Adding options to a <select> using jQuery?
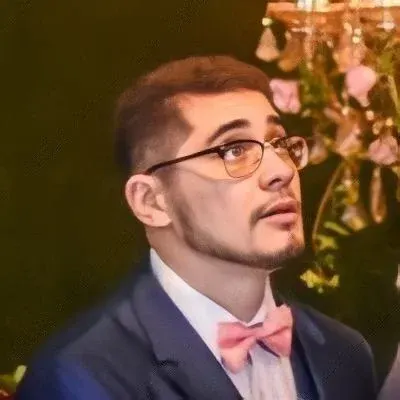
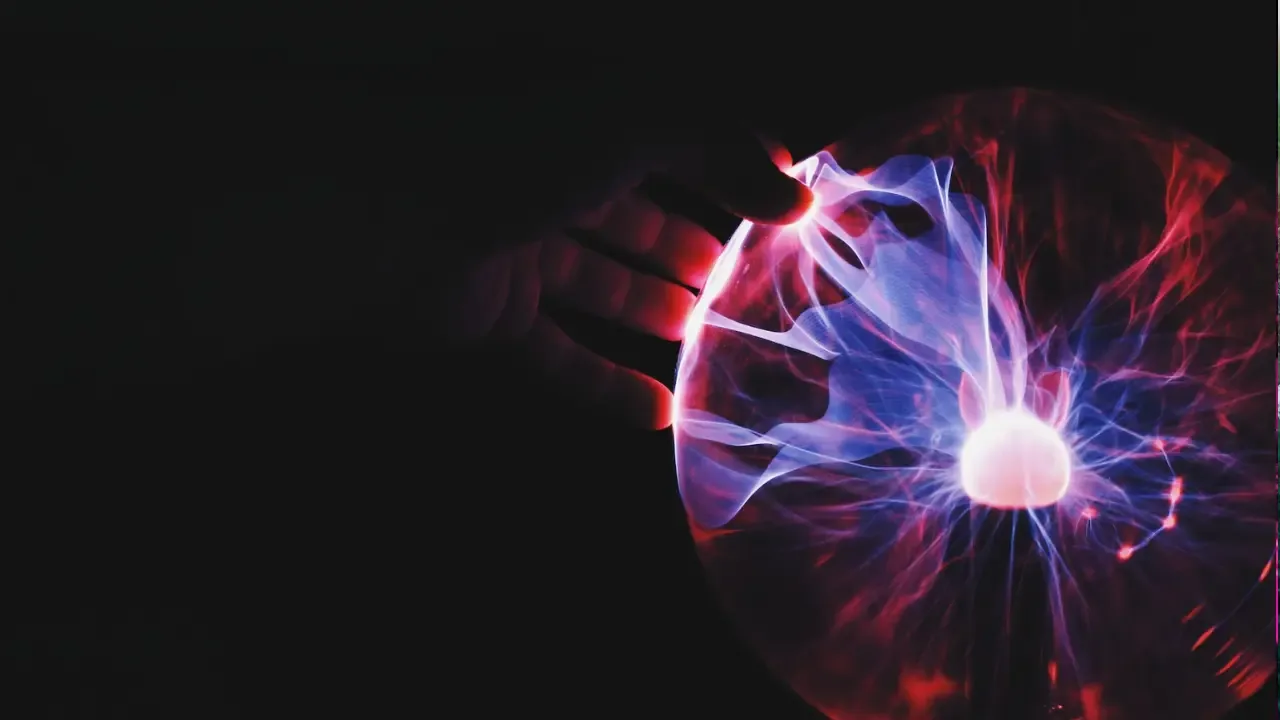
Adding Options to a <select> using jQuery? Easy peasy! 🎉
If you're wondering what's the easiest way to add an <option>
to a dropdown using jQuery, you've come to the right place! 💡
The common approach is to use the .append()
method to add the new option dynamically. Let's take a look at the example provided:
$("#mySelect").append('<option value=1>My option</option>');
✅ Will this work? Absolutely! This code will successfully add a new option with the value 1
and the label "My option" to the dropdown with the ID mySelect
.
But wait, there's more! 🚀
While the code above does the job, there's an even cooler way to conveniently add options to a <select>
element using jQuery. Instead of manually appending the option, we can utilize the .append()
method in a smarter way.
Let me show you an example:
const option = $('<option>', {
value: 2,
text: 'Another option'
});
$("#mySelect").append(option);
🌟 Voila! By creating a new <option>
element using the $('<option>')
syntax, we can easily set the value and label of the option using the properties value
and text
.
What about adding multiple options at once? 🌌
Adding a single option is great, but what if you have multiple options to add at once? jQuery has got you covered! 🙌
Check out this example:
const options = [
{ value: 1, text: 'Option 1' },
{ value: 2, text: 'Option 2' },
{ value: 3, text: 'Option 3' }
];
$.each(options, function(i, option) {
$("#mySelect").append($('<option>', option));
});
🎯 Boom! By utilizing the $.each()
method, we can iterate over an array of options and dynamically add each one of them to the dropdown. Cool, isn't it?
Time to show your jQuery skills! 💪
Now that you know how to add options to a <select>
using jQuery, it's time to put your knowledge into practice! Give it a try in your own project and see the magic happen. 🪄✨
If you have any questions, suggestions, or other jQuery tricks up your sleeve, we'd love to hear from you! Share your thoughts in the comments below and let's get the conversation rolling! 😄📝
Happy coding! 💻🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
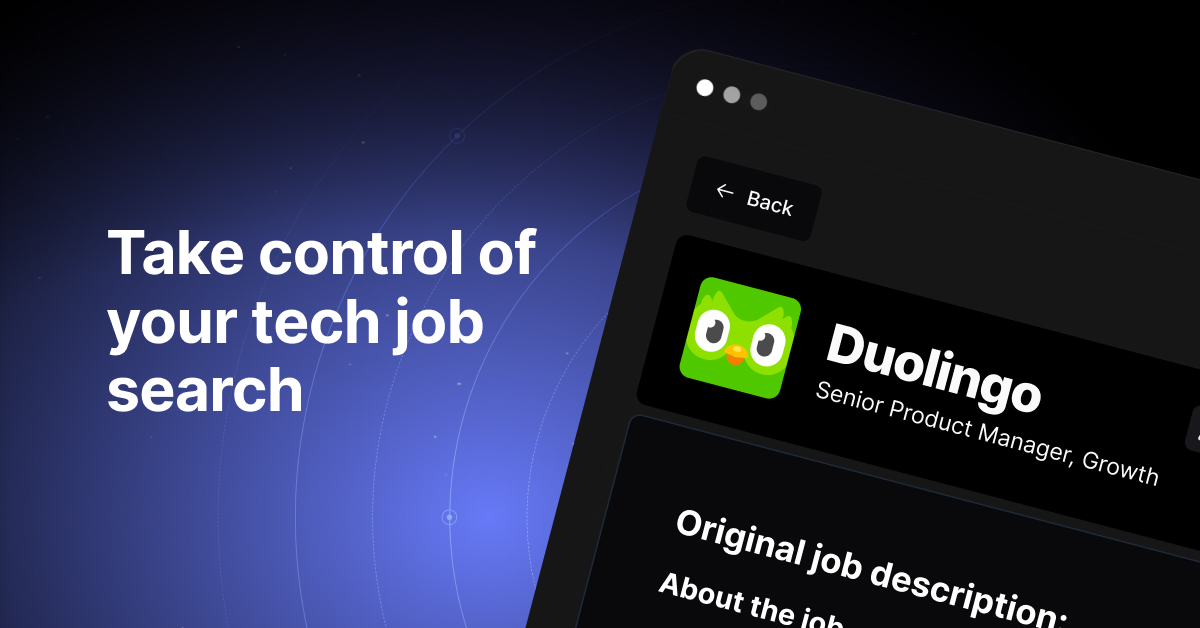