Adding a table row in jQuery
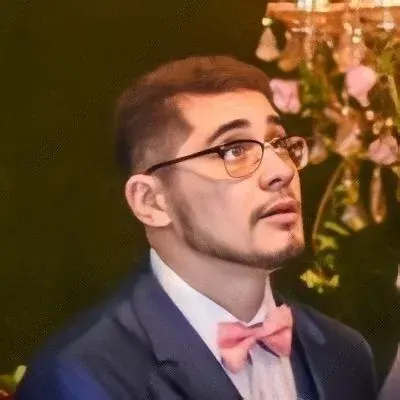
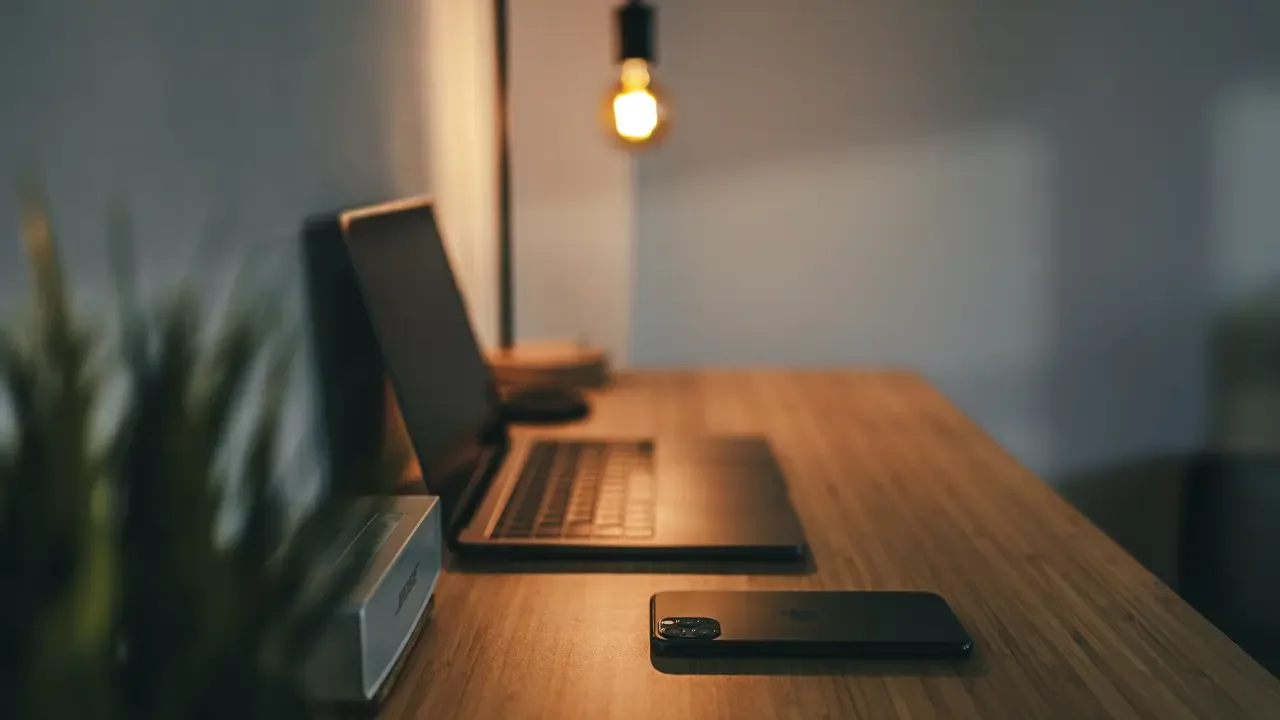
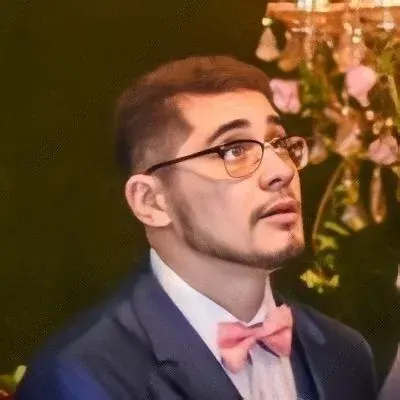
🌟 Adding a Table Row in jQuery Made Easy: Common Issues and Solutions 🌟
So, you want to add an additional row to your table using jQuery? Awesome! It's a handy trick that can come in handy when dynamically updating your table's content. In this blog post, we'll address common issues, provide easy solutions, and explore alternative methods. Let's dive right in! 💪
The Classic Append Method:
The code snippet you provided demonstrates the classic way to add a table row in jQuery:
$('#myTable').append('<tr><td>my data</td><td>more data</td></tr>');
This approach is simple, concise, and works perfectly fine for adding a row to your table. However, there are some limitations and considerations you should be aware of. Let's discuss them one by one. 🤔
Limitations and Considerations:
1. Complex Elements:
The append method is great for adding simple HTML elements like text, but things can get a bit more complicated when it comes to adding inputs, selects, or other complex elements. Here's an example:
$('#myTable').append('<tr><td><input type="text" /></td><td>more data</td></tr>');
In this case, the input element will be added as a string, not a live input field. To overcome this limitation, you can use the .clone()
method to create a copy of the desired element and then append it to the table row. Here's how:
var $input = $('<input type="text" />');
$('#myTable').append('<tr><td></td><td>more data</td></tr>');
$('#myTable tr:last-child td:first-child').append($input.clone());
By cloning the input element, you ensure that the cloned element is independent and retains its functionality. 🔄
2. Numerous Rows:
Appending rows one by one can be a cumbersome task, especially when you have a large number of rows to add dynamically. In such cases, repeatedly calling .append()
can impact the performance of your webpage. A more efficient approach is to construct the entire table row as a string and then append it in one go. Here's an example:
var newRow = '<tr><td>row data 1</td><td>row data 2</td></tr>';
$('#myTable').append(newRow);
By constructing the entire row as a string, you reduce the number of DOM manipulation operations, resulting in improved performance. 🚀
Alternative Method: HTML Fragments
If you're looking for an alternative to the .append()
method, you can use HTML fragments to add new rows to your table. HTML fragments are basically strings of HTML code that can be inserted into the DOM. Here's an example:
var newRowFragment = $('<tr><td>my data</td><td>more data</td></tr>');
$('#myTable').append(newRowFragment);
By wrapping the table row inside the jQuery function $()
, you create a jQuery object that represents the HTML element. This allows you to treat the HTML string as a native jQuery object and manipulate it accordingly. 🧩
Your Turn to Add Some Rows! 🎉
Now that you've learned the ins and outs of adding table rows in jQuery, it's time for you to give it a try! Experiment with different scenarios, test complex element additions, and optimize your performance using HTML fragments. Feel free to share your experiences or ask any questions in the comments below. Happy coding! 😄
Remember, adding table rows dynamically is just one of the many tricks you can perform with jQuery. If you're hungry for more jQuery tips and tricks, stay tuned for our future blog posts!
📢 Share your thoughts and experiences in the comments below! 🙌