A component is changing an uncontrolled input of type text to be controlled error in ReactJS
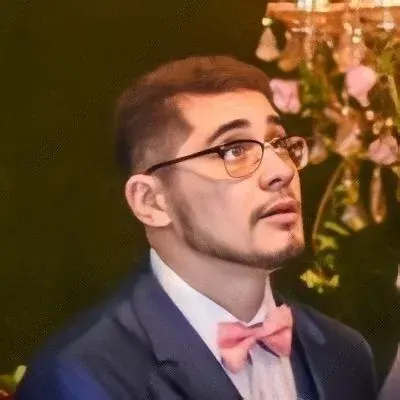
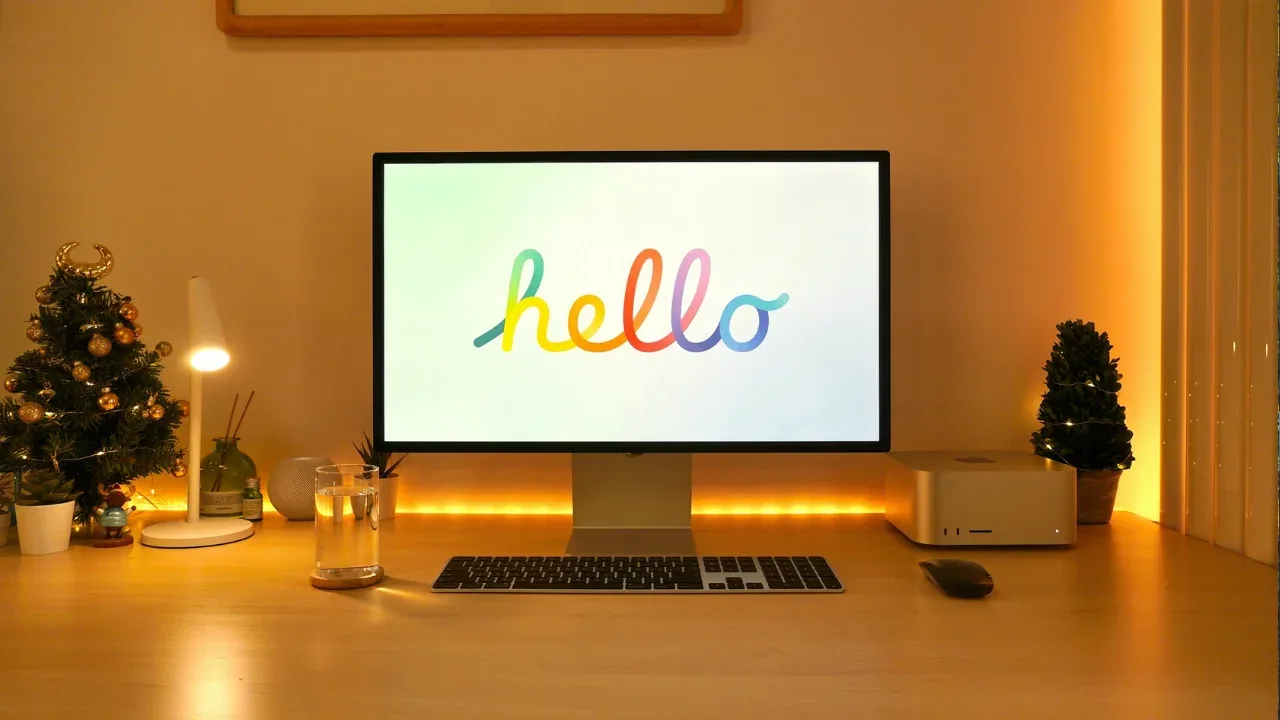
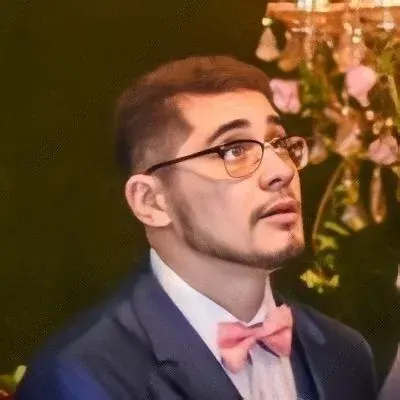
📝 Title: How to Fix the "Changing an Uncontrolled Input to be Controlled" Error in ReactJS
Hey there! Are you experiencing the dreaded "Warning: A component is changing an uncontrolled input of type text to be controlled" error in ReactJS? Don't worry, we've got you covered! In this article, we'll dive into this common issue, provide you with easy solutions, and help you understand the concept of controlled vs uncontrolled input elements in React. Let's get started! 💪🚀
Understanding the Error and Its Cause
When you see the warning message stating that a component is changing an uncontrolled input to be controlled, it means that you're trying to convert an input element from an uncontrolled state to a controlled state (or vice versa) within your ReactJS component. This violates the React principle of maintaining the same type of input element throughout its lifetime.
In the provided code, the input element's state is controlled using the value
prop, which is tied to the fields
object in the component's state. However, changing the value of the input without updating the corresponding fields
state can trigger this error.
Solving the Issue: Controlled vs Uncontrolled Inputs
To resolve this error, you have a couple of options depending on your specific use case. Let's explore them:
Convert the Input Element to Uncontrolled: If you prefer to use an uncontrolled input element, you should remove the
value
prop from theinput
element in therender
method. This will allow it to be uncontrolled and manage its own state.render() { return ( <div className="form-group"> <input onChange={this.onChange.bind(this, "name")} className="form-control" type="text" ref="name" placeholder="Name *" /> <span style={{ color: "red" }}>{this.state.errors["name"]}</span> </div> ); }
Synchronize the Component State: If you prefer to use a controlled input element and maintain the state in your component, you need to ensure that the
fields
state object is always updated when the input value changes. In the provided code, we already have anonChange
method that handles this. Make sure you're correctly updating thefields
object in theonChange
method:onChange(field, e) { let fields = { ...this.state.fields }; // Create a copy of the fields object fields[field] = e.target.value; this.setState({ fields }); }
With these solutions in mind, you can choose the approach that fits your requirements and fix the "changing an uncontrolled input to be controlled" error.
Take Control of Your Input Elements
Remember, the key to avoiding this error and maintaining a smooth ReactJS application lies in understanding the difference between controlled and uncontrolled input elements.
Controlled inputs are tied to the component's state and are updated via the
onChange
event handler. They provide a centralized way to manage and access the input values.Uncontrolled inputs manage their own state internally, without relying on the component's state. They are useful in scenarios where you only need the final input value and don't require real-time updates.
Choose wisely based on the specific requirements of your project. 🤔💡
Join the Conversation!
We hope this guide helped you understand and resolve the "changing an uncontrolled input to be controlled" error in ReactJS. If you still have questions or want to share your experiences, we'd love to hear from you! Leave a comment below and let's keep the conversation going. Happy coding! 🎉🔥