Ajax success event not working
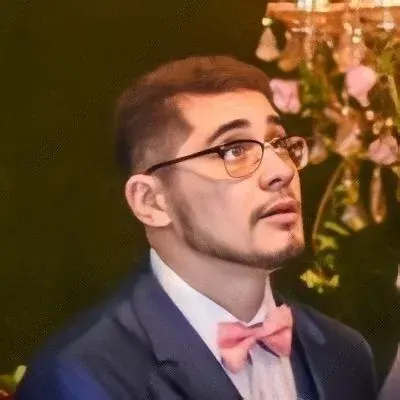
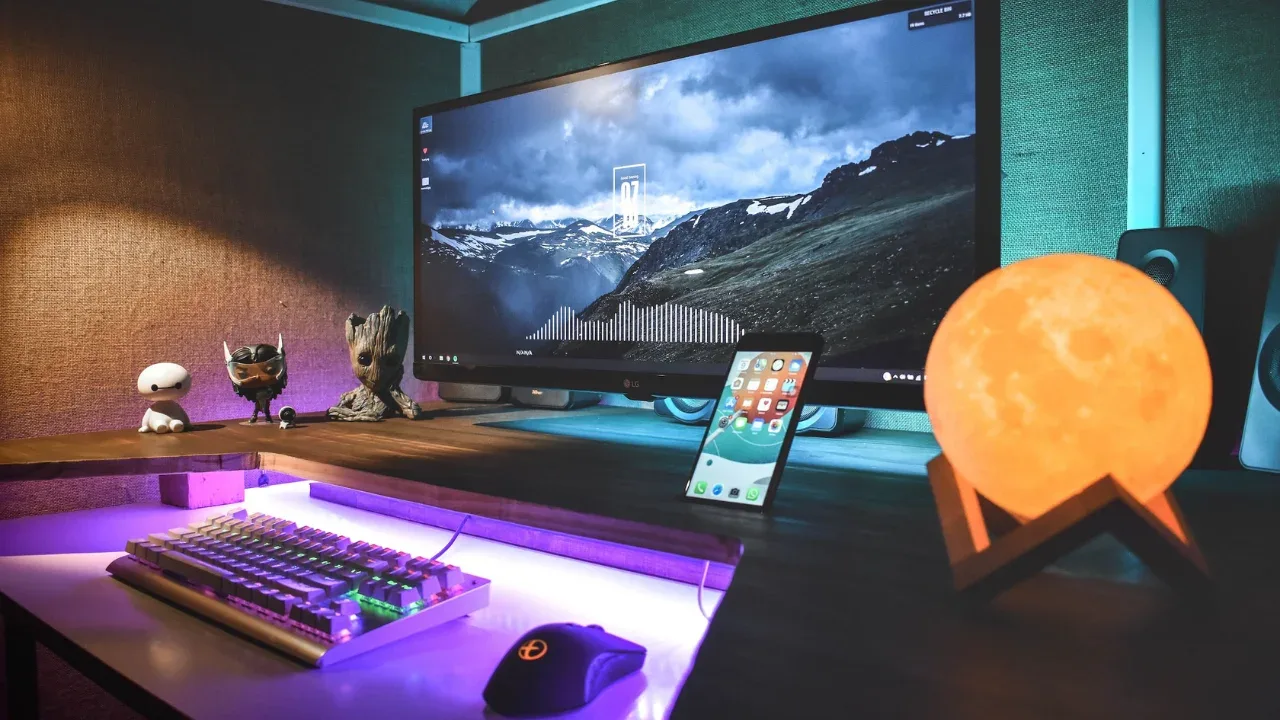
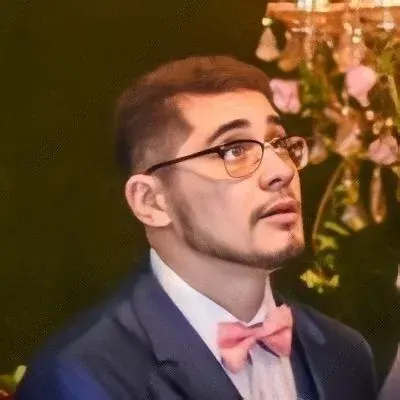
👋 Hey there! Welcome to my tech blog, where I write about all things tech in a fun and easy-to-understand way. Today, we're going to tackle a common issue that many developers face: the "Ajax success event not working" problem. 🤔
So, you're using the $.ajax
function to submit a registration form, but you're having trouble with the success
callback not working as expected. Let's dive right into it and find some easy solutions!
One possible reason why the success
event might not be firing is that the AJAX request might not be returning the expected response. In your code snippet, you have specified the dataType
as 'json'
, which means that the response from the server should be in JSON format. So, let's make sure that the response from submit1.php
is indeed returning valid JSON.
To do that, let's modify your AJAX request code to log the response to the console so that we can see what's being returned. Here's the updated code:
$(document).ready(function() {
$("form#regist").submit(function() {
var str = $("#regist").serialize();
$.ajax({
type: 'POST',
url: 'submit1.php',
data: $("#regist").serialize(),
dataType: 'json',
success: function(response) {
console.log(response); // Log the response to the console
// Rest of your code...
}
});
return false;
});
});
Now, open up your browser's console, submit the registration form, and take a look at the response that's being logged. Make sure that it's in valid JSON format. If it's not, you might need to adjust the response format in submit1.php
so that it matches the expected JSON format.
If the response is indeed returning valid JSON, the next step is to check if the success callback is being triggered but the code inside it is not executing properly. In your case, you want to append a message to an element with the id
of "loading"
. Make sure that this element exists in your HTML code.
Also, it's important to note that the success
callback function can take arguments. One commonly used argument is data
, which represents the response data returned from the server. You can use this argument to access and manipulate the response data. For example:
success: function(data) {
$("#loading").append("<h2>" + data.message + "</h2>");
}
Make sure to replace data.message
with the actual JSON property that contains the message you want to display. This will dynamically append the message to the element with the "loading"
id without requiring a page refresh.
Once you've made these adjustments, give it another go and see if the success
callback is now working as expected. 🚀
I hope this guide has helped you troubleshoot and solve the "Ajax success event not working" problem. If you have any further questions or need more assistance, don't hesitate to drop a comment below. I'm here to help! 😊
Now, it's your turn! Have you ever faced a similar issue when working with Ajax? What were your solutions? Share your experiences in the comments section below! Let's learn from each other and keep the tech community thriving! 💪✨