Add new attribute (element) to JSON object using JavaScript
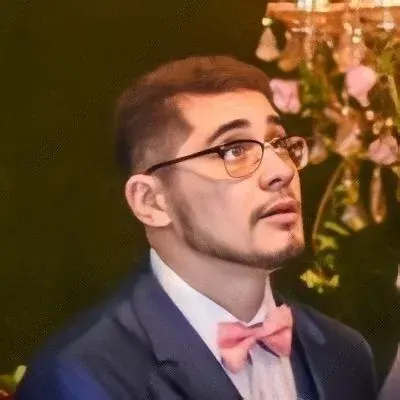
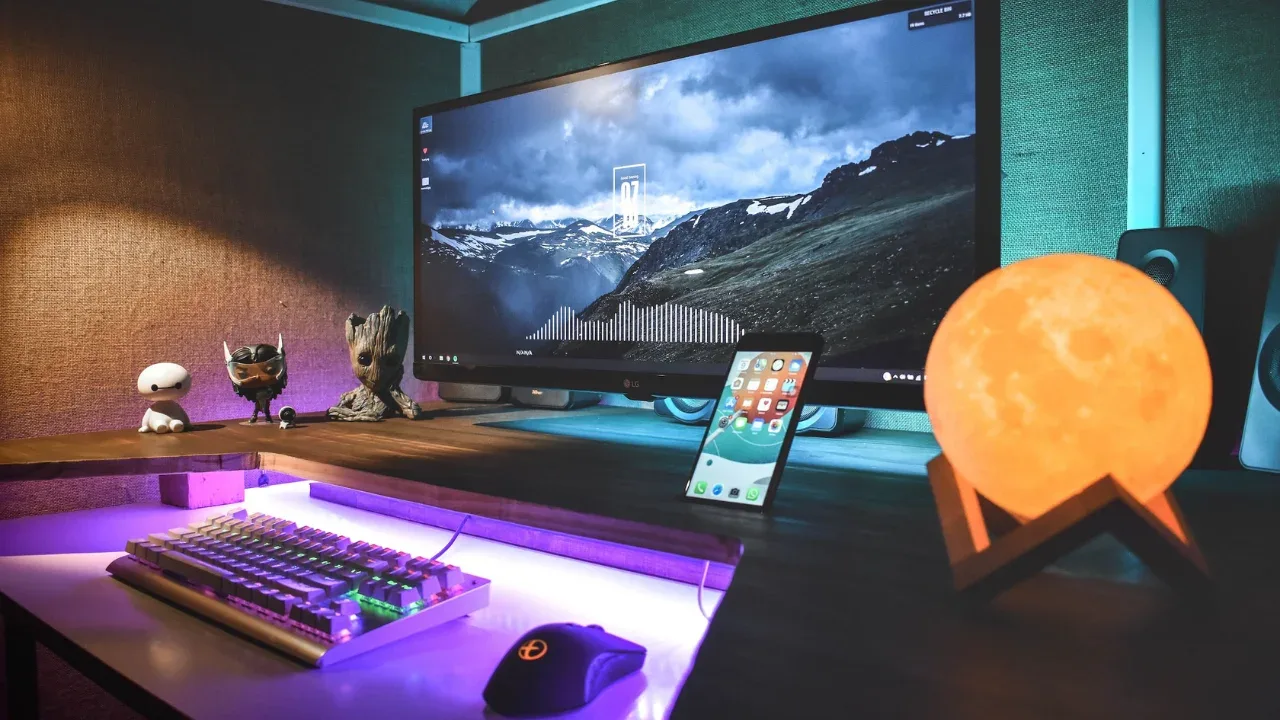
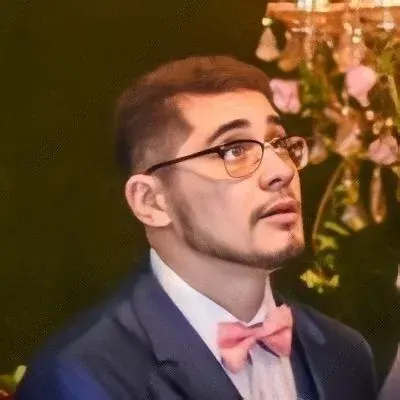
💡 Adding a New Attribute to a JSON Object in JavaScript
So, you've got a JSON object in JavaScript and you want to add a new attribute to it? No problem! In this guide, we'll walk you through the steps to do just that. Whether you're a beginner or an experienced developer, we've got you covered. Let's dive in!
📚 Understanding JSON Objects
Before we start adding new attributes to our JSON object, let's have a quick refresher on what JSON objects are.
JSON, which stands for JavaScript Object Notation, is a lightweight data interchange format. It is commonly used for storing and transmitting data between a server and a web application. JSON objects consist of key-value pairs, where the keys are strings and the values can be any valid JSON data type (e.g., string, number, boolean, array, object).
Now that we have that covered, let's move on to adding a new attribute to our JSON object using JavaScript.
🚀 Adding a New Attribute
To add a new attribute to a JSON object in JavaScript, we can follow these steps:
Retrieve the JSON object you want to modify.
Use the dot notation or square bracket notation to add a new attribute to the object.
Set the value of the new attribute.
Let's see some examples of how we can accomplish this:
// Example 1: Using dot notation
let myObj = { "name": "John", "age": 30 };
myObj.favoriteColor = "blue";
// Example 2: Using square bracket notation
let myObj = { "name": "John", "age": 30 };
myObj["favoriteColor"] = "blue";
In both examples, we start with a JSON object myObj
that has two attributes, name
and age
. We then add a new attribute favoriteColor
with the value "blue"
using either dot notation (myObj.favoriteColor
) or square bracket notation (myObj["favoriteColor"]
).
⚠️ Common Issues and Tips
While adding a new attribute to a JSON object is relatively straightforward, there are a few things to keep in mind:
Make sure you have the correct reference to the JSON object you want to modify. Double-check the variable name and any potential scoping issues.
Check that the attribute you're adding doesn't already exist in the JSON object. If it does, the previous value will be overwritten.
If you're adding a value that requires quotation marks (e.g., a string), make sure to enclose it in double or single quotes.
📣 Call-to-Action
And there you have it! Adding a new attribute to a JSON object using JavaScript is as easy as pie. Whether you prefer dot notation or square bracket notation, you now have the tools to modify your JSON objects in no time.
Are you working on a project where you need to modify JSON objects frequently? Share your experience in the comments below. We'd love to hear from you!