Access Control Request Headers, is added to header in AJAX request with jQuery
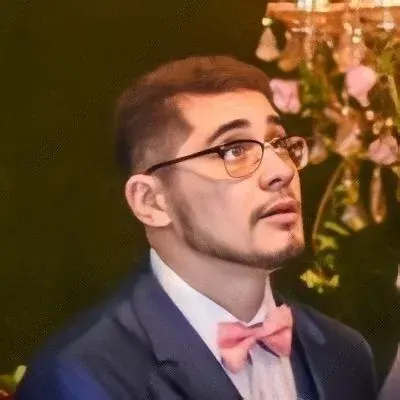
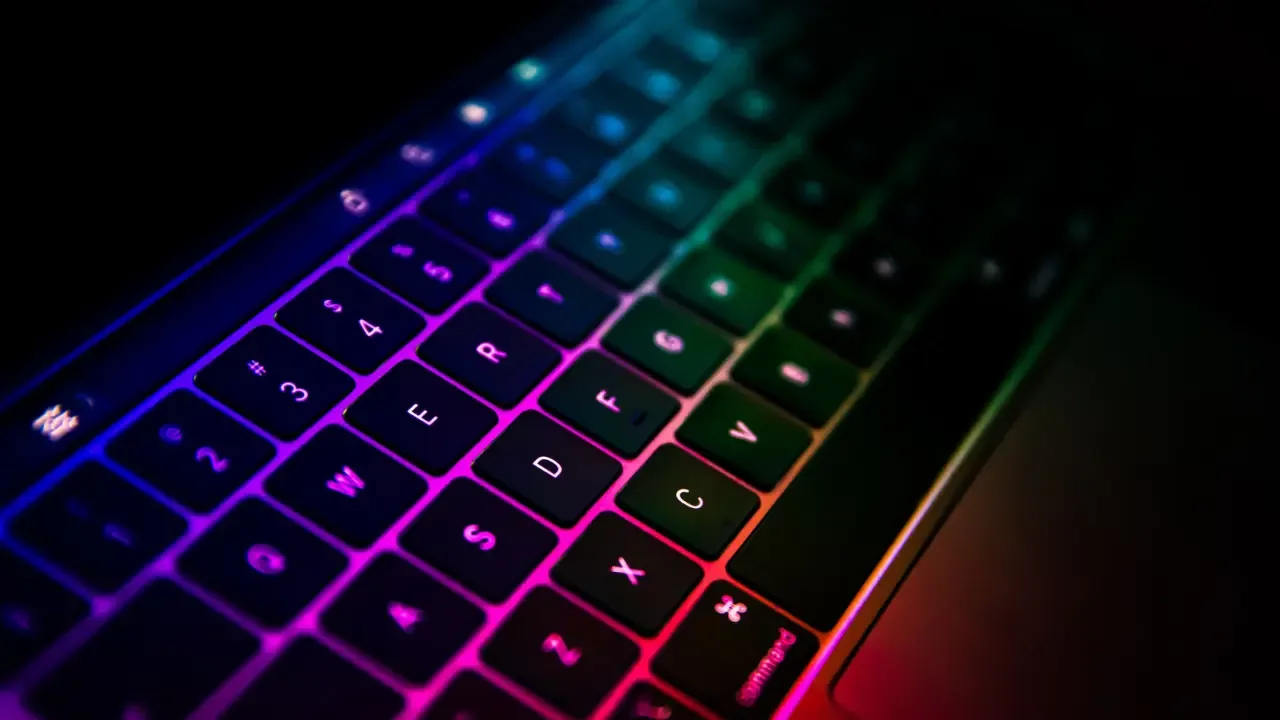
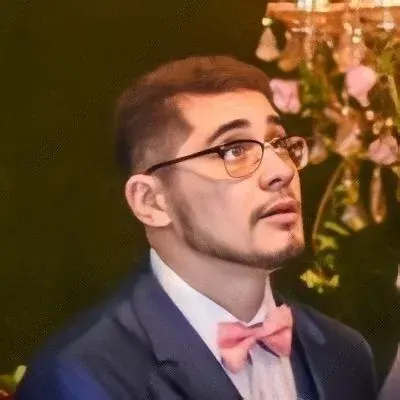
💻 How to Add Custom Headers in AJAX Requests with jQuery
Are you looking to add custom headers to your AJAX POST requests using jQuery? 🤔 Look no further! In this blog post, we'll address a common issue where the headers are not being added correctly and provide easy solutions to fix it. So let's dive in and get your custom headers flowing! 💪
The Problem 🚫
The user tried adding custom headers to an AJAX POST request using the jQuery ajax()
function. They used the headers
option to specify the headers and expected to see them in the actual request. However, when they checked the request headers using FireBug, they found that the custom headers were instead being added to the Access-Control-Request-Headers
header. This was not the expected behavior. 😕
The Explanation 📚
The behavior observed by the user is actually related to the browser's implementation of Cross-Origin Resource Sharing (CORS). CORS is a security mechanism that restricts AJAX requests made across different domains. It involves a preflight request with the OPTIONS
method, which is sent before the actual request to check if the server supports the requested method, headers, and origin. In the preflight request, the headers that were added using jQuery's headers
option are placed in the Access-Control-Request-Headers
header. This is normal and expected behavior when dealing with CORS. ✅
The Solution 💡
To resolve the issue and see the custom headers in the actual request, you can follow one of the below solutions:
Solution 1: Server-side Configuration
Server-side configuration is required to allow and handle CORS requests. You'll need to configure your server to respond to the preflight request with the appropriate headers to allow the custom headers. The server should include the following response headers:
Access-Control-Allow-Origin: *
Access-Control-Allow-Headers: my-first-header, my-second-header
These headers specify that the resource can be accessed from any origin (*
) and that the requested custom headers are allowed. The server would need to respond with these headers for the OPTIONS
request.
Solution 2: beforeSend Callback
Another solution is to use the beforeSend
callback option provided by jQuery's ajax()
function. This option allows you to modify the request object and add custom headers before the request is sent. Here's how you can do it:
$.ajax({
type: 'POST',
url: url,
beforeSend: function(xhr) {
xhr.setRequestHeader("My-First-Header", "first value");
xhr.setRequestHeader("My-Second-Header", "second value");
}
}).done(function(data) {
alert(data);
});
By using the beforeSend
callback, you can directly set the custom headers on the xhr
object. This approach bypasses the preflight request and ensures that the custom headers are included in the actual request. 🚀
Wrapping Up 🎉
Adding custom headers to AJAX requests using jQuery is a common requirement when working with APIs or cross-domain requests. By understanding the behavior of CORS and using the appropriate solutions, you can ensure that your custom headers are added correctly and that your requests work as expected. 💪
So go ahead and give these solutions a try! If you have any questions or other problems you'd like us to address, feel free to leave a comment below. Happy coding! 😊👩💻👨💻
P.S. Don't forget to share this post with your fellow developers who might also find it helpful! Sharing is caring, after all. 💙💌