Access props inside quotes in React JSX
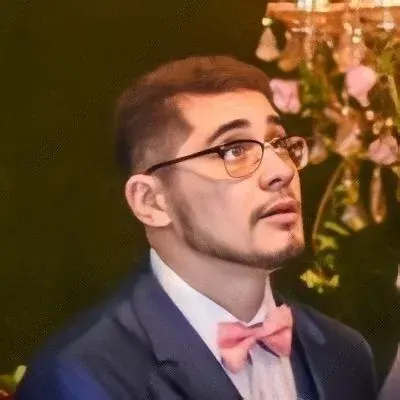
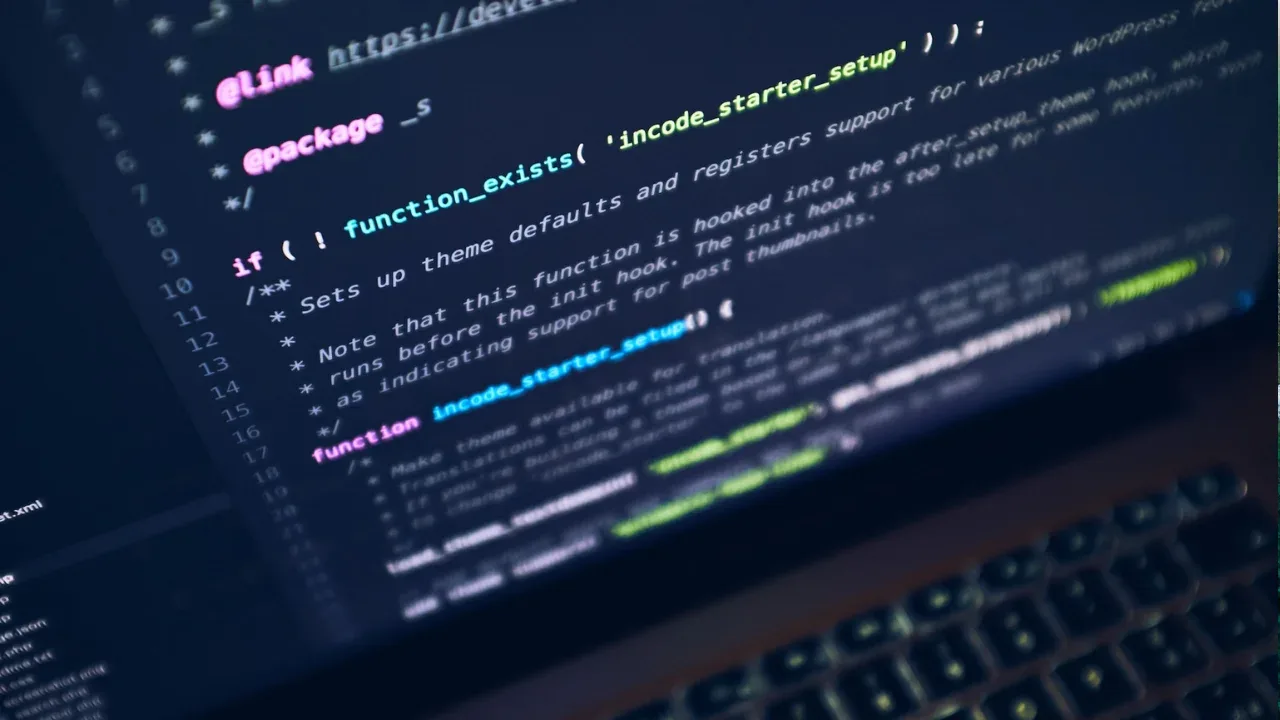
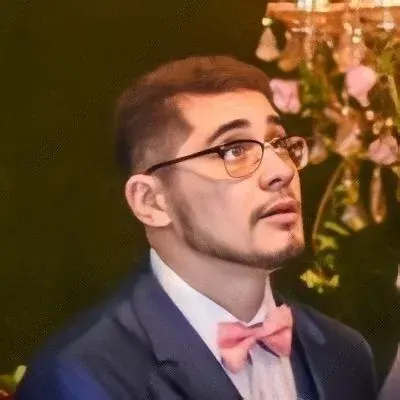
📝🔍 Accessing props inside quotes in React JSX
Are you struggling to reference a value from props
inside a quoted attribute in your React JSX code? Don't worry, we've got you covered! 🙌
When working with JSX, it's common to encounter the need to access values from props
within quoted attribute values. Let's explore a specific example to help you understand how to tackle this challenge.
Consider the following JSX code:
<img className="image" src="images/{this.props.image}" />
In this code snippet, we want to reference the value of this.props.image
within the src
attribute of the img
JSX element.
However, when rendering this JSX code, the resulting HTML output is not what we expected:
<img class="image" src="images/{this.props.image}">
The value of this.props.image
is not being interpolated correctly within the src
attribute. So, how can we resolve this issue? Let's dive into the solutions! 💡
➡️ Solution 1: Using curly braces for expression interpolation
To access a value from props
inside a quoted attribute value, you can use curly braces as an expression interpolation syntax in JSX. To achieve this, we modify the problematic line of code as follows:
<img className="image" src={`images/${this.props.image}`} />
By surrounding the attribute value with backticks (`) and using ${}
inside the curly braces, we can dynamically interpolate the value of this.props.image
into the src
attribute.
When this code is rendered, the resulting HTML output will now correctly include the value of this.props.image
:
<img class="image" src="images/your_image.jpg">
👉 Helpful tip: Make sure to use backticks (`) and not single quotes or double quotes to enable expression interpolation within JSX.
➡️ Solution 2: Concatenating strings
Another approach to accessing props inside quotes is by concatenating strings. Although this is a slightly less concise solution, it gets the job done.
Here's an alternative way to tackle the problem:
<img className="image" src={"images/" + this.props.image} />
By concatenating the string "images/"
with this.props.image
, we can achieve the correct value assignment for the src
attribute.
After rendering, the resulting HTML output will be the same as in the previous solution:
<img class="image" src="images/your_image.jpg">
✅ Problem solved! With either of these solutions, you can successfully reference props inside quotes within your React JSX code.
Now that you're equipped with this knowledge, go ahead and give it a try in your own project! And don't forget to share this guide with other React developers who might be facing the same challenge. Together, we can make coding easier for everyone! 🚀
📢 Let us know in the comments below if you found this guide helpful or if you have any other questions related to React JSX. We're always here to assist you! 💪👇