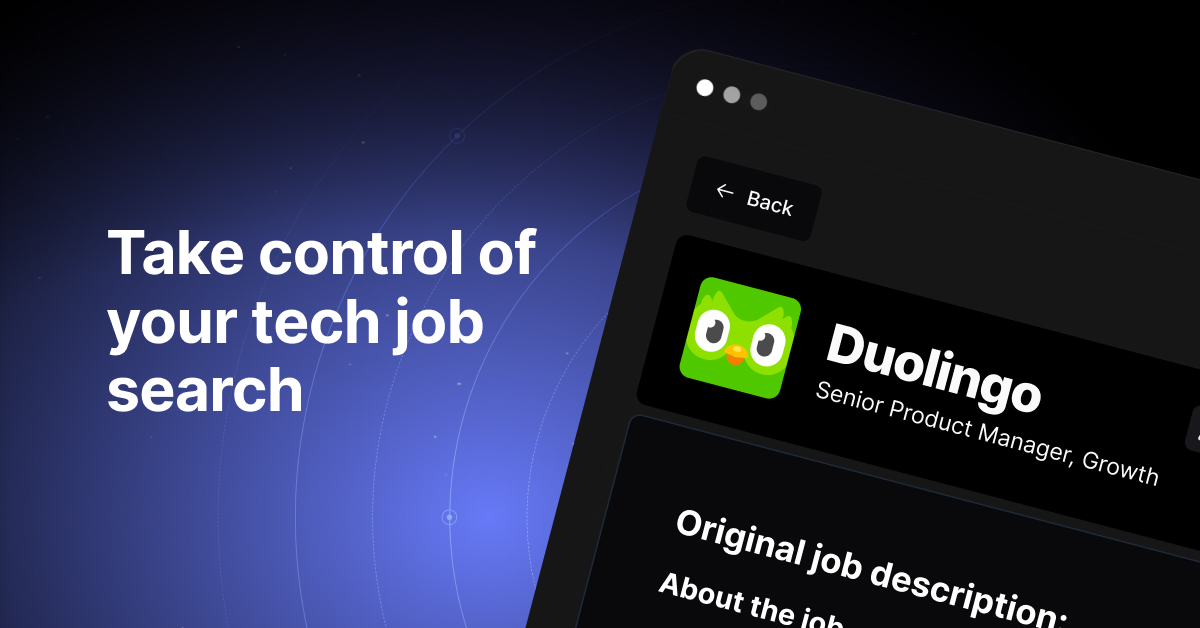