Angular HTTP GET with TypeScript error http.get(...).map is not a function in [null]
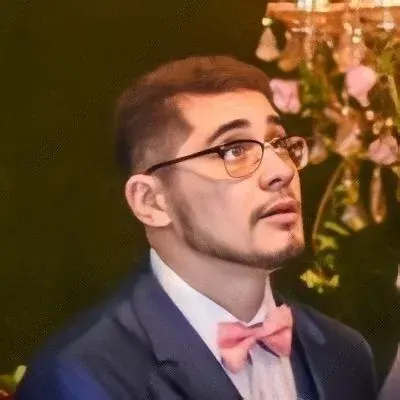
![Cover Image for Angular HTTP GET with TypeScript error http.get(...).map is not a function in [null]](https://images.ctfassets.net/4jrcdh2kutbq/6XyqXj45QZ4M3eNKD0twkA/66b2d2f6d8c48cc92347d3e7bb893d5e/Untitled_design__14_.webp?w=3840&q=75)
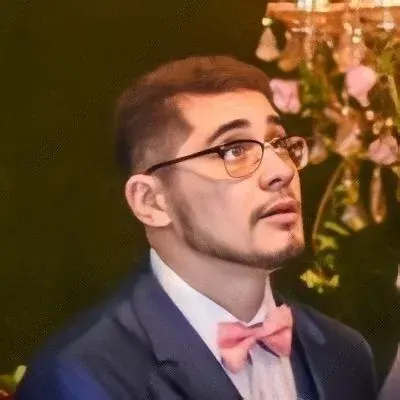
š Title: Fixing 'http.get(...).map is not a function' Error in Angular
š Hey there, Angular developer! Are you facing an issue with the Angular HTTP GET method and TypeScript? If you're seeing the dreaded http.get(...).map is not a function in [null]
error, don't worry ā I've got your back! In this blog post, I'll walk you through the common causes of this error and provide easy solutions to fix it. So, let's dive in and get your code running smoothly again!
The Problem
š” Before we jump into the solutions, let's understand the problem first. In the provided code, it seems that the http.get(...).map
is throwing an error, specifically a TypeError
. This error usually occurs when the map
function is not recognized as a function on the returned Observable š from the http.get
method.
The Root Cause
š The most likely reason for this error is a missing import of the map
operator from the rxjs/operators
package. The map
operator is responsible for transforming the response from the HTTP request š into a JSON object. Without the correct import, the map
function will not be recognized, hence throwing the error.
The Solution
ā
Let's address this issue by adding the necessary import to the code. Open the hall.service.ts
file and update the import statements at the beginning as follows:
import { map } from 'rxjs/operators';
Then modify the getHalls
method to include the imported map
operator:
getHalls() {
return this.http.get(HallService.PATH + 'hall.json').pipe(
map((res: Response) => res.json())
);
}
By including the map
operator within the pipe
function, you are indicating to Angular that you want to apply the map
transformation to the HTTP response.
Verifying the Fix
š Now that we've made the necessary changes, let's verify if the error has been resolved. Check the HallListComponent
and ensure that the HTTP request is correctly mapping the response using the map
operator.
ngOnInit() {
this._service.getHalls().subscribe((halls: Hall[]) => {
this.halls = halls;
});
}
Additional Considerations
š If you're using Angular version 5 or above, please note that the Http
module has been deprecated in favor of the newer HttpClient
module. If you're facing this issue while using HttpClient
, make sure to import the map
operator from rxjs/operators
as shown in the previous solution, and update your code accordingly.
Conclusion
šŖ You're almost there! By following these simple steps, you should be able to fix the http.get(...).map is not a function in [null]
error in your Angular project. Remember to import the map
operator from rxjs/operators
and use it within the pipe
function when mapping the HTTP response.
š I hope this blog post helped you in understanding and resolving the issue. If you have any further questions or need more assistance, feel free to leave a comment below. Happy coding! š
š Don't forget to share this post with your fellow Angular developers who might be facing the same issue. Together, we can help others overcome coding obstacles!