Abort Ajax requests using jQuery
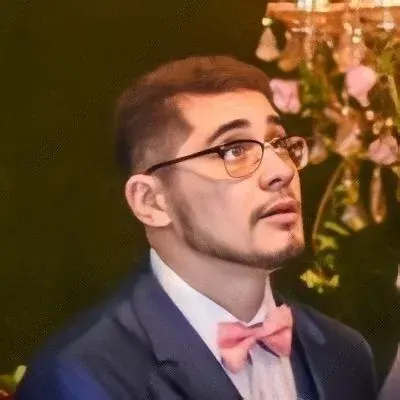
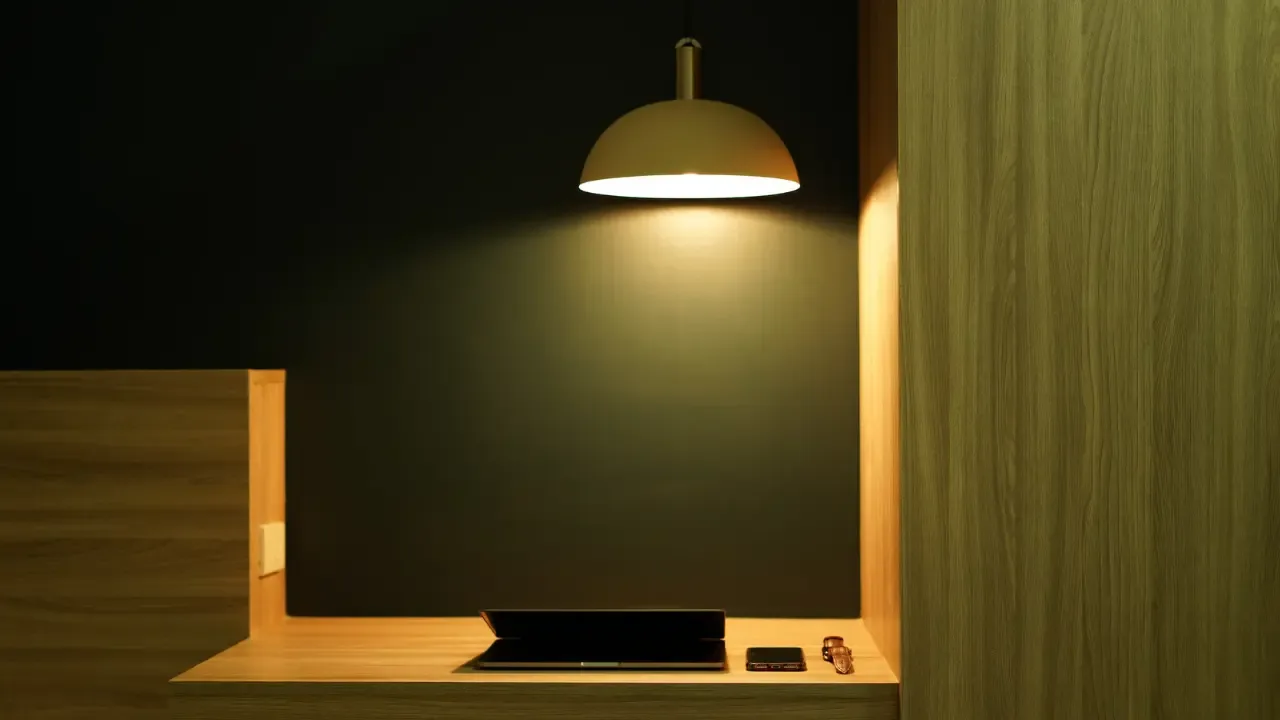
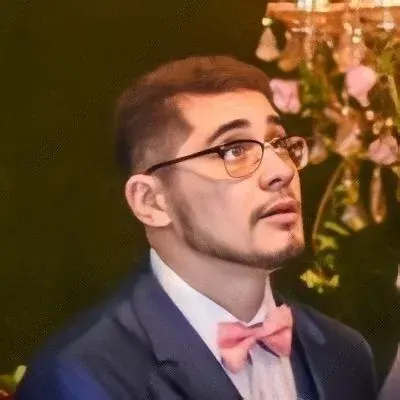
π£Hey there, fellow tech enthusiasts!β¨
Today, we're diving deep into the realm of jQuery and tackling a common issue that many developers face β how to abort Ajax requests.π
Picture this: you're in the middle of sending an Ajax request, eagerly waiting for a response...but suddenly, you realize you don't actually need that response anymore.π« Fear not, my friend, for jQuery comes to our rescue with its handy feature that allows us to gracefully abort those pesky Ajax requests.πͺ
So, let's address the burning question: **Is it possible to cancel/abort an Ajax request using jQuery, even before receiving a response?**π€
π―The Short Answer: Yes, it's absolutely possible!π‘
Now, let's dive into the nitty-gritty details and explore the solutions to quickly and effortlessly abort Ajax requests using jQuery. Here we go!π΅οΈββοΈ
Solution 1: Using the $.ajax() Method
One straightforward approach is to use jQuery's $.ajax()
method.π‘ This method returns an XMLHttpRequest object which can be used to abort the ongoing request. By saving the returned object, you can call the abort()
method whenever you need to cancel the request.
Take a look at this example:
// Saving the request
const request = $.ajax({
url: 'your-url-here',
type: 'GET',
// Other request parameters
});
// Aborting the request
request.abort();
π Easy peasy, right? Just save the request as a variable and call its abort()
method when you're ready to cancel it!
Solution 2: Using the $.ajaxSetup() Method
Another way to handle this is by using $.ajaxSetup()
, which globally sets options for future Ajax requests. By setting the xhr
option to a function and returning the XMLHttpRequest object, you can then abort the request whenever needed.π
Here's an example:
$.ajaxSetup({
xhr: function() {
const xhr = new XMLHttpRequest();
return xhr;
}
});
// Saving the request
const request = $.ajax({
url: 'your-url-here',
type: 'GET',
// Other request parameters
});
// Aborting the request
request.abort();
β‘οΈWith this method, the abort()
functionality is available for all the requests made using jQuery's Ajax methods.
Engage with us! π€
Now that you're armed with these handy solutions, go ahead and give them a try!π§
Have you ever found yourself in a situation where you needed to abort an ongoing Ajax request? Tell us about your experience and any additional techniques you've used. We'd love to hear from you!π¬
Don't forget to share this post with your fellow developers who might be grappling with Ajax request cancellations. Let's spread the knowledge!π
Until next time, happy coding!π©βπ»π¨βπ»