Angular window resize event
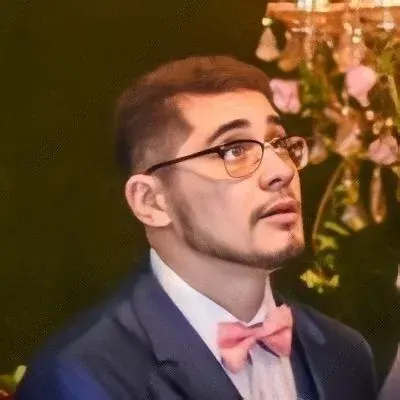
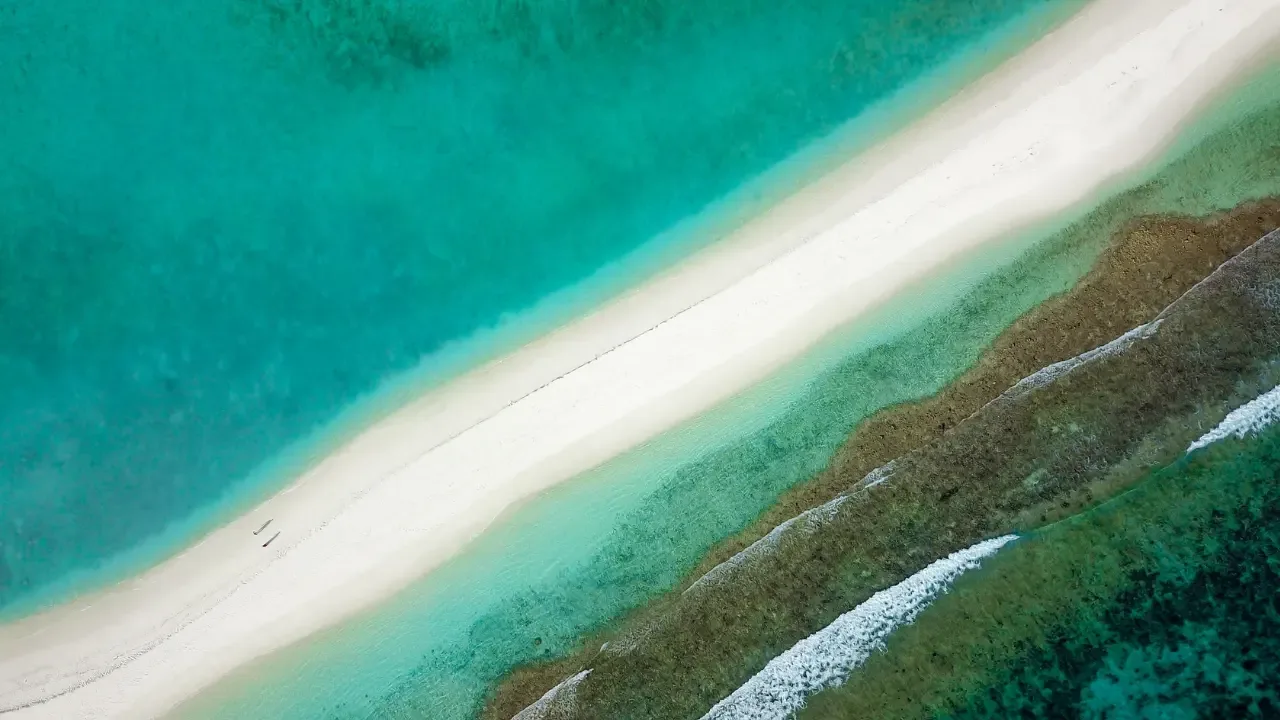
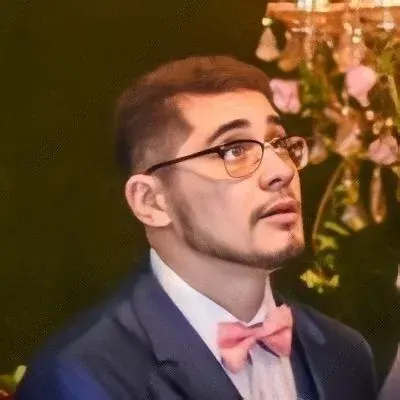
🖥️ Angular Window Resize Event: Easy Solutions for Retrieving Width and Height
Are you having trouble retrieving the width and height from an Angular window resize event? Don't worry, we've got you covered! In this blog post, we will address this common issue and provide you with easy solutions to help you get the desired results. So let's dive right in!
📋 The Problem
The user is looking to perform certain tasks based on the window resize event in their Angular application. They have already set up the event listener in their DOM, and the event is correctly firing. However, they are unsure how to retrieve the width and height from the event object.
💡 The Solution
To retrieve the width and height from the window resize event object in Angular, you can utilize the window.innerWidth
and window.innerHeight
properties. These properties provide the width and height of the browser window, respectively.
In your code snippet, you can modify the onResize
method in your AppComponent
as follows:
export class AppComponent {
onResize(event) {
const width = event.target.innerWidth;
const height = event.target.innerHeight;
console.log(`Width: ${width}, Height: ${height}`);
}
}
By accessing the innerWidth
and innerHeight
properties of the event.target
, you will be able to retrieve the current width and height of the browser window when the resize event occurs. You can then perform your desired tasks or calculations based on these values.
🤓 Example Usage
Let's say you want to dynamically change the background color of an element based on the window's width. Here's an example of how you can achieve this using the retrieved width from the resize event:
export class AppComponent {
onResize(event) {
const width = event.target.innerWidth;
const element = document.getElementById('myElement');
if (width > 768) {
element.style.backgroundColor = 'blue';
} else {
element.style.backgroundColor = 'red';
}
}
}
In this example, we retrieve the window's width using event.target.innerWidth
and then use an if
statement to conditionally change the background color of an element based on the width.
📢 Your Turn to Engage!
Now that you have learned how to retrieve the width and height from the Angular window resize event, it's time to put your newfound knowledge into practice!
We would love to hear about the tasks or functionalities you plan to implement based on the window's dimensions in your Angular application. Share your thoughts, ideas, or even code snippets in the comments section below. Let's learn and grow together!
So what are you waiting for? Get coding, and let the window resize event empower your Angular application! 💪🚀
Please note: Keep in mind that you may need to handle additional logic or considerations specific to your project's requirements. The solutions provided in this blog post are intended to serve as a foundation and may require customization based on your specific use case.
Happy coding and happy window resizing! 😉