Ajax request returns 200 OK, but an error event is fired instead of success
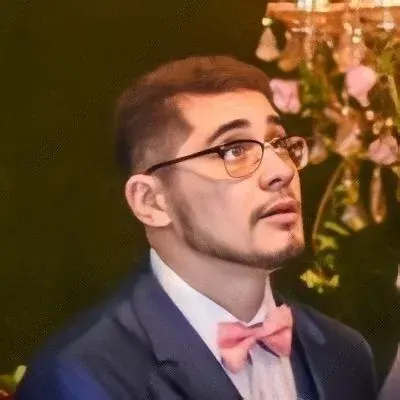
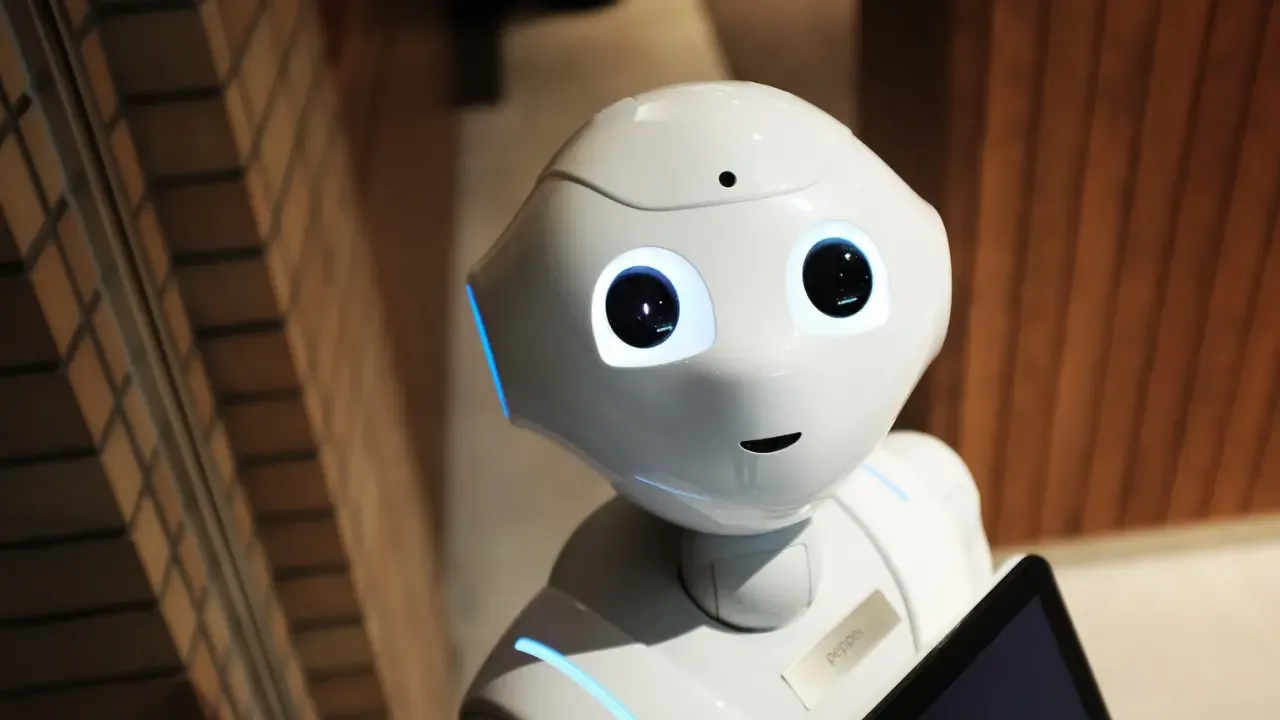
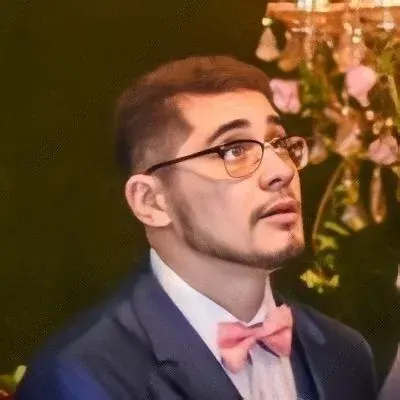
💡 Understanding the Problem
So, you've implemented an AJAX request on your website. The endpoint is called from a webpage, and it always returns a 200 OK
status code. However, instead of triggering the success event as expected, jQuery executes the error event. 🤔
Based on the provided code, it seems that you're trying to delete a row using AJAX with jQuery. The code looks fine at first glance, but let's dive deeper into the issue and find a solution. 💪
🚦 Possible Causes
There are a few potential causes for this problem:
Incorrect AJAX configuration: The AJAX request might be missing some important configuration options or have incorrect values.
Server-side issue: The server might not be responding as expected, causing the error event to be triggered.
👨🔧 Troubleshooting Steps
Let's take a step-by-step approach to troubleshooting the issue:
1. Check the AJAX Configuration
Double-check the AJAX configuration in your code to ensure everything is set up correctly. Here are a few specific areas to focus on:
URL: Make sure the URL points to the correct endpoint that handles the deletion operation (
Jqueryoperation.aspx?Operation=DeleteRow
in your case).Data: Ensure that the data being sent in the AJAX request is formatted correctly. In your code, it seems that you're sending a JSON string (
var json = "{'TwitterId':'" + row + "'}";
). JSON data should typically be enclosed in double quotes ("
), not single quotes ('
).Content Type: Verify that the
contentType
property is set to'application/json; charset=utf-8'
if you intend to send JSON data.
2. Debug the Server-side Code
Since the error event is triggered on the client-side, it's essential to investigate the server-side code as well. In your case, the server-side logic is written in C#.
Check the following aspects of your server-side code:
Page_Load
Method: Ensure that thePage_Load
method is called properly and that it triggers thetest
method correctly.test
Method: Debug thetest
method to ensure that it executes successfully. You can add debugging statements or use breakpoints to verify this.
3. Inspect the Response
To gain more insights, we can examine the response received from the server when the error event is triggered. Modify the AjaxFailed
function in your JavaScript code to include the following code:
function AjaxFailed(result) {
console.log(result); // Output the result to the browser console
alert(result.status + ' ' + result.statusText);
}
Now, when the error event is fired, open the browser console (usually accessible with F12), and inspect the logged result
object. This will provide detailed information about the error that occurred, such as the status code and status text.
🛠️ Possible Solutions
Based on the troubleshooting steps, here are a few potential solutions to consider:
Fix the JSON Data Format: Ensure that the JSON data you're sending in the AJAX request is formatted correctly. Use double quotes (
"
) instead of single quotes ('
) when creating the JSON string in your JavaScript code.Check the Server-side Code: Debug the server-side code (
JqueryOpeartion.aspx
) to make sure thetest
method is executing correctly and returning the expected response.Inspect the Response: Examine the response received in the
AjaxFailed
function to get more detailed information about the error. This will help identify any issues on the server-side that might be causing the error event to be triggered.
✅ Conclusion
By going through the troubleshooting steps and considering the possible solutions, you should be able to identify and resolve the issue with your AJAX request. Remember to double-check the AJAX configuration, debug the server-side code, and inspect the response for more insights.
If you still face challenges or have any further questions, don't hesitate to reach out. We're here to help you! 🙌
📣 Call to Action
Have you ever encountered similar issues with AJAX requests? How did you solve them? Share your experiences and tips in the comments below! Let's help each other troubleshoot these pesky problems. 😄