Why is executing Java code in comments with certain Unicode characters allowed?
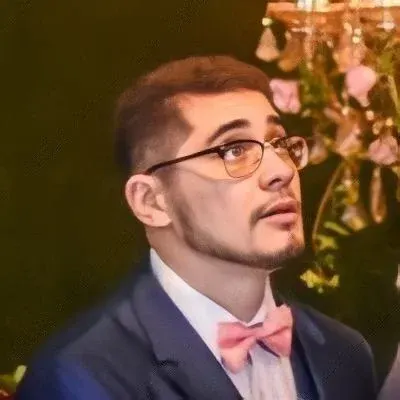
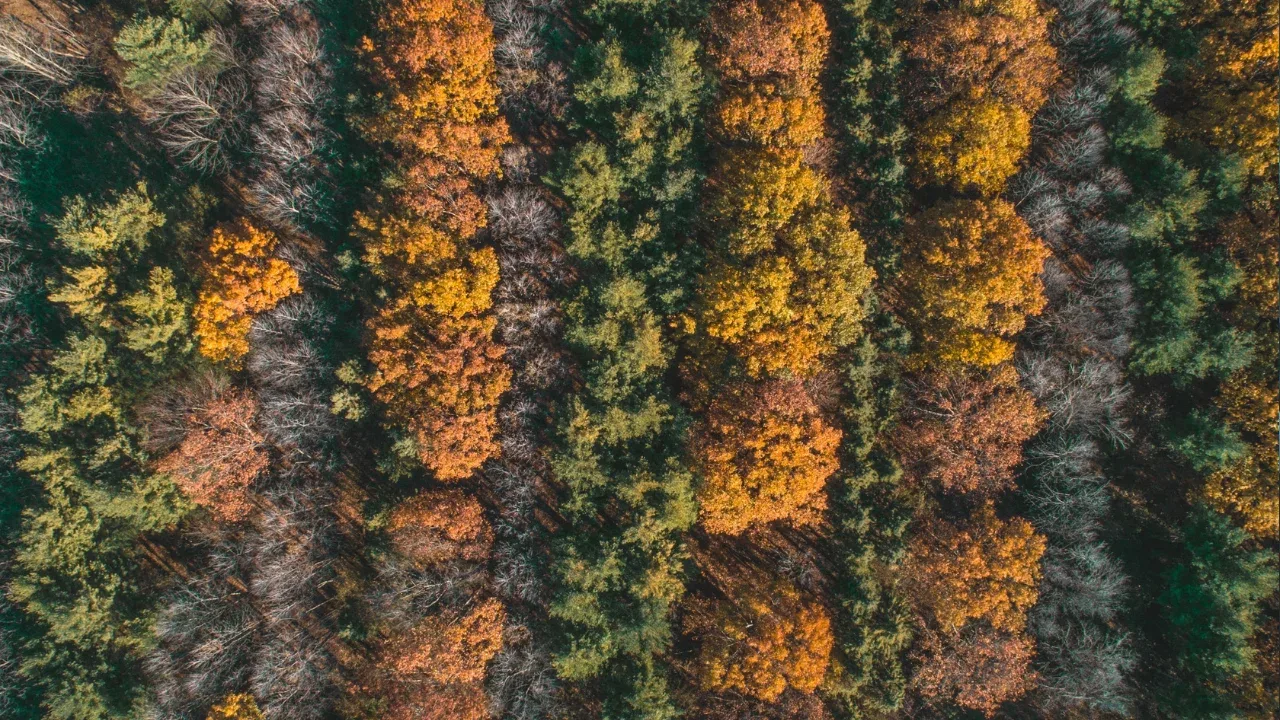
Understanding Execution of Java Code in Comments with Unicode Characters
Have you ever come across a Java code snippet that executes code within comments using certain Unicode characters? If you haven't, take a look at the example below:
public static void main(String... args) {
// The comment below is not a typo.
// \u000d System.out.println("Hello World!");
}
Surprisingly, executing the above code will produce the output "Hello World!" as if the commented line was not actually a comment. But why does this happen? And more importantly, why is it allowed in comments in the first place?
The Magic of Unicode Characters
To understand why Java allows this behavior, we need to delve into the mechanics of Unicode characters and how the Java compiler interprets them. In Java, Unicode characters can be represented using the escape sequence \u
followed by the Unicode hexadecimal value.
In our example, the Unicode escape sequence \u000d
represents the Carriage Return (CR) character. Typically, when the compiler encounters this character within a string literal, it interprets it as a line terminator, causing the printed text to appear on a new line.
However, in our case, the CR character is cleverly placed within a comment. The Java compiler, during its lexical analysis phase, recognizes comments and ignores everything within them. But when it encounters the CR character, it treats it as a line terminator and effectively terminates the comment. As a result, the subsequent code following the comment gets executed.
The Quirks and Benefits of Allowing Such Code Execution
Now that we understand how this code execution in comments works, let's address the elephant in the room - why is it allowed in the Java specification?
One possible reason for allowing this behavior is maintaining backwards compatibility. The Java language has undergone numerous updates and versions over the years, and certain code constructs that might otherwise be flagged as errors or deprecated are allowed to exist for the sake of preserving older codebases.
Furthermore, there might be cases where developers find these unconventional techniques useful. For instance, this feature can be leveraged in code obfuscation or as an unconventional form of code hiding. Although it can be misused by malicious programmers, it ultimately empowers developers with creative approaches to problem-solving.
Ensuring Code Safety
While the ability to execute code within comments can be intriguing, it is crucial to strike a balance between empowerment and security. To ensure code safety, consider the following best practices:
Code Review: Encourage thorough code reviews to catch any potentially harmful code execution in comments.
Static Code Analysis: Employ static analysis tools that can detect unusual code patterns, including code execution within comments.
Education: Continuously educate developers on secure coding practices and potential risks associated with unconventional coding techniques.
By implementing these measures, developers can harness the power of Java's flexibility while maintaining a secure development environment.
Your Takeaway
Next time you stumble upon a Java code snippet that executes code within comments using Unicode characters, don't be perplexed. Embrace the peculiarities of the Java language and its willingness to accommodate unconventional coding techniques.
What's your perspective on this feature? Do you find it useful or potentially problematic? Share your thoughts in the comments below and let's spark a vibrant discussion!
🔥👇 What's your take on executing Java code in comments with Unicode characters? Let us know in the comments below! 👇🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
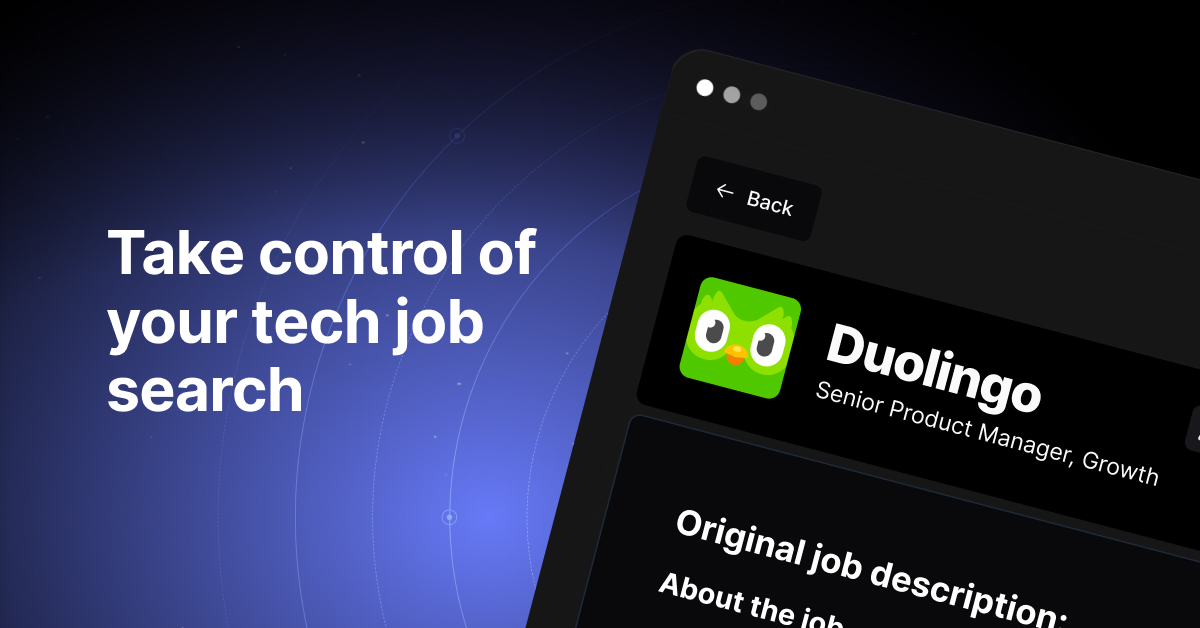