An index signature parameter type cannot be a union type. Consider using a mapped object type instead
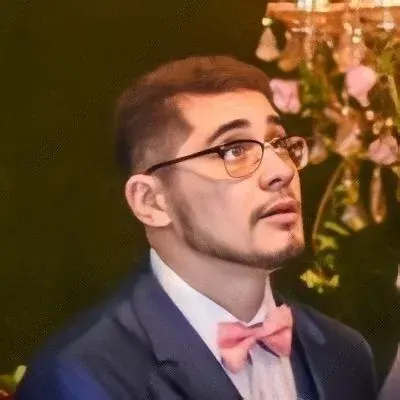
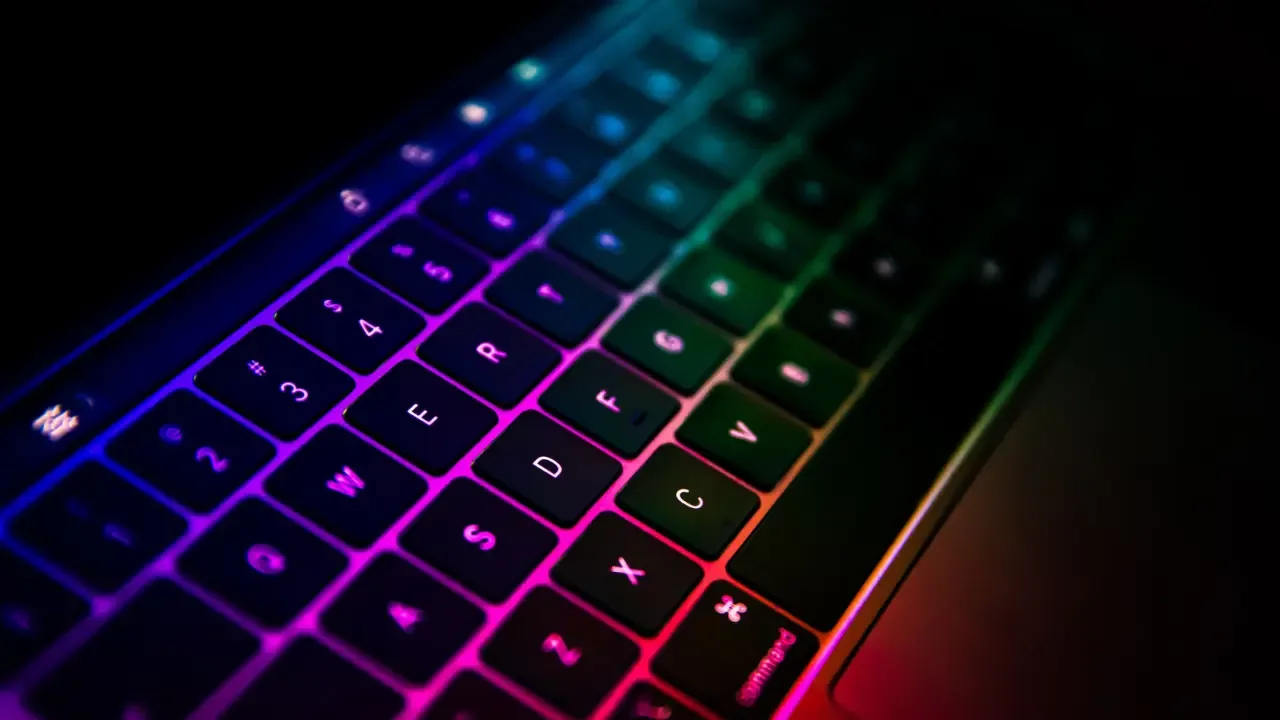
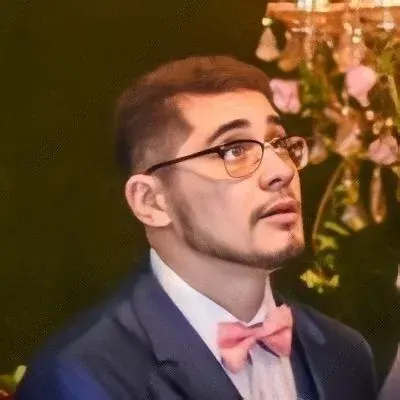
Understanding the Error: Union Types and Index Signatures
First, let's understand the error message you encountered: "An index signature parameter type cannot be a union type. Consider using a mapped object type instead."
In TypeScript, an index signature allows you to define the types of properties that can be accessed using bracket notation, like an array. However, there are some limitations on the types that can be used as index signatures. One of these limitations is that the parameter type of an index signature cannot be a union type.
A union type is a type that represents values that can be of more than one type. For example, string | number
represents a type that can be either a string or a number. In your case, you attempted to use an enum as the parameter type for the index signature, which is a union type of the enum keys.
The Solution: Mapped Object Type
To overcome this limitation, TypeScript provides a concept called a mapped object type, which allows you to define a type that maps keys to specific value types. With mapped object types, you can achieve the desired behavior of using an enum as the index signature parameter type.
Here's how you can refactor your code to use a mapped object type:
enum Option {
ONE = 'one',
TWO = 'two',
THREE = 'three'
}
interface OptionRequirement {
someBool: boolean;
someString: string;
}
type OptionRequirements = {
[Key in Option]: OptionRequirement;
};
In this code, we use the type
keyword to create a new type called OptionRequirements
, which uses a mapped object type. The [Key in Option]
syntax iterates over each key in the Option
enum and assigns it as a key in the OptionRequirements
object type. The value type for each key is OptionRequirement
.
By using this mapped object type, you can now access specific OptionRequirement
values using the keys of the Option
enum, like this:
const requirements: OptionRequirements = {
[Option.ONE]: { someBool: true, someString: 'Hello' },
[Option.TWO]: { someBool: false, someString: 'World' },
[Option.THREE]: { someBool: true, someString: '!' },
};
const optionOneRequirements = requirements[Option.ONE];
console.log(optionOneRequirements); // { someBool: true, someString: 'Hello' }
Share Your Thoughts and Get Involved!
Now that you understand the solution to the error you encountered and how to use mapped object types, it's time to put your knowledge into action!
Did you find this blog post helpful? Have you encountered this error before, and if so, how did you solve it? Share your experiences and let's learn from each other in the comments section below. And don't forget to share this post with your fellow TypeScript enthusiasts and developers to help them overcome this common stumbling block.
Happy coding! 👩💻🔥